IMZMLprocess¶
%load_ext autoreload
import os,sys
import pandas as pd
import seaborn as sns
import numpy as np
import progressbar
import dill as pickle
from datetime import datetime
import matplotlib as mpl
import matplotlib.pyplot as plt
import logging
sys.path = ["../"] + sys.path
print(sys.path)
%autoreload 2
from pIMZ.regions import SpectraRegion, ProteinWeights
%autoreload 2
from pIMZ.imzml import IMZMLExtract
%autoreload 2
from pIMZ.comparative import CombinedSpectra
import pIMZ
print(pIMZ.__file__)
The autoreload extension is already loaded. To reload it, use:
%reload_ext autoreload
['../', '/mnt/f/dev/git/pyIMS/examples', '/usr/lib/python38.zip', '/usr/lib/python3.8', '/usr/lib/python3.8/lib-dynload', '', '/home/mjoppich/.local/lib/python3.8/site-packages', '/home/mjoppich/.local/lib/python3.8/site-packages/pIMZ-1.0-py3.8-linux-x86_64.egg', '/home/mjoppich/.local/lib/python3.8/site-packages/progressbar-2.5-py3.8.egg', '/usr/lib/python3/dist-packages', '/usr/local/lib/python3.8/dist-packages', '/usr/local/lib/python3.8/dist-packages/mpld3-0.3.1.dev1-py3.8.egg', '/usr/local/lib/python3.8/dist-packages/IPython/extensions', '/home/mjoppich/.ipython', '../']
../pIMZ/__init__.py
Load an imzML file
imze = IMZMLExtract("/mnt/d/dev/data/msi/slideD/181114_AT1_Slide_D_Proteins.imzML")
Opening regions file for /mnt/d/dev/data/msi/slideD/181114_AT1_Slide_D_Proteins.imzML
0 356 400 215 273
1 436 478 632 687
2 1572 1612 608 666
3 1149 1197 142 205
4 618 666 211 266
5 633 684 630 688
6 1357 1400 628 686
Explore which regions are there?
imze.list_regions()
0 ((356, 400, 215, 273), 2655)
1 ((436, 478, 632, 687), 2408)
2 ((1572, 1612, 608, 666), 2419)
3 ((1149, 1197, 142, 205), 3136)
4 ((618, 666, 211, 266), 2744)
5 ((633, 684, 630, 688), 3068)
6 ((1357, 1400, 628, 686), 2596)
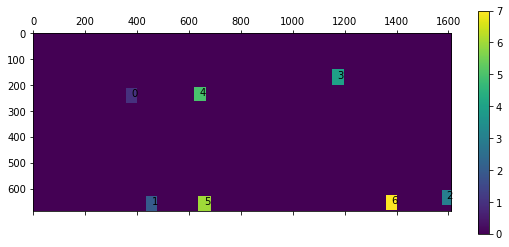
{0: ((356, 400, 215, 273), 2655),
1: ((436, 478, 632, 687), 2408),
2: ((1572, 1612, 608, 666), 2419),
3: ((1149, 1197, 142, 205), 3136),
4: ((618, 666, 211, 266), 2744),
5: ((633, 684, 630, 688), 3068),
6: ((1357, 1400, 628, 686), 2596)}
Start with prozessing the first region , region 0
spectra0_orig = imze.get_region_array(0, makeNullLine=True)
spectra0_intra = imze.normalize_region_array(spectra0_orig, normalize="intra_median")
spectra0 = imze.normalize_region_array(spectra0_intra, normalize="inter_median")
spectra1_orig = imze.get_region_array(1, makeNullLine=True)
spectra1_intra = imze.normalize_region_array(spectra1_orig, normalize="intra_median")
spectra1 = imze.normalize_region_array(spectra1_intra, normalize="inter_median")
imze.plot_fcs(spectra0_orig, [(5,30),(10,30),(20,30),(25,30),(35,30),(40,30)])
imze.plot_fcs(spectra0_intra, [(5,30),(10,30),(20,30),(25,30),(35,30),(40,30)])
imze.plot_fcs(spectra0, [(5,30),(10,30),(20,30),(25,30),(35,30),(40,30)])
imze.plot_fcs(spectra1_orig, [(5,30),(10,30),(20,30),(25,30),(35,30),(40,30)])
imze.plot_fcs(spectra1_intra, [(5,30),(10,30),(20,30),(25,30),(35,30),(40,30)])
imze.plot_fcs(spectra1, [(5,30),(10,30),(20,30),(25,30),(35,30),(40,30)])
We can now ensure that the normalized spectra are indeed comparable.
Where are the highest peaks? This may give a hint on whether or not a normalization by max intensity would also have worked. => here: most probably yes!
imze.list_highest_peaks(spectra0, counter=True)
Let’s plot the norm of each spectrum
imze.plot_tnc(spectra0)
It can be seen that the norm of the spectra differs. However, given that intensities should be comparable, and that there was sample everywhere => just continue
spec = SpectraRegion(spectra0, imze.mzValues)
spec.calculate_similarity(mode="spectra_log")
There are various (unsupervised) clustering techniques. Like UMAP+HDBSCAN
spec.segment(method="UMAP_DBSCAN", number_of_regions=15, min_cluster_size=9, num_samples=1000)
spec.redo_hdbscan_on_dimred(number_of_regions=15, min_cluster_size=9, num_samples=500)
len(spec.dimred_labels)
mpl.rcParams['figure.figsize'] = (10,6)
spec.vis_umap(legend=True)
mpl.rcParams['figure.figsize'] = (6,4)
spec.plot_segments()
spec.filter_clusters(method='remove_singleton')
spec.filter_clusters(method='merge_background')
spec.filter_clusters(method='remove_islands')
spec.plot_segments()
Which may or may not work well - more robustly, and maybe faster is a classic clustering of the similarity scores:
spec.segment(method="WARD", number_of_regions=15)
spec.plot_segments()
spec.filter_clusters(method='remove_singleton')
spec.filter_clusters(method='merge_background')
spec.filter_clusters(method='remove_islands')
spec.plot_segments()
A manual curation of the segmentation is still possible!
spec.segmented[0:6,] = 0
spec.plot_segments()
Consensus Analysis
spec.consensus_spectra()
spec.consensus_similarity()
spec.plot_consensus_similarity()
spec.plot_consensus_similarity(mode="spectra")
All clusters hare a 95% similarity in median. Cluster 0 (background) is the most heterogeneous one.
#spec.plot_inter_consensus_similarity()
Differential Analysis¶
One of the stand-alone features of pyIMS is the integration with differential expression analysis. Here several key-features are presented.
First, single masses are looked at:
spec.mass_heatmap(14954, min_cut_off=0.0025)
The mass with m/z-value 14954 apparently is most active within the aorta structure (center of the image). Is this specific to a specific cluster
dfobj = spec.mass_dabest(14954)
The DABEST-Plot also clearly reveals that in contrast to the background, cluster 8 intensity values for this mass are quite higher than for all other clusters.
Abviously this mass is most intense in Cluster 8, but also cluster 14. We can now take a look at this mass by setting cluster 14 as reference cluster
spec.mass_dabest(14954, background=14)
Not knowing where this cluster 8 is, we can highlight it specifically:
spec.plot_segments(highlight=8)
Or together with cluster 14, where this mass is also prevalent.
On a sidenote: background is set to 0, other regions == 1, and the target region is set to 2
spec.plot_segments(highlight=[8,11])
Just for the sake of clarity: we now remove all differential expression results!
spec.clear_de_results()
Protein m/z to name¶
For any combination of sequencing results with these IMS analyses, knowing which protein was measured is of interest.
Using a previously performed LC-MS/MS experiment, which delivers detected proteins together with the masses, allows an easy translation of m/z values to protein name. This work is done in the ProteinWeights object.
pw = ProteinWeights("protein_weights.tsv")
2020-10-01 10:19:23,279 ProteinWeights INFO: Loaded a total of 5371 proteins with 5341 masses
pw.print_collisions(print_proteins=False)
2020-10-01 10:43:14,176 ProteinWeights INFO: Number of total proteins: 5371
2020-10-01 10:43:14,176 ProteinWeights INFO: Number of total proteins: 5371
2020-10-01 10:43:14,177 ProteinWeights INFO: Number of total masses: 5341
2020-10-01 10:43:14,177 ProteinWeights INFO: Number of total masses: 5341
2020-10-01 10:43:14,178 ProteinWeights INFO: Number of proteins with collision: 1485
2020-10-01 10:43:14,178 ProteinWeights INFO: Number of proteins with collision: 1485
2020-10-01 10:43:14,180 ProteinWeights INFO: Mean Number of Collidings: 1.2552188552188552
2020-10-01 10:43:14,180 ProteinWeights INFO: Mean Number of Collidings: 1.2552188552188552
2020-10-01 10:43:14,181 ProteinWeights INFO: Median Number of Collidings: 1.2552188552188552
2020-10-01 10:43:14,181 ProteinWeights INFO: Median Number of Collidings: 1.2552188552188552
pw.print_collisions(maxdist=1.0, print_proteins=False)
2020-10-01 10:43:02,510 ProteinWeights INFO: Number of total proteins: 5371
2020-10-01 10:43:02,510 ProteinWeights INFO: Number of total proteins: 5371
2020-10-01 10:43:02,511 ProteinWeights INFO: Number of total masses: 5341
2020-10-01 10:43:02,511 ProteinWeights INFO: Number of total masses: 5341
2020-10-01 10:43:02,512 ProteinWeights INFO: Number of proteins with collision: 1119
2020-10-01 10:43:02,512 ProteinWeights INFO: Number of proteins with collision: 1119
2020-10-01 10:43:02,513 ProteinWeights INFO: Mean Number of Collidings: 1.2100089365504916
2020-10-01 10:43:02,513 ProteinWeights INFO: Mean Number of Collidings: 1.2100089365504916
2020-10-01 10:43:02,515 ProteinWeights INFO: Median Number of Collidings: 1.2100089365504916
2020-10-01 10:43:02,515 ProteinWeights INFO: Median Number of Collidings: 1.2100089365504916
pw_theo = ProteinWeights("protein_weights.theo.tsv", max_mass=30010)
2020-10-01 10:54:39,539 ProteinWeights INFO: Loaded a total of 7283 proteins with 10191 masses
2020-10-01 10:54:39,539 ProteinWeights INFO: Loaded a total of 7283 proteins with 10191 masses
2020-10-01 10:54:39,539 ProteinWeights INFO: Loaded a total of 7283 proteins with 10191 masses
pw_theo.print_collisions(print_proteins=False)
2020-10-01 10:54:45,850 ProteinWeights INFO: Number of total proteins: 7283
2020-10-01 10:54:45,850 ProteinWeights INFO: Number of total proteins: 7283
2020-10-01 10:54:45,850 ProteinWeights INFO: Number of total proteins: 7283
2020-10-01 10:54:45,851 ProteinWeights INFO: Number of total masses: 10191
2020-10-01 10:54:45,851 ProteinWeights INFO: Number of total masses: 10191
2020-10-01 10:54:45,851 ProteinWeights INFO: Number of total masses: 10191
2020-10-01 10:54:45,852 ProteinWeights INFO: Number of proteins with collision: 6316
2020-10-01 10:54:45,852 ProteinWeights INFO: Number of proteins with collision: 6316
2020-10-01 10:54:45,852 ProteinWeights INFO: Number of proteins with collision: 6316
2020-10-01 10:54:45,855 ProteinWeights INFO: Mean Number of Collidings: 2.8738125395820138
2020-10-01 10:54:45,855 ProteinWeights INFO: Mean Number of Collidings: 2.8738125395820138
2020-10-01 10:54:45,855 ProteinWeights INFO: Mean Number of Collidings: 2.8738125395820138
2020-10-01 10:54:45,858 ProteinWeights INFO: Median Number of Collidings: 2.0
2020-10-01 10:54:45,858 ProteinWeights INFO: Median Number of Collidings: 2.0
2020-10-01 10:54:45,858 ProteinWeights INFO: Median Number of Collidings: 2.0
2020-10-01 10:54:45,860 ProteinWeights INFO: Proteins with collision: [('Mbp', 30), ('Crem', 25), ('Homer1', 18), ('Clta', 18), ('Eif4e2', 18), ('U2af1l4', 16), ('Tspan32', 16), ('Tpd52', 16), ('Asph', 16), ('Hmga1', 15)]
2020-10-01 10:54:45,860 ProteinWeights INFO: Proteins with collision: [('Mbp', 30), ('Crem', 25), ('Homer1', 18), ('Clta', 18), ('Eif4e2', 18), ('U2af1l4', 16), ('Tspan32', 16), ('Tpd52', 16), ('Asph', 16), ('Hmga1', 15)]
2020-10-01 10:54:45,860 ProteinWeights INFO: Proteins with collision: [('Mbp', 30), ('Crem', 25), ('Homer1', 18), ('Clta', 18), ('Eif4e2', 18), ('U2af1l4', 16), ('Tspan32', 16), ('Tpd52', 16), ('Asph', 16), ('Hmga1', 15)]
pw_theo.print_collisions(maxdist=1.0, print_proteins=False)
2020-10-01 10:54:54,221 ProteinWeights INFO: Number of total proteins: 7283
2020-10-01 10:54:54,221 ProteinWeights INFO: Number of total proteins: 7283
2020-10-01 10:54:54,221 ProteinWeights INFO: Number of total proteins: 7283
2020-10-01 10:54:54,223 ProteinWeights INFO: Number of total masses: 10191
2020-10-01 10:54:54,223 ProteinWeights INFO: Number of total masses: 10191
2020-10-01 10:54:54,223 ProteinWeights INFO: Number of total masses: 10191
2020-10-01 10:54:54,224 ProteinWeights INFO: Number of proteins with collision: 4881
2020-10-01 10:54:54,224 ProteinWeights INFO: Number of proteins with collision: 4881
2020-10-01 10:54:54,224 ProteinWeights INFO: Number of proteins with collision: 4881
2020-10-01 10:54:54,226 ProteinWeights INFO: Mean Number of Collidings: 1.9096496619545176
2020-10-01 10:54:54,226 ProteinWeights INFO: Mean Number of Collidings: 1.9096496619545176
2020-10-01 10:54:54,226 ProteinWeights INFO: Mean Number of Collidings: 1.9096496619545176
2020-10-01 10:54:54,228 ProteinWeights INFO: Median Number of Collidings: 2.0
2020-10-01 10:54:54,228 ProteinWeights INFO: Median Number of Collidings: 2.0
2020-10-01 10:54:54,228 ProteinWeights INFO: Median Number of Collidings: 2.0
2020-10-01 10:54:54,230 ProteinWeights INFO: Proteins with collision: [('Mbp', 19), ('Spcs2', 11), ('Tpd52', 11), ('Erh', 10), ('Crem', 10), ('Vps25', 10), ('Ifi27', 10), ('Nudt7', 10), ('Clta', 10), ('Eif4e2', 10)]
2020-10-01 10:54:54,230 ProteinWeights INFO: Proteins with collision: [('Mbp', 19), ('Spcs2', 11), ('Tpd52', 11), ('Erh', 10), ('Crem', 10), ('Vps25', 10), ('Ifi27', 10), ('Nudt7', 10), ('Clta', 10), ('Eif4e2', 10)]
2020-10-01 10:54:54,230 ProteinWeights INFO: Proteins with collision: [('Mbp', 19), ('Spcs2', 11), ('Tpd52', 11), ('Erh', 10), ('Crem', 10), ('Vps25', 10), ('Ifi27', 10), ('Nudt7', 10), ('Clta', 10), ('Eif4e2', 10)]
With the m/z->protein object we can now find all marker masses for the 15 detected regions
For example we can also try to find out, which protein corresponds to mass 14954 ! (it’s Ifitm3 …)
pw.get_protein_from_mass(14954)
[('Ifitm3', 14954.0)]
pw_theo.get_protein_from_mass(14954)
[('Tnfrsf12a', 14952.97469999999), ('Ifitm3', 14954.185999999994)]
for y in pw_theo.protein2mass:
if not y.startswith(("Ccl", "Ccr", "Cxc", "Il")):
continue
for x in pw_theo.get_masses_for_protein(y):
massprots = pw_theo.get_protein_from_mass(x)
if len(massprots) <= 2:
print(x, massprots)
slided_0.mass_heatmap(x)
2020-10-01 11:06:33,879 SpectraRegion INFO: Processing Mass 19494.35879999999 with best existing mass 19493.942202125156
19494.35879999999 [('Ccl27a', 19494.35879999999)]
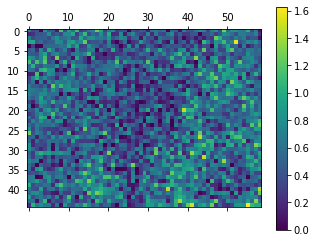
2020-10-01 11:06:33,996 SpectraRegion INFO: Processing Mass 10099.632999999985 with best existing mass 10099.587949181696
10099.632999999985 [('Ccl27a', 10099.632999999985), ('Chchd7', 10101.449999999995)]
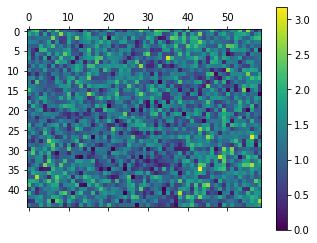
2020-10-01 11:06:34,132 SpectraRegion INFO: Processing Mass 14651.815799999984 with best existing mass 14651.133720567635
14651.815799999984 [('Ccl27a', 14651.815799999984), ('Vbp1', 14652.91139999998)]
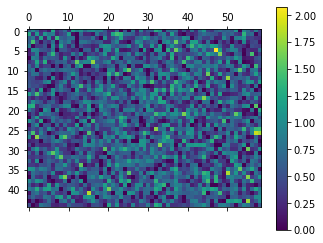
2020-10-01 11:06:34,479 SpectraRegion INFO: Processing Mass 12579.54569999998 with best existing mass 12579.09381867344
12579.54569999998 [('Ccl19', 12579.54569999998)]
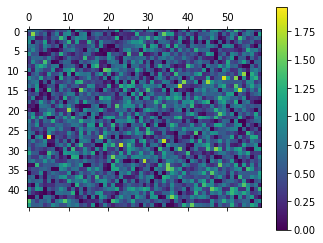
2020-10-01 11:06:34,601 SpectraRegion INFO: Processing Mass 14557.82079999998 with best existing mass 14557.567461341532
14557.82079999998 [('Ccl21a', 14557.82079999998)]
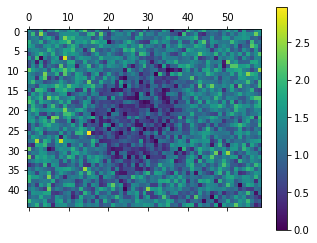
2020-10-01 11:06:34,721 SpectraRegion INFO: Processing Mass 15271.877899999985 with best existing mass 15271.387471243903
15271.877899999985 [('Il11', 15271.877899999985)]
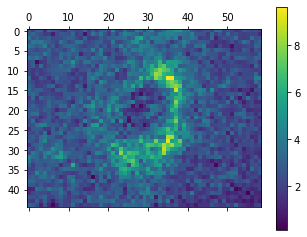
2020-10-01 11:06:34,839 SpectraRegion INFO: Processing Mass 13065.960899999984 with best existing mass 13066.543846577173
13065.960899999984 [('Il7', 13065.960899999984)]
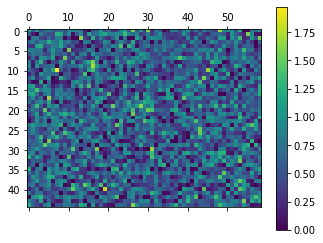
2020-10-01 11:06:34,959 SpectraRegion INFO: Processing Mass 4892.7843 with best existing mass 4893.078363213031
4892.7843 [('Il2ra', 4892.7843)]
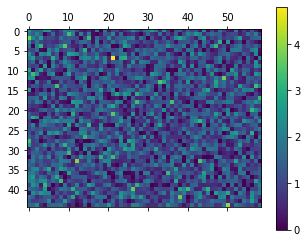
2020-10-01 11:06:35,070 SpectraRegion INFO: Processing Mass 12904.570899999993 with best existing mass 12905.066592751478
12904.570899999993 [('Il15ra', 12904.570899999993), ('Cav2', 12905.530399999985)]
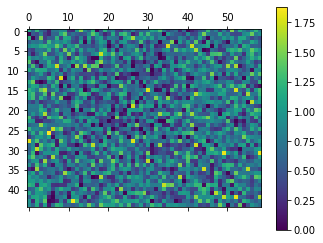
2020-10-01 11:06:35,189 SpectraRegion INFO: Processing Mass 18122.08549999999 with best existing mass 18122.14011121341
18122.08549999999 [('Il15ra', 18122.08549999999), ('Msh3', 18122.3698)]
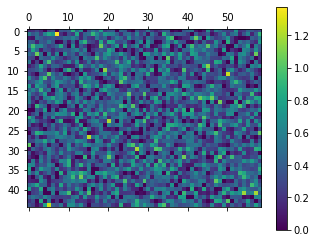
2020-10-01 11:06:35,304 SpectraRegion INFO: Processing Mass 21365.61989999999 with best existing mass 21365.26738664723
21365.61989999999 [('Il15ra', 21365.61989999999), ('Epo', 21364.868400000014)]
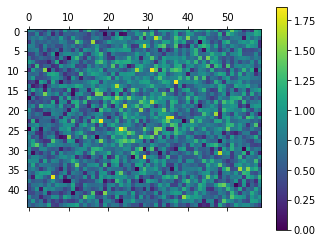
2020-10-01 11:06:35,416 SpectraRegion INFO: Processing Mass 11161.56199999999 with best existing mass 11162.017731361968
11161.56199999999 [('Il15ra', 11161.56199999999), ('Rbis', 11162.921699999992)]
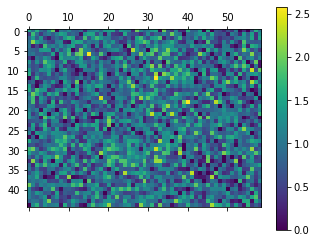
2020-10-01 11:06:35,523 SpectraRegion INFO: Processing Mass 21687.485200000006 with best existing mass 21688.22189429862
21687.485200000006 [('Il3ra', 21687.485200000006)]
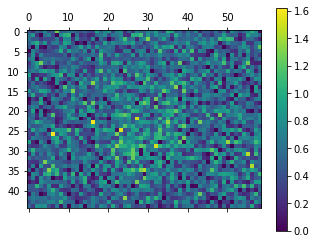
2020-10-01 11:06:35,643 SpectraRegion INFO: Processing Mass 26612.208100000018 with best existing mass 26612.523569375648
26612.208100000018 [('Il22ra2', 26612.208100000018)]
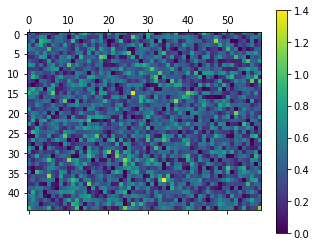
2020-10-01 11:06:35,759 SpectraRegion INFO: Processing Mass 17490.081799999993 with best existing mass 17489.81329483055
17490.081799999993 [('Il17a', 17490.081799999993)]
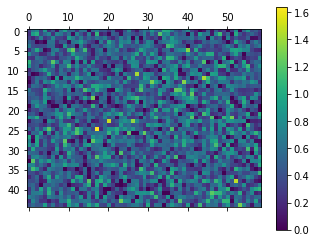
2020-10-01 11:06:35,893 SpectraRegion INFO: Processing Mass 18279.695999999993 with best existing mass 18279.089965399136
18279.695999999993 [('Il1rn', 18279.695999999993)]
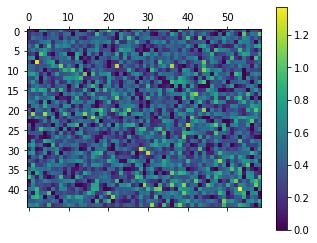
2020-10-01 11:06:36,017 SpectraRegion INFO: Processing Mass 5903.754799999999 with best existing mass 5904.197616140279
5903.754799999999 [('Il1rn', 5903.754799999999)]
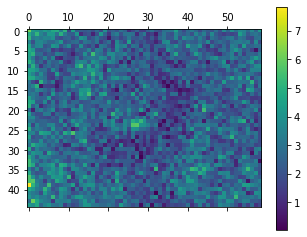
2020-10-01 11:06:36,130 SpectraRegion INFO: Processing Mass 13626.99059999998 with best existing mass 13626.43226872047
13626.99059999998 [('Cxcl17', 13626.99059999998), ('Wfdc10', 13626.576499999985)]
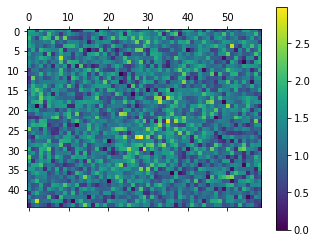
2020-10-01 11:06:36,247 SpectraRegion INFO: Processing Mass 18202.5728 with best existing mass 18202.1241715196
18202.5728 [('Il17rd', 18202.5728)]
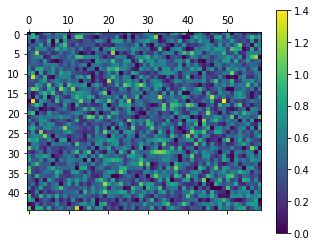
2020-10-01 11:06:36,359 SpectraRegion INFO: Processing Mass 23928.884999999987 with best existing mass 23929.28471608513
23928.884999999987 [('Ilrun', 23928.884999999987), ('Stradb', 23930.18550000001)]
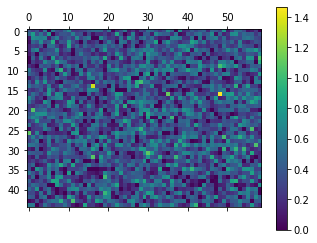
2020-10-01 11:06:36,468 SpectraRegion INFO: Processing Mass 24900.095199999996 with best existing mass 24899.657372252623
24900.095199999996 [('Ilrun', 24900.095199999996)]
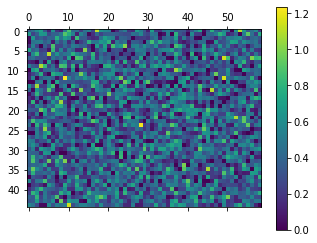
2020-10-01 11:06:36,579 SpectraRegion INFO: Processing Mass 29990.628199999992 with best existing mass 29989.96370079532
29990.628199999992 [('Il33', 29990.628199999992), ('Gnas', 29991.278499999975)]
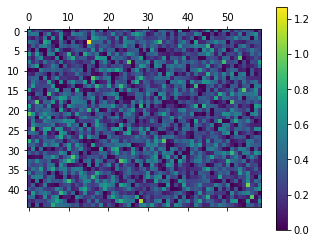
2020-10-01 11:06:36,693 SpectraRegion INFO: Processing Mass 23789.840400000016 with best existing mass 23790.444460459297
23789.840400000016 [('Il6', 23789.840400000016)]
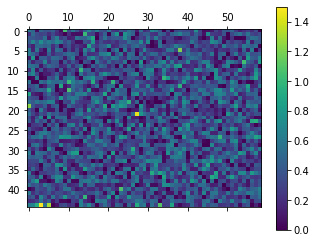
2020-10-01 11:06:36,811 SpectraRegion INFO: Processing Mass 24383.67730000004 with best existing mass 24383.53381329573
24383.67730000004 [('Il6', 24383.67730000004), ('Trappc4', 24384.7599)]
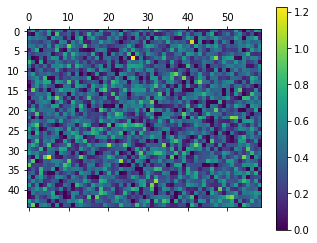
2020-10-01 11:06:36,928 SpectraRegion INFO: Processing Mass 19399.816999999995 with best existing mass 19400.375942899052
19399.816999999995 [('Il2', 19399.816999999995)]
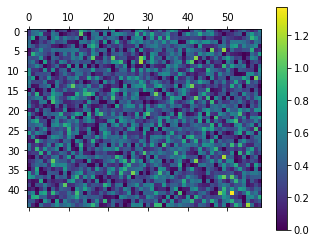
2020-10-01 11:06:37,040 SpectraRegion INFO: Processing Mass 16810.60699999999 with best existing mass 16810.70334883464
16810.60699999999 [('Il21', 16810.60699999999)]
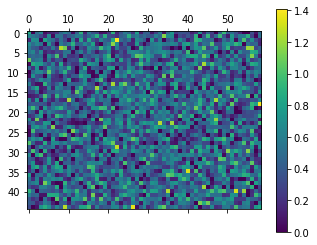
2020-10-01 11:06:37,159 SpectraRegion INFO: Processing Mass 8872.763199999998 with best existing mass 8872.66264674908
8872.763199999998 [('Il18', 8872.763199999998), ('Ss18l2', 8873.036699999993)]
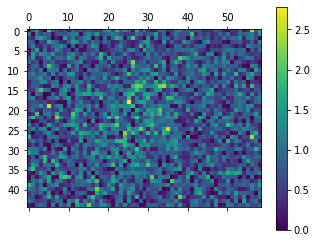
2020-10-01 11:06:37,264 SpectraRegion INFO: Processing Mass 22149.901800000007 with best existing mass 22150.016657575838
22149.901800000007 [('Il18', 22149.901800000007), ('Bhlha15', 22149.542699999965)]
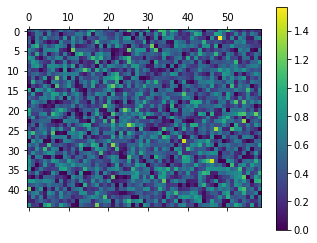
2020-10-01 11:06:37,373 SpectraRegion INFO: Processing Mass 5272.061099999999 with best existing mass 5271.870799757418
5272.061099999999 [('Il4', 5272.061099999999)]
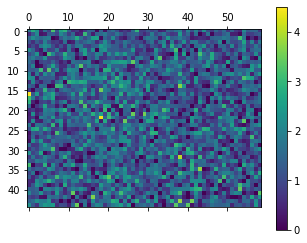
2020-10-01 11:06:37,674 SpectraRegion INFO: Processing Mass 14107.345999999974 with best existing mass 14107.845763770905
14107.345999999974 [('Il13', 14107.345999999974), ('Tnfrsf18', 14106.149199999985)]
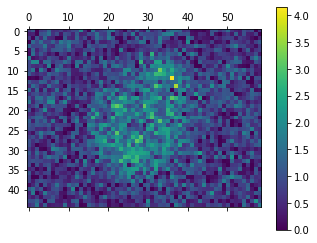
2020-10-01 11:06:37,783 SpectraRegion INFO: Processing Mass 15409.923999999985 with best existing mass 15410.227726869734
15409.923999999985 [('Il5', 15409.923999999985)]
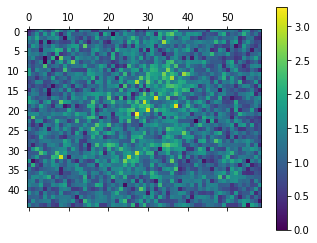
2020-10-01 11:06:37,890 SpectraRegion INFO: Processing Mass 18540.14679999999 with best existing mass 18540.17001130423
18540.14679999999 [('Il3', 18540.14679999999)]
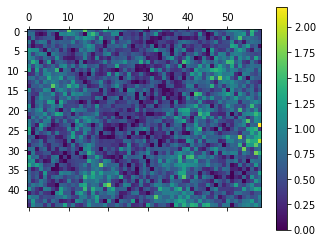
2020-10-01 11:06:38,012 SpectraRegion INFO: Processing Mass 6986.472600000002 with best existing mass 6986.246130093767
6986.472600000002 [('Cxcl14', 6986.472600000002)]
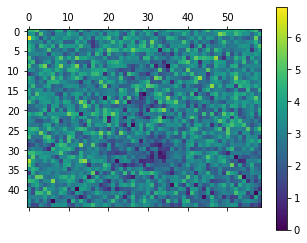
2020-10-01 11:06:38,138 SpectraRegion INFO: Processing Mass 22348.43089999998 with best existing mass 22347.713108521315
22348.43089999998 [('Il17d', 22348.43089999998), ('Fgf7', 22346.767500000016)]
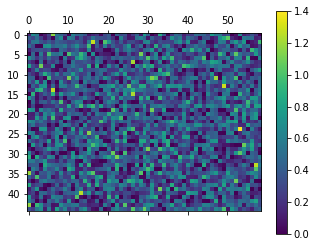
2020-10-01 11:06:38,248 SpectraRegion INFO: Processing Mass 20308.54879999999 with best existing mass 20308.874137320254
20308.54879999999 [('Il17b', 20308.54879999999), ('Il17c', 20308.787899999992)]
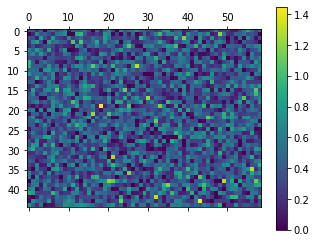
2020-10-01 11:06:38,353 SpectraRegion INFO: Processing Mass 24178.823800000006 with best existing mass 24178.29169628363
24178.823800000006 [('Il12a', 24178.823800000006), ('Ankrd55', 24177.100800000022)]
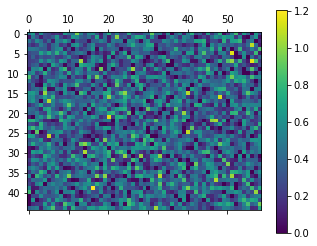
26204.00270000001 [('Prss2', 26203.25329999999), ('Il12a', 26204.00270000001)]
2020-10-01 11:06:38,454 SpectraRegion INFO: Processing Mass 26204.00270000001 with best existing mass 26203.548468564775
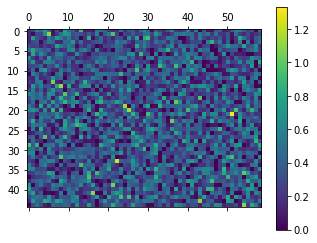
26895.941099999996
2020-10-01 11:06:38,561 SpectraRegion INFO: Processing Mass 26895.941099999996 with best existing mass 26896.240613480608
[('Cxcl16', 26895.941099999996)]
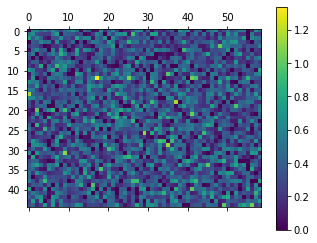
2020-10-01 11:06:38,675 SpectraRegion INFO: Processing Mass 16325.812299999983 with best existing mass 16326.271587357554
16325.812299999983 [('Fam183b', 16327.27959999999), ('Ccl2', 16325.812299999983)]
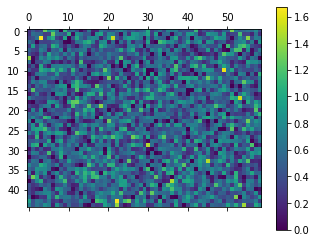
2020-10-01 11:06:38,787 SpectraRegion INFO: Processing Mass 10892.872099999986 with best existing mass 10893.392019390252
10892.872099999986 [('Fam216b', 10891.48299999999), ('Ccl11', 10892.872099999986)]
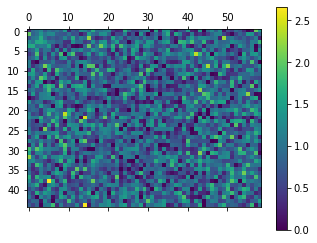
2020-10-01 11:06:38,898 SpectraRegion INFO: Processing Mass 11658.704599999988 with best existing mass 11658.522558545646
11658.704599999988 [('Pate3', 11659.626499999986), ('Ccl12', 11658.704599999988)]
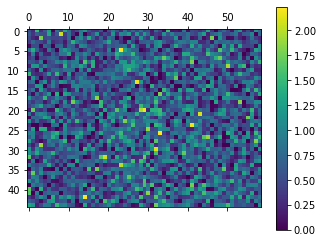
2020-10-01 11:06:39,010 SpectraRegion INFO: Processing Mass 11017.193999999998 with best existing mass 11017.140942882841
11017.193999999998 [('Eif4ebp3', 11018.495599999993), ('Ccl8', 11017.193999999998)]
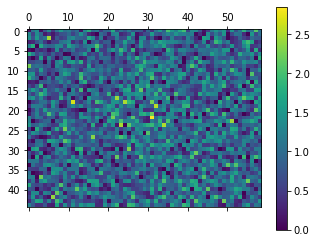
2020-10-01 11:06:39,129 SpectraRegion INFO: Processing Mass 10754.69929999999 with best existing mass 10754.55176376442
10754.69929999999 [('Ccl20', 10754.69929999999)]
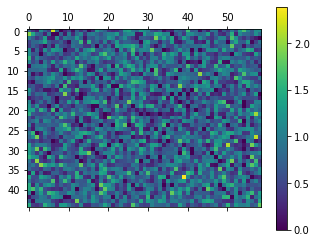
2020-10-01 11:06:39,246 SpectraRegion INFO: Processing Mass 13870.752799999982 with best existing mass 13870.911849278998
13870.752799999982 [('Ccl9', 13870.752799999982)]
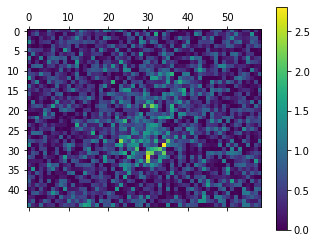
2020-10-01 11:06:39,354 SpectraRegion INFO: Processing Mass 10167.716399999996 with best existing mass 10167.498943781287
10167.716399999996 [('Rad54b', 10169.499699999991), ('Ccl4', 10167.716399999996)]
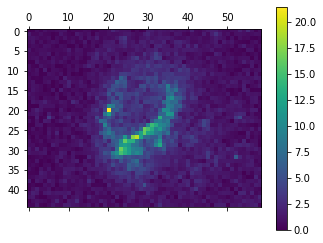
2020-10-01 11:06:39,473 SpectraRegion INFO: Processing Mass 10254.250199999993 with best existing mass 10253.51953694077
10254.250199999993 [('Cxcl1', 10254.250199999993)]
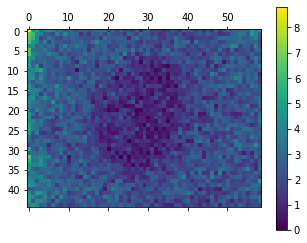
2020-10-01 11:06:39,589 SpectraRegion INFO: Processing Mass 10620.574399999989 with best existing mass 10620.238907778563
10620.574399999989 [('Gm525', 10620.183199999994), ('Cxcl2', 10620.574399999989)]
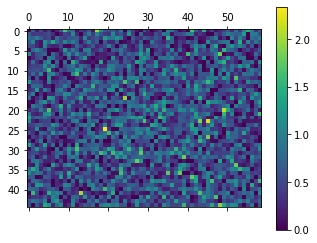
2020-10-01 11:06:39,698 SpectraRegion INFO: Processing Mass 10788.802299999987 with best existing mass 10789.261827670878
10788.802299999987 [('Zfp866', 10789.753199999994), ('Cxcl10', 10788.802299999987)]
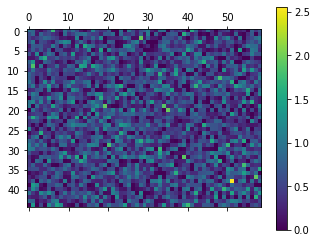
2020-10-01 11:06:39,816 SpectraRegion INFO: Processing Mass 11152.35079999999 with best existing mass 11152.962932082024
11152.35079999999 [('Cxcl11', 11152.35079999999)]
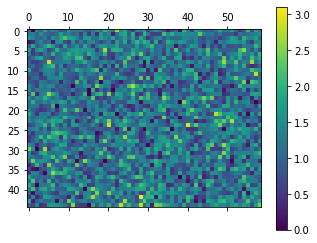
2020-10-01 11:06:39,928 SpectraRegion INFO: Processing Mass 11538.699599999984 with best existing mass 11539.301034693031
11538.699599999984 [('Crem', 11537.28529999999), ('Ccl17', 11538.699599999984)]
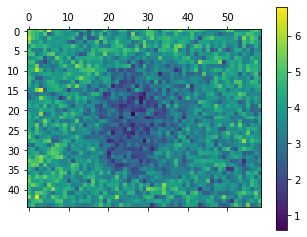
2020-10-01 11:06:40,049 SpectraRegion INFO: Processing Mass 21899.871200000005 with best existing mass 21899.500544164013
21899.871200000005 [('Psmb1', 21900.825699999972), ('Il18bp', 21899.871200000005)]
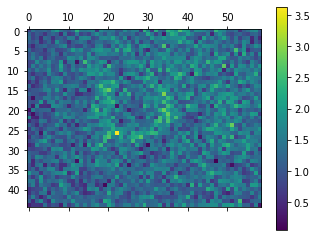
2020-10-01 11:06:40,167 SpectraRegion INFO: Processing Mass 26763.888900000027 with best existing mass 26763.43689070807
26763.888900000027 [('Il34', 26763.888900000027)]
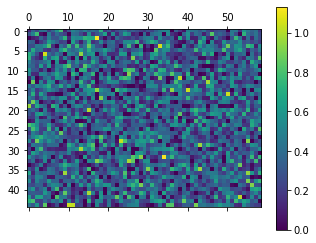
2020-10-01 11:06:40,279 SpectraRegion INFO: Processing Mass 18538.088100000008 with best existing mass 18538.66087809091
18538.088100000008 [('Il6st', 18538.088100000008), ('Mfap5', 18537.995099999996)]
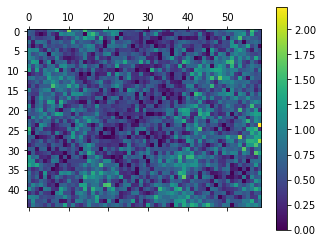
2020-10-01 11:06:40,385 SpectraRegion INFO: Processing Mass 7292.779600000001 with best existing mass 7292.600172398589
7292.779600000001 [('Il6st', 7292.779600000001)]
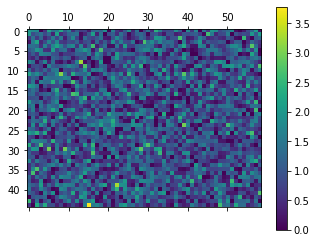
2020-10-01 11:06:40,496 SpectraRegion INFO: Processing Mass 10560.59809999999 with best existing mass 10559.873579245592
10560.59809999999 [('Mrps21', 10561.238699999993), ('Cxcl12', 10560.59809999999)]
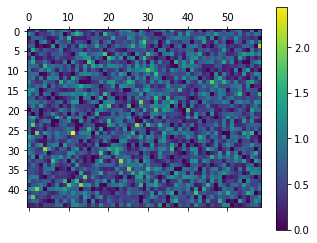
2020-10-01 11:06:40,788 SpectraRegion INFO: Processing Mass 13634.183899999978 with best existing mass 13633.977934787092
13634.183899999978 [('Ube2a', 13635.07789999999), ('Cxcl12', 13634.183899999978)]
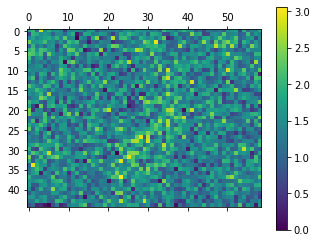
2020-10-01 11:06:40,905 SpectraRegion INFO: Processing Mass 10031.886499999991 with best existing mass 10031.676954582104
10031.886499999991 [('Tnfrsf12a', 10032.82969999999), ('Cxcl12', 10031.886499999991)]
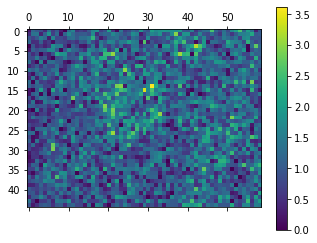
2020-10-01 11:06:41,020 SpectraRegion INFO: Processing Mass 14569.692699999976 with best existing mass 14569.640527048126
14569.692699999976 [('Ccl28', 14569.692699999976), ('Trmt2b', 14568.390499999981)]
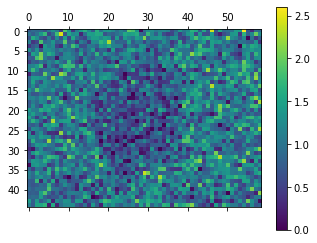
2020-10-01 11:06:41,132 SpectraRegion INFO: Processing Mass 20308.787899999992 with best existing mass 20308.874137320254
20308.787899999992 [('Il17b', 20308.54879999999), ('Il17c', 20308.787899999992)]
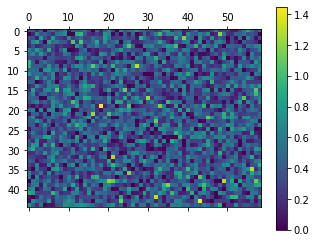
2020-10-01 11:06:41,242 SpectraRegion INFO: Processing Mass 11199.70799999999 with best existing mass 11199.746061695076
11199.70799999999 [('Ss18l2', 11201.606299999994), ('Il31', 11199.70799999999)]
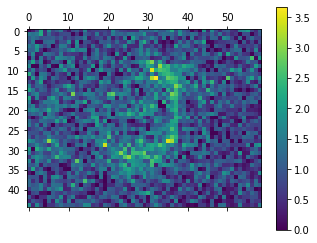
2020-10-01 11:06:41,349 SpectraRegion INFO: Processing Mass 18119.635499999997 with best existing mass 18119.121844786765
18119.635499999997 [('Tpo', 18119.2567), ('Il31', 18119.635499999997)]
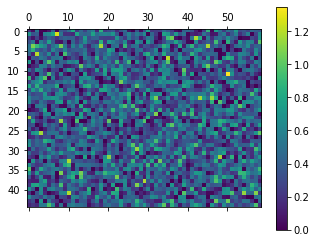
2020-10-01 11:06:41,457 SpectraRegion INFO: Processing Mass 20872.213499999998 with best existing mass 20871.780825890197
20872.213499999998 [('Il24', 20872.213499999998)]
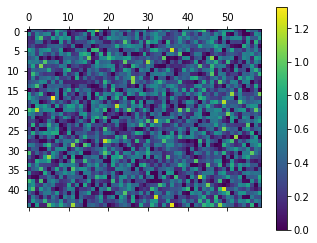
2020-10-01 11:06:41,570 SpectraRegion INFO: Processing Mass 17542.508099999995 with best existing mass 17542.6329572969
17542.508099999995 [('Arl14epl', 17540.928299999996), ('Il20', 17542.508099999995)]
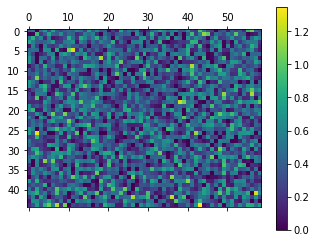
2020-10-01 11:06:41,682 SpectraRegion INFO: Processing Mass 11672.063399999986 with best existing mass 11672.104757465566
11672.063399999986 [('Il19', 11672.063399999986)]
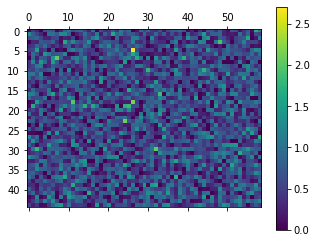
2020-10-01 11:06:41,790 SpectraRegion INFO: Processing Mass 20287.34260000001 with best existing mass 20287.746272333712
20287.34260000001 [('Il19', 20287.34260000001)]
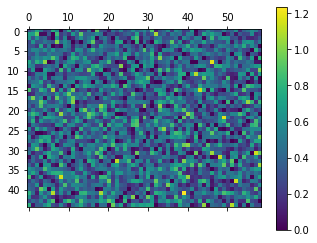
2020-10-01 11:06:41,897 SpectraRegion INFO: Processing Mass 22536.800200000012 with best existing mass 22536.354760186845
22536.800200000012 [('Sult2a2', 22538.584699999978), ('Ildr2', 22536.800200000012)]
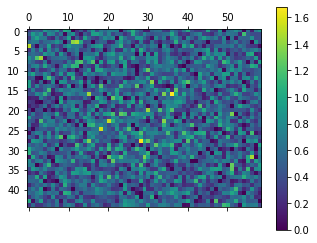
slided_0.mass_heatmap(Ccl9)
2020-10-01 10:55:57,023 SpectraRegion INFO: Processing Mass 13870 with best existing mass 13869.402716065675
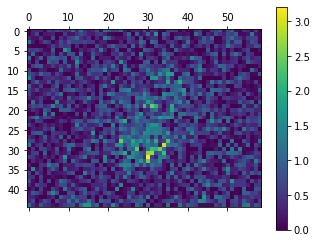
array([[0. , 0.33562942, 0.29980934, ..., 0.08112048, 0.57262519,
0. ],
[0.02545898, 0.28970706, 0.69557764, ..., 1.32654748, 0.20488023,
0.10190447],
[0.3632469 , 0.17660935, 0.16031279, ..., 0.66523704, 0.66826813,
0.07720524],
...,
[0.31846044, 0.3063842 , 0.13660394, ..., 0.6416831 , 0.39386746,
0.42146079],
[0.35692512, 0.74327409, 0. , ..., 0.33920547, 0.06606368,
0.6278585 ],
[1.12895917, 0.37550086, 0.18109881, ..., 0.37619895, 0.84541711,
0.65886899]])
Marker masses/proteins/genes are genes which are differentially regulated when compared to the specific cluster and all other clusters (including or excluding the background cluster).
Making the distinction regarding the background cluster might be required if the target tissue is embedded in another tissue. Excluding the background might then deliver more sensitive results.
mgenes = spec.find_all_markers(pw, includeBackground=False,
replaceExisting=False,
outdirectory="./deresults/",
use_methods=["ttest", "rank"])
mgenes["ttest"]
mgenes_bg = spec.find_all_markers(pw, includeBackground=True, replaceExisting=False, use_methods = ["ttest", "rank"])
After both find_all_markers runs we can list all DE results, which are then printed. Each row shows one contained DE results beginning with the test performed and then a tuple of two region/cluster IDs. In the first row here:
a t-test was performed on cluster 9 versus clusters 8,10,11,12,13,14,15 .
spec.list_de_results()
It’s also easy to save the result to disk:
markerGenes = mgenes["ttest"]
markerGenes.to_csv("deresults/marker_genes.tsv", sep="\t", index=False)
markerGenesBG = mgenes_bg["ttest"]
markerGenesBG.to_csv("deresults/marker_genes_bg.tsv", sep="\t", index=False)
print("Number of unique, differentially detected proteins (from masses): {}".format(len(set(mgenes["ttest"]["gene"]))))
print("Number of unique, differentially detected proteins (from masses): {}".format(len(set(mgenes_bg["ttest"]["gene"]))))
In order to save the results, the SpectraRegion object can be pickled:
with open("slideD_region_0.spec.pickle", "wb") as fout:
pickle.dump(spec, fout)
Cell-type detection¶
Cell-type detection requires analyseMarkers.py from https://github.com/mjoppich/scrnaseq_celltype_prediction .
One (obvious) question is: what can I do with those marker proteins? Well, a lot!
First, these are the proteins which you are interested in: these define your clusters, because they are present.
Second, these proteins give a clue about which cell types might be present, if cell-type specific genes were found.
Using the scrnaseq-celltype-prediction tool, this can be analysed! Let’s download it first (requires wget, python3 and several other libraries - maybe). Did you know? This tool achieves better predictions than SingleR :)
Because we know what kind of sample we got (aorta embedded in liver), we can specify organs to specifically check for cell types. Organs to be considered are “Connective tissue” “Vasculature” “Heart” “Skeletal Muscle” “Smooth muscle” “Immune system” “Blood” “Epithelium” “Liver”. This allows a context-specific evaluation!
! ls analyseMarkers.py
! wget https://raw.githubusercontent.com/mjoppich/scrnaseq_celltype_prediction/master/analyseMarkers.py
! python3 analyseMarkers.py --organs "Connective tissue" "Vasculature" "Heart" "Skeletal Muscle" "Smooth muscle" "Immune system" "Blood" "Epithelium" "Liver" --pvaladj qvalue --markers deresults/marker_genes.tsv -n 2
This is quite interesting. For cluster 8 this tool predicts mainly monocytes or gamma delta t cells - cells which do not migrate into other tissues.
This makes sense, because one would expect these cell-types in the inner of the vessel.
H2AFX is known to be upregulated in lymphoblasts (check wikipedia ;) ). Ifitm3 is highly expressed in both, Liver and Monocytes: https://www.proteinatlas.org/ENSG00000142089-IFITM3/tissue (Consensus dataset)
spec.plot_segments(highlight=(8))
! python3 analyseMarkers.py --organs "Connective tissue" "Vasculature" "Heart" "Skeletal Muscle" "Smooth muscle" "Immune system" "Blood" "Epithelium" "Liver" --pvaladj qvalue --markers deresults/marker_genes_bg.tsv -n 2
In this analysis, the background was kept in. More differential genes are found, but also more liver-related cell types are found.
This suggests that the analysis which includes the liver background has influenced the found differential proteins too much.
Nonetheless, the results remain consistent. For cluster 8, the missing monocytes are replaced by hepatocytes, which are monocyte-like cells residing in the liver.
Cluster 9 showing high amounds of cardiomyocytes is also not unlikely, given its location at the outer area of the aorta.
spec.plot_segments(highlight=(9))
Finally the SpectraRegion can also be exported to Aorta3D.
#spec.to_aorta3d("./slided_test/", "slided", 0, protWeights=pw, nodf=False, pathPrefix="../data/test_msi/")
!ls slided_test
Preparations for CombinedSpectra - A comparative analysis¶
spectra1 = imze.get_region_array(1, makeNullLine=True)
imze.normalize_region_array(spectra1, normalize="vector")
imze.list_highest_peaks(spectra1, counter=True)
print("Got spectra", spectra1.shape)
spec1 = SpectraRegion(spectra1, imze.mzValues)
spec1.calculate_similarity(mode="spectra_log_dist")
spec1.segment(method="WARD", number_of_regions=15)
spec1.plot_segments()
So far the results were only for one IMS sample.
Now the idea is to integrate multiple samples, in order to compare the different samples which were measured on the same slide.
Hence the remaining samples are processed, all in the same fashion. With the inter and intro normalization, the sample are made comparable.
pw = ProteinWeights("protein_weights.tsv")
def process_imzeregion(imze, regionID, basename):
print("Processing regionID", regionID, "for basename", basename)
spectra_orig = imze.get_region_array(regionID, makeNullLine=True)
spectra_intra = imze.normalize_region_array(spectra_orig, normalize="intra_median")
spectra = imze.normalize_region_array(spectra_intra, normalize="inter_median")
rspec = SpectraRegion(spectra, imze.mzValues, name=basename + "_" + str(regionID))
rspec.calculate_similarity(mode="spectra_log_dist")
rspec.segment(method="WARD", number_of_regions=15)
rspec.plot_segments()
rspec.filter_clusters(method='remove_singleton')
rspec.filter_clusters(method='merge_background')
rspec.filter_clusters(method='remove_islands')
rspec.filter_clusters(method='remove_islands', minIslandSize=15)
rspec.plot_segments()
#rspec.consensus_spectra()
#rspec.consensus_similarity()
#rspec.plot_consensus_similarity()
mgenes = None
mgenes_bg = None
mgenes = rspec.find_all_markers(pw, includeBackground=False, replaceExisting=False, use_methods = ["ttest", "rank"])
mgenes_bg = rspec.find_all_markers(pw, includeBackground=True, replaceExisting=False, use_methods = ["ttest", "rank"])
return rspec, mgenes, mgenes_bg
First set the logging level to warn, to remove too many messages …
#loggers = [logging.getLogger(name) for name in logging.root.manager.loggerDict]
#for logger in loggers:
# logger.setLevel(logging.WARN)
slided_0, slided0_mgenes, slided0_mgenes_bg = process_imzeregion(imze=imze, regionID= 0, basename="slideD")
slided_1, slided1_mgenes, slided1_mgenes_bg = process_imzeregion(imze=imze, regionID= 1, basename="slideD")
Fetching region range
Fetching region shape
Found region 0 with shape (45, 59, 17900)
Fetching region spectra
5% (135 of 2655) |# | Elapsed Time: 0:00:00 ETA: 0:00:03
Processing regionID 0 for basename slideD
100% (2655 of 2655) |####################| Elapsed Time: 0:00:03 Time: 0:00:03
100% (2655 of 2655) |####################| Elapsed Time: 0:00:00 Time: 0:00:00
Started Log Value: 0.14000733569264412
100% (45 of 45) |########################| Elapsed Time: 0:00:00 Time: 0:00:00
Got 2655 median-enabled pixels
5-Number stats for medians: (2655, 2655, 0.2905536462232088, 0.5712127712945096, 0.6654297160132764, 0.7571640240536153, 1.119313296797138)
Started Log Value: 0.21725614592432976
Collecting fold changes
100% (45 of 45) |########################| Elapsed Time: 0:00:17 Time: 0:00:17
Got a total of 47524500 fold changes
Median elements [23762250]
Median elements
Global Median 0.64579
2020-09-30 22:41:10,939 SpectraRegion INFO: dimensions inputarray: 17900
2020-09-30 22:41:10,939 SpectraRegion INFO: Creating C++ obj
2020-09-30 22:41:10,940 SpectraRegion INFO: 17900 (45, 59, 17900)
2020-09-30 22:41:10,940 SpectraRegion INFO: dimensions 17900
2020-09-30 22:41:10,941 SpectraRegion INFO: input dimensions (45, 59, 17900)
2020-09-30 22:41:10,942 SpectraRegion INFO: Switching to dot mode
2020-09-30 22:41:11,029 SpectraRegion INFO: Starting calc similarity c++
2020-09-30 22:41:59,819 SpectraRegion INFO: outclust dimensions (2655, 2655)
2020-09-30 22:41:59,821 SpectraRegion INFO: Calculating spectra similarity
2020-09-30 22:41:59,959 SpectraRegion INFO: Calculating spectra similarity done
2020-09-30 22:41:59,959 SpectraRegion INFO: Calculating dist pixel map
2020-09-30 22:42:34,879 SpectraRegion INFO: Calculating dist pixel map done
2020-09-30 22:42:34,903 SpectraRegion INFO: Calculating clusters
2020-09-30 22:42:35,018 SpectraRegion INFO: Calculating clusters done
2020-09-30 22:42:35,108 SpectraRegion INFO: Calculating clusters saved
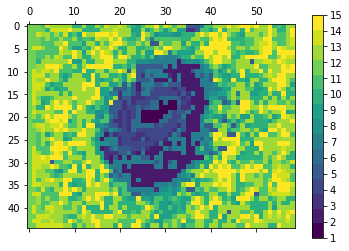
2020-09-30 22:42:35,211 SpectraRegion INFO: Assigning clusters to background: {10, 11, 12, 13, 14, 15}
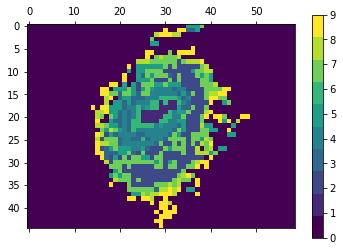
2020-09-30 22:42:35,308 SpectraRegion INFO: DE data for case: [5]
2020-09-30 22:42:35,309 SpectraRegion INFO: DE data for control: [7, 9, 3, 8, 2, 4, 6, 1]
2020-09-30 22:42:35,309 SpectraRegion INFO: Running [5] against [7, 9, 3, 8, 2, 4, 6, 1]
2020-09-30 22:42:35,310 SpectraRegion INFO: DE result key: ((5,), (1, 2, 3, 4, 6, 7, 8, 9))
2020-09-30 22:42:35,664 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 725)
2020-09-30 22:42:35,667 SpectraRegion INFO: DE Sample DataFrame ready. Shape (725, 3)
2020-09-30 22:42:35,670 SpectraRegion INFO: Performing DE-test: ttest
/usr/local/lib/python3.8/dist-packages/anndata/_core/anndata.py:119: ImplicitModificationWarning: Transforming to str index.
warnings.warn("Transforming to str index.", ImplicitModificationWarning)
2020-09-30 22:42:35,933 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((5,), (1, 2, 3, 4, 6, 7, 8, 9))
2020-09-30 22:42:35,934 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:42:40,400 SpectraRegion INFO: DE-test (rank) finished. Results available: ((5,), (1, 2, 3, 4, 6, 7, 8, 9))
2020-09-30 22:42:40,402 SpectraRegion INFO: DE result for case ((5,), (1, 2, 3, 4, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 22:42:40,402 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:42:40,406 SpectraRegion INFO: DE result for case ((5,), (1, 2, 3, 4, 6, 7, 8, 9)) with (207, 7) results (filtered)
2020-09-30 22:42:40,426 SpectraRegion INFO: Created matrices with shape (100, 17900) and (625, 17900) (target, bg)
2020-09-30 22:42:40,879 SpectraRegion INFO: DE result for case ((5,), (1, 2, 3, 4, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 22:42:40,880 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:42:40,883 SpectraRegion INFO: DE result for case ((5,), (1, 2, 3, 4, 6, 7, 8, 9)) with (126, 7) results (filtered)
2020-09-30 22:42:40,903 SpectraRegion INFO: Created matrices with shape (100, 17900) and (625, 17900) (target, bg)
2020-09-30 22:42:41,228 SpectraRegion INFO: DE data for case: [7]
2020-09-30 22:42:41,229 SpectraRegion INFO: DE data for control: [5, 9, 3, 8, 2, 4, 6, 1]
2020-09-30 22:42:41,230 SpectraRegion INFO: Running [7] against [5, 9, 3, 8, 2, 4, 6, 1]
2020-09-30 22:42:41,230 SpectraRegion INFO: DE result key: ((7,), (1, 2, 3, 4, 5, 6, 8, 9))
2020-09-30 22:42:41,588 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 725)
2020-09-30 22:42:41,590 SpectraRegion INFO: DE Sample DataFrame ready. Shape (725, 3)
2020-09-30 22:42:41,594 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:42:41,832 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((7,), (1, 2, 3, 4, 5, 6, 8, 9))
2020-09-30 22:42:41,833 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:42:46,299 SpectraRegion INFO: DE-test (rank) finished. Results available: ((7,), (1, 2, 3, 4, 5, 6, 8, 9))
2020-09-30 22:42:46,301 SpectraRegion INFO: DE result for case ((7,), (1, 2, 3, 4, 5, 6, 8, 9)) with (17900, 7) results
2020-09-30 22:42:46,302 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:42:46,305 SpectraRegion INFO: DE result for case ((7,), (1, 2, 3, 4, 5, 6, 8, 9)) with (158, 7) results (filtered)
2020-09-30 22:42:46,326 SpectraRegion INFO: Created matrices with shape (161, 17900) and (564, 17900) (target, bg)
2020-09-30 22:42:46,683 SpectraRegion INFO: DE result for case ((7,), (1, 2, 3, 4, 5, 6, 8, 9)) with (17900, 7) results
2020-09-30 22:42:46,684 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:42:46,686 SpectraRegion INFO: DE result for case ((7,), (1, 2, 3, 4, 5, 6, 8, 9)) with (125, 7) results (filtered)
2020-09-30 22:42:46,706 SpectraRegion INFO: Created matrices with shape (161, 17900) and (564, 17900) (target, bg)
2020-09-30 22:42:46,956 SpectraRegion INFO: DE data for case: [9]
2020-09-30 22:42:46,957 SpectraRegion INFO: DE data for control: [5, 7, 3, 8, 2, 4, 6, 1]
2020-09-30 22:42:46,958 SpectraRegion INFO: Running [9] against [5, 7, 3, 8, 2, 4, 6, 1]
2020-09-30 22:42:46,958 SpectraRegion INFO: DE result key: ((9,), (1, 2, 3, 4, 5, 6, 7, 8))
2020-09-30 22:42:47,303 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 725)
2020-09-30 22:42:47,305 SpectraRegion INFO: DE Sample DataFrame ready. Shape (725, 3)
2020-09-30 22:42:47,309 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:42:47,544 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((9,), (1, 2, 3, 4, 5, 6, 7, 8))
2020-09-30 22:42:47,545 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:42:52,026 SpectraRegion INFO: DE-test (rank) finished. Results available: ((9,), (1, 2, 3, 4, 5, 6, 7, 8))
2020-09-30 22:42:52,028 SpectraRegion INFO: DE result for case ((9,), (1, 2, 3, 4, 5, 6, 7, 8)) with (17900, 7) results
2020-09-30 22:42:52,029 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:42:52,032 SpectraRegion INFO: DE result for case ((9,), (1, 2, 3, 4, 5, 6, 7, 8)) with (1163, 7) results (filtered)
2020-09-30 22:42:52,051 SpectraRegion INFO: Created matrices with shape (89, 17900) and (636, 17900) (target, bg)
2020-09-30 22:42:55,168 SpectraRegion INFO: DE result for case ((9,), (1, 2, 3, 4, 5, 6, 7, 8)) with (17900, 7) results
2020-09-30 22:42:55,169 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:42:55,172 SpectraRegion INFO: DE result for case ((9,), (1, 2, 3, 4, 5, 6, 7, 8)) with (1153, 7) results (filtered)
2020-09-30 22:42:55,191 SpectraRegion INFO: Created matrices with shape (89, 17900) and (636, 17900) (target, bg)
2020-09-30 22:42:58,062 SpectraRegion INFO: DE data for case: [3]
2020-09-30 22:42:58,063 SpectraRegion INFO: DE data for control: [5, 7, 9, 8, 2, 4, 6, 1]
2020-09-30 22:42:58,064 SpectraRegion INFO: Running [3] against [5, 7, 9, 8, 2, 4, 6, 1]
2020-09-30 22:42:58,064 SpectraRegion INFO: DE result key: ((3,), (1, 2, 4, 5, 6, 7, 8, 9))
2020-09-30 22:42:58,414 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 725)
2020-09-30 22:42:58,416 SpectraRegion INFO: DE Sample DataFrame ready. Shape (725, 3)
2020-09-30 22:42:58,419 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:42:58,664 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((3,), (1, 2, 4, 5, 6, 7, 8, 9))
2020-09-30 22:42:58,664 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:43:03,157 SpectraRegion INFO: DE-test (rank) finished. Results available: ((3,), (1, 2, 4, 5, 6, 7, 8, 9))
2020-09-30 22:43:03,160 SpectraRegion INFO: DE result for case ((3,), (1, 2, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 22:43:03,161 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:43:03,164 SpectraRegion INFO: DE result for case ((3,), (1, 2, 4, 5, 6, 7, 8, 9)) with (226, 7) results (filtered)
2020-09-30 22:43:03,184 SpectraRegion INFO: Created matrices with shape (51, 17900) and (674, 17900) (target, bg)
2020-09-30 22:43:03,755 SpectraRegion INFO: DE result for case ((3,), (1, 2, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 22:43:03,756 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:43:03,759 SpectraRegion INFO: DE result for case ((3,), (1, 2, 4, 5, 6, 7, 8, 9)) with (215, 7) results (filtered)
2020-09-30 22:43:03,777 SpectraRegion INFO: Created matrices with shape (51, 17900) and (674, 17900) (target, bg)
2020-09-30 22:43:04,305 SpectraRegion INFO: DE data for case: [8]
2020-09-30 22:43:04,306 SpectraRegion INFO: DE data for control: [5, 7, 9, 3, 2, 4, 6, 1]
2020-09-30 22:43:04,307 SpectraRegion INFO: Running [8] against [5, 7, 9, 3, 2, 4, 6, 1]
2020-09-30 22:43:04,308 SpectraRegion INFO: DE result key: ((8,), (1, 2, 3, 4, 5, 6, 7, 9))
2020-09-30 22:43:04,653 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 725)
2020-09-30 22:43:04,656 SpectraRegion INFO: DE Sample DataFrame ready. Shape (725, 3)
2020-09-30 22:43:04,659 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:43:04,947 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((8,), (1, 2, 3, 4, 5, 6, 7, 9))
2020-09-30 22:43:04,949 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:43:09,524 SpectraRegion INFO: DE-test (rank) finished. Results available: ((8,), (1, 2, 3, 4, 5, 6, 7, 9))
2020-09-30 22:43:09,526 SpectraRegion INFO: DE result for case ((8,), (1, 2, 3, 4, 5, 6, 7, 9)) with (17900, 7) results
2020-09-30 22:43:09,527 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:43:09,529 SpectraRegion INFO: DE result for case ((8,), (1, 2, 3, 4, 5, 6, 7, 9)) with (671, 7) results (filtered)
2020-09-30 22:43:09,550 SpectraRegion INFO: Created matrices with shape (55, 17900) and (670, 17900) (target, bg)
2020-09-30 22:43:11,156 SpectraRegion INFO: DE result for case ((8,), (1, 2, 3, 4, 5, 6, 7, 9)) with (17900, 7) results
2020-09-30 22:43:11,156 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:43:11,159 SpectraRegion INFO: DE result for case ((8,), (1, 2, 3, 4, 5, 6, 7, 9)) with (619, 7) results (filtered)
2020-09-30 22:43:11,179 SpectraRegion INFO: Created matrices with shape (55, 17900) and (670, 17900) (target, bg)
2020-09-30 22:43:12,705 SpectraRegion INFO: DE data for case: [2]
2020-09-30 22:43:12,706 SpectraRegion INFO: DE data for control: [5, 7, 9, 3, 8, 4, 6, 1]
2020-09-30 22:43:12,707 SpectraRegion INFO: Running [2] against [5, 7, 9, 3, 8, 4, 6, 1]
2020-09-30 22:43:12,707 SpectraRegion INFO: DE result key: ((2,), (1, 3, 4, 5, 6, 7, 8, 9))
2020-09-30 22:43:13,022 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 725)
2020-09-30 22:43:13,025 SpectraRegion INFO: DE Sample DataFrame ready. Shape (725, 3)
2020-09-30 22:43:13,028 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:43:13,274 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((2,), (1, 3, 4, 5, 6, 7, 8, 9))
2020-09-30 22:43:13,274 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:43:17,938 SpectraRegion INFO: DE-test (rank) finished. Results available: ((2,), (1, 3, 4, 5, 6, 7, 8, 9))
2020-09-30 22:43:17,942 SpectraRegion INFO: DE result for case ((2,), (1, 3, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 22:43:17,943 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:43:17,946 SpectraRegion INFO: DE result for case ((2,), (1, 3, 4, 5, 6, 7, 8, 9)) with (739, 7) results (filtered)
2020-09-30 22:43:17,966 SpectraRegion INFO: Created matrices with shape (150, 17900) and (575, 17900) (target, bg)
2020-09-30 22:43:19,971 SpectraRegion INFO: DE result for case ((2,), (1, 3, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 22:43:19,972 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:43:19,974 SpectraRegion INFO: DE result for case ((2,), (1, 3, 4, 5, 6, 7, 8, 9)) with (659, 7) results (filtered)
2020-09-30 22:43:19,994 SpectraRegion INFO: Created matrices with shape (150, 17900) and (575, 17900) (target, bg)
2020-09-30 22:43:21,786 SpectraRegion INFO: DE data for case: [4]
2020-09-30 22:43:21,787 SpectraRegion INFO: DE data for control: [5, 7, 9, 3, 8, 2, 6, 1]
2020-09-30 22:43:21,787 SpectraRegion INFO: Running [4] against [5, 7, 9, 3, 8, 2, 6, 1]
2020-09-30 22:43:21,788 SpectraRegion INFO: DE result key: ((4,), (1, 2, 3, 5, 6, 7, 8, 9))
2020-09-30 22:43:22,115 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 725)
2020-09-30 22:43:22,118 SpectraRegion INFO: DE Sample DataFrame ready. Shape (725, 3)
2020-09-30 22:43:22,122 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:43:22,377 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((4,), (1, 2, 3, 5, 6, 7, 8, 9))
2020-09-30 22:43:22,378 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:43:26,863 SpectraRegion INFO: DE-test (rank) finished. Results available: ((4,), (1, 2, 3, 5, 6, 7, 8, 9))
2020-09-30 22:43:26,865 SpectraRegion INFO: DE result for case ((4,), (1, 2, 3, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 22:43:26,866 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:43:26,869 SpectraRegion INFO: DE result for case ((4,), (1, 2, 3, 5, 6, 7, 8, 9)) with (1194, 7) results (filtered)
2020-09-30 22:43:26,889 SpectraRegion INFO: Created matrices with shape (70, 17900) and (655, 17900) (target, bg)
2020-09-30 22:43:29,143 SpectraRegion INFO: DE result for case ((4,), (1, 2, 3, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 22:43:29,143 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:43:29,146 SpectraRegion INFO: DE result for case ((4,), (1, 2, 3, 5, 6, 7, 8, 9)) with (1194, 7) results (filtered)
2020-09-30 22:43:29,166 SpectraRegion INFO: Created matrices with shape (70, 17900) and (655, 17900) (target, bg)
2020-09-30 22:43:31,449 SpectraRegion INFO: DE data for case: [6]
2020-09-30 22:43:31,450 SpectraRegion INFO: DE data for control: [5, 7, 9, 3, 8, 2, 4, 1]
2020-09-30 22:43:31,450 SpectraRegion INFO: Running [6] against [5, 7, 9, 3, 8, 2, 4, 1]
2020-09-30 22:43:31,451 SpectraRegion INFO: DE result key: ((6,), (1, 2, 3, 4, 5, 7, 8, 9))
2020-09-30 22:43:31,808 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 725)
2020-09-30 22:43:31,811 SpectraRegion INFO: DE Sample DataFrame ready. Shape (725, 3)
2020-09-30 22:43:31,814 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:43:32,095 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((6,), (1, 2, 3, 4, 5, 7, 8, 9))
2020-09-30 22:43:32,096 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:43:36,630 SpectraRegion INFO: DE-test (rank) finished. Results available: ((6,), (1, 2, 3, 4, 5, 7, 8, 9))
2020-09-30 22:43:36,633 SpectraRegion INFO: DE result for case ((6,), (1, 2, 3, 4, 5, 7, 8, 9)) with (17900, 7) results
2020-09-30 22:43:36,634 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:43:36,637 SpectraRegion INFO: DE result for case ((6,), (1, 2, 3, 4, 5, 7, 8, 9)) with (876, 7) results (filtered)
2020-09-30 22:43:36,657 SpectraRegion INFO: Created matrices with shape (27, 17900) and (698, 17900) (target, bg)
2020-09-30 22:43:39,190 SpectraRegion INFO: DE result for case ((6,), (1, 2, 3, 4, 5, 7, 8, 9)) with (17900, 7) results
2020-09-30 22:43:39,191 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:43:39,194 SpectraRegion INFO: DE result for case ((6,), (1, 2, 3, 4, 5, 7, 8, 9)) with (851, 7) results (filtered)
2020-09-30 22:43:39,212 SpectraRegion INFO: Created matrices with shape (27, 17900) and (698, 17900) (target, bg)
2020-09-30 22:43:41,633 SpectraRegion INFO: DE data for case: [1]
2020-09-30 22:43:41,633 SpectraRegion INFO: DE data for control: [5, 7, 9, 3, 8, 2, 4, 6]
2020-09-30 22:43:41,634 SpectraRegion INFO: Running [1] against [5, 7, 9, 3, 8, 2, 4, 6]
2020-09-30 22:43:41,635 SpectraRegion INFO: DE result key: ((1,), (2, 3, 4, 5, 6, 7, 8, 9))
2020-09-30 22:43:41,951 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 725)
2020-09-30 22:43:41,953 SpectraRegion INFO: DE Sample DataFrame ready. Shape (725, 3)
2020-09-30 22:43:41,958 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:43:42,208 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((1,), (2, 3, 4, 5, 6, 7, 8, 9))
2020-09-30 22:43:42,209 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:43:46,705 SpectraRegion INFO: DE-test (rank) finished. Results available: ((1,), (2, 3, 4, 5, 6, 7, 8, 9))
2020-09-30 22:43:46,707 SpectraRegion INFO: DE result for case ((1,), (2, 3, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 22:43:46,708 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:43:46,711 SpectraRegion INFO: DE result for case ((1,), (2, 3, 4, 5, 6, 7, 8, 9)) with (2116, 7) results (filtered)
2020-09-30 22:43:46,730 SpectraRegion INFO: Created matrices with shape (22, 17900) and (703, 17900) (target, bg)
2020-09-30 22:43:52,934 SpectraRegion INFO: DE result for case ((1,), (2, 3, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 22:43:52,934 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:43:52,937 SpectraRegion INFO: DE result for case ((1,), (2, 3, 4, 5, 6, 7, 8, 9)) with (2057, 7) results (filtered)
2020-09-30 22:43:52,957 SpectraRegion INFO: Created matrices with shape (22, 17900) and (703, 17900) (target, bg)
2020-09-30 22:43:58,938 SpectraRegion INFO: DE data for case: [0]
2020-09-30 22:43:58,939 SpectraRegion INFO: DE data for control: [5, 7, 9, 3, 8, 2, 4, 6, 1]
2020-09-30 22:43:58,939 SpectraRegion INFO: Running [0] against [5, 7, 9, 3, 8, 2, 4, 6, 1]
2020-09-30 22:43:58,940 SpectraRegion INFO: DE result key: ((0,), (1, 2, 3, 4, 5, 6, 7, 8, 9))
2020-09-30 22:44:01,962 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 2655)
2020-09-30 22:44:01,966 SpectraRegion INFO: DE Sample DataFrame ready. Shape (2655, 3)
2020-09-30 22:44:01,970 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:44:03,054 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((0,), (1, 2, 3, 4, 5, 6, 7, 8, 9))
2020-09-30 22:44:03,054 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:44:12,751 SpectraRegion INFO: DE-test (rank) finished. Results available: ((0,), (1, 2, 3, 4, 5, 6, 7, 8, 9))
2020-09-30 22:44:12,754 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 22:44:12,755 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:44:12,757 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 6, 7, 8, 9)) with (1307, 7) results (filtered)
2020-09-30 22:44:12,820 SpectraRegion INFO: Created matrices with shape (1930, 17900) and (725, 17900) (target, bg)
2020-09-30 22:44:16,470 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 22:44:16,471 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:44:16,474 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 6, 7, 8, 9)) with (1307, 7) results (filtered)
2020-09-30 22:44:16,533 SpectraRegion INFO: Created matrices with shape (1930, 17900) and (725, 17900) (target, bg)
2020-09-30 22:44:20,095 SpectraRegion INFO: DE data for case: [5]
2020-09-30 22:44:20,095 SpectraRegion INFO: DE data for control: [0, 7, 9, 3, 8, 2, 4, 6, 1]
2020-09-30 22:44:20,096 SpectraRegion INFO: Running [5] against [0, 7, 9, 3, 8, 2, 4, 6, 1]
2020-09-30 22:44:20,096 SpectraRegion INFO: DE result key: ((5,), (0, 1, 2, 3, 4, 6, 7, 8, 9))
2020-09-30 22:44:23,299 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 2655)
2020-09-30 22:44:23,302 SpectraRegion INFO: DE Sample DataFrame ready. Shape (2655, 3)
2020-09-30 22:44:23,306 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:44:24,389 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((5,), (0, 1, 2, 3, 4, 6, 7, 8, 9))
2020-09-30 22:44:24,390 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:44:34,434 SpectraRegion INFO: DE-test (rank) finished. Results available: ((5,), (0, 1, 2, 3, 4, 6, 7, 8, 9))
2020-09-30 22:44:34,438 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 22:44:34,439 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:44:34,442 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 6, 7, 8, 9)) with (450, 7) results (filtered)
2020-09-30 22:44:34,512 SpectraRegion INFO: Created matrices with shape (100, 17900) and (2555, 17900) (target, bg)
2020-09-30 22:44:36,091 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 22:44:36,092 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:44:36,095 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 6, 7, 8, 9)) with (450, 7) results (filtered)
2020-09-30 22:44:36,171 SpectraRegion INFO: Created matrices with shape (100, 17900) and (2555, 17900) (target, bg)
2020-09-30 22:44:37,735 SpectraRegion INFO: DE data for case: [7]
2020-09-30 22:44:37,736 SpectraRegion INFO: DE data for control: [0, 5, 9, 3, 8, 2, 4, 6, 1]
2020-09-30 22:44:37,736 SpectraRegion INFO: Running [7] against [0, 5, 9, 3, 8, 2, 4, 6, 1]
2020-09-30 22:44:37,737 SpectraRegion INFO: DE result key: ((7,), (0, 1, 2, 3, 4, 5, 6, 8, 9))
2020-09-30 22:44:40,747 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 2655)
2020-09-30 22:44:40,750 SpectraRegion INFO: DE Sample DataFrame ready. Shape (2655, 3)
2020-09-30 22:44:40,754 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:44:41,814 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((7,), (0, 1, 2, 3, 4, 5, 6, 8, 9))
2020-09-30 22:44:41,815 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:44:51,507 SpectraRegion INFO: DE-test (rank) finished. Results available: ((7,), (0, 1, 2, 3, 4, 5, 6, 8, 9))
2020-09-30 22:44:51,510 SpectraRegion INFO: DE result for case ((7,), (0, 1, 2, 3, 4, 5, 6, 8, 9)) with (17900, 7) results
2020-09-30 22:44:51,511 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:44:51,513 SpectraRegion INFO: DE result for case ((7,), (0, 1, 2, 3, 4, 5, 6, 8, 9)) with (680, 7) results (filtered)
2020-09-30 22:44:51,575 SpectraRegion INFO: Created matrices with shape (161, 17900) and (2494, 17900) (target, bg)
2020-09-30 22:44:53,629 SpectraRegion INFO: DE result for case ((7,), (0, 1, 2, 3, 4, 5, 6, 8, 9)) with (17900, 7) results
2020-09-30 22:44:53,629 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:44:53,632 SpectraRegion INFO: DE result for case ((7,), (0, 1, 2, 3, 4, 5, 6, 8, 9)) with (680, 7) results (filtered)
2020-09-30 22:44:53,700 SpectraRegion INFO: Created matrices with shape (161, 17900) and (2494, 17900) (target, bg)
2020-09-30 22:44:55,663 SpectraRegion INFO: DE data for case: [9]
2020-09-30 22:44:55,664 SpectraRegion INFO: DE data for control: [0, 5, 7, 3, 8, 2, 4, 6, 1]
2020-09-30 22:44:55,665 SpectraRegion INFO: Running [9] against [0, 5, 7, 3, 8, 2, 4, 6, 1]
2020-09-30 22:44:55,665 SpectraRegion INFO: DE result key: ((9,), (0, 1, 2, 3, 4, 5, 6, 7, 8))
2020-09-30 22:44:59,028 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 2655)
2020-09-30 22:44:59,031 SpectraRegion INFO: DE Sample DataFrame ready. Shape (2655, 3)
2020-09-30 22:44:59,036 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:45:00,122 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((9,), (0, 1, 2, 3, 4, 5, 6, 7, 8))
2020-09-30 22:45:00,123 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:45:10,082 SpectraRegion INFO: DE-test (rank) finished. Results available: ((9,), (0, 1, 2, 3, 4, 5, 6, 7, 8))
2020-09-30 22:45:10,085 SpectraRegion INFO: DE result for case ((9,), (0, 1, 2, 3, 4, 5, 6, 7, 8)) with (17900, 7) results
2020-09-30 22:45:10,086 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:45:10,088 SpectraRegion INFO: DE result for case ((9,), (0, 1, 2, 3, 4, 5, 6, 7, 8)) with (88, 7) results (filtered)
2020-09-30 22:45:10,149 SpectraRegion INFO: Created matrices with shape (89, 17900) and (2566, 17900) (target, bg)
2020-09-30 22:45:10,246 SpectraRegion INFO: DE result for case ((9,), (0, 1, 2, 3, 4, 5, 6, 7, 8)) with (17900, 7) results
2020-09-30 22:45:10,247 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:45:10,251 SpectraRegion INFO: DE result for case ((9,), (0, 1, 2, 3, 4, 5, 6, 7, 8)) with (65, 7) results (filtered)
2020-09-30 22:45:10,322 SpectraRegion INFO: Created matrices with shape (89, 17900) and (2566, 17900) (target, bg)
2020-09-30 22:45:10,389 SpectraRegion INFO: DE data for case: [3]
2020-09-30 22:45:10,390 SpectraRegion INFO: DE data for control: [0, 5, 7, 9, 8, 2, 4, 6, 1]
2020-09-30 22:45:10,390 SpectraRegion INFO: Running [3] against [0, 5, 7, 9, 8, 2, 4, 6, 1]
2020-09-30 22:45:10,391 SpectraRegion INFO: DE result key: ((3,), (0, 1, 2, 4, 5, 6, 7, 8, 9))
2020-09-30 22:45:13,479 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 2655)
2020-09-30 22:45:13,483 SpectraRegion INFO: DE Sample DataFrame ready. Shape (2655, 3)
2020-09-30 22:45:13,487 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:45:14,728 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((3,), (0, 1, 2, 4, 5, 6, 7, 8, 9))
2020-09-30 22:45:14,729 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:45:24,500 SpectraRegion INFO: DE-test (rank) finished. Results available: ((3,), (0, 1, 2, 4, 5, 6, 7, 8, 9))
2020-09-30 22:45:24,504 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 22:45:24,504 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:45:24,507 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 6, 7, 8, 9)) with (1263, 7) results (filtered)
2020-09-30 22:45:24,567 SpectraRegion INFO: Created matrices with shape (51, 17900) and (2604, 17900) (target, bg)
2020-09-30 22:45:28,347 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 22:45:28,348 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:45:28,351 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 6, 7, 8, 9)) with (1261, 7) results (filtered)
2020-09-30 22:45:28,419 SpectraRegion INFO: Created matrices with shape (51, 17900) and (2604, 17900) (target, bg)
2020-09-30 22:45:32,101 SpectraRegion INFO: DE data for case: [8]
2020-09-30 22:45:32,102 SpectraRegion INFO: DE data for control: [0, 5, 7, 9, 3, 2, 4, 6, 1]
2020-09-30 22:45:32,103 SpectraRegion INFO: Running [8] against [0, 5, 7, 9, 3, 2, 4, 6, 1]
2020-09-30 22:45:32,103 SpectraRegion INFO: DE result key: ((8,), (0, 1, 2, 3, 4, 5, 6, 7, 9))
2020-09-30 22:45:35,400 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 2655)
2020-09-30 22:45:35,403 SpectraRegion INFO: DE Sample DataFrame ready. Shape (2655, 3)
2020-09-30 22:45:35,407 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:45:36,533 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((8,), (0, 1, 2, 3, 4, 5, 6, 7, 9))
2020-09-30 22:45:36,534 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:45:46,385 SpectraRegion INFO: DE-test (rank) finished. Results available: ((8,), (0, 1, 2, 3, 4, 5, 6, 7, 9))
2020-09-30 22:45:46,389 SpectraRegion INFO: DE result for case ((8,), (0, 1, 2, 3, 4, 5, 6, 7, 9)) with (17900, 7) results
2020-09-30 22:45:46,389 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:45:46,392 SpectraRegion INFO: DE result for case ((8,), (0, 1, 2, 3, 4, 5, 6, 7, 9)) with (253, 7) results (filtered)
2020-09-30 22:45:46,452 SpectraRegion INFO: Created matrices with shape (55, 17900) and (2600, 17900) (target, bg)
2020-09-30 22:45:46,931 SpectraRegion INFO: DE result for case ((8,), (0, 1, 2, 3, 4, 5, 6, 7, 9)) with (17900, 7) results
2020-09-30 22:45:46,932 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:45:46,934 SpectraRegion INFO: DE result for case ((8,), (0, 1, 2, 3, 4, 5, 6, 7, 9)) with (250, 7) results (filtered)
2020-09-30 22:45:46,994 SpectraRegion INFO: Created matrices with shape (55, 17900) and (2600, 17900) (target, bg)
2020-09-30 22:45:47,441 SpectraRegion INFO: DE data for case: [2]
2020-09-30 22:45:47,442 SpectraRegion INFO: DE data for control: [0, 5, 7, 9, 3, 8, 4, 6, 1]
2020-09-30 22:45:47,443 SpectraRegion INFO: Running [2] against [0, 5, 7, 9, 3, 8, 4, 6, 1]
2020-09-30 22:45:47,443 SpectraRegion INFO: DE result key: ((2,), (0, 1, 3, 4, 5, 6, 7, 8, 9))
2020-09-30 22:45:50,457 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 2655)
2020-09-30 22:45:50,461 SpectraRegion INFO: DE Sample DataFrame ready. Shape (2655, 3)
2020-09-30 22:45:50,466 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:45:51,702 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((2,), (0, 1, 3, 4, 5, 6, 7, 8, 9))
2020-09-30 22:45:51,702 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:46:01,520 SpectraRegion INFO: DE-test (rank) finished. Results available: ((2,), (0, 1, 3, 4, 5, 6, 7, 8, 9))
2020-09-30 22:46:01,523 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 22:46:01,524 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:46:01,526 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 6, 7, 8, 9)) with (1796, 7) results (filtered)
2020-09-30 22:46:01,589 SpectraRegion INFO: Created matrices with shape (150, 17900) and (2505, 17900) (target, bg)
2020-09-30 22:46:07,617 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 22:46:07,618 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:46:07,621 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 6, 7, 8, 9)) with (1796, 7) results (filtered)
2020-09-30 22:46:07,683 SpectraRegion INFO: Created matrices with shape (150, 17900) and (2505, 17900) (target, bg)
2020-09-30 22:46:13,652 SpectraRegion INFO: DE data for case: [4]
2020-09-30 22:46:13,653 SpectraRegion INFO: DE data for control: [0, 5, 7, 9, 3, 8, 2, 6, 1]
2020-09-30 22:46:13,653 SpectraRegion INFO: Running [4] against [0, 5, 7, 9, 3, 8, 2, 6, 1]
2020-09-30 22:46:13,653 SpectraRegion INFO: DE result key: ((4,), (0, 1, 2, 3, 5, 6, 7, 8, 9))
2020-09-30 22:46:16,729 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 2655)
2020-09-30 22:46:16,732 SpectraRegion INFO: DE Sample DataFrame ready. Shape (2655, 3)
2020-09-30 22:46:16,737 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:46:17,761 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((4,), (0, 1, 2, 3, 5, 6, 7, 8, 9))
2020-09-30 22:46:17,762 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:46:27,516 SpectraRegion INFO: DE-test (rank) finished. Results available: ((4,), (0, 1, 2, 3, 5, 6, 7, 8, 9))
2020-09-30 22:46:27,520 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 22:46:27,520 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:46:27,523 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 6, 7, 8, 9)) with (1742, 7) results (filtered)
2020-09-30 22:46:27,588 SpectraRegion INFO: Created matrices with shape (70, 17900) and (2585, 17900) (target, bg)
2020-09-30 22:46:31,784 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 22:46:31,785 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:46:31,787 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 6, 7, 8, 9)) with (1742, 7) results (filtered)
2020-09-30 22:46:31,853 SpectraRegion INFO: Created matrices with shape (70, 17900) and (2585, 17900) (target, bg)
2020-09-30 22:46:35,893 SpectraRegion INFO: DE data for case: [6]
2020-09-30 22:46:35,893 SpectraRegion INFO: DE data for control: [0, 5, 7, 9, 3, 8, 2, 4, 1]
2020-09-30 22:46:35,894 SpectraRegion INFO: Running [6] against [0, 5, 7, 9, 3, 8, 2, 4, 1]
2020-09-30 22:46:35,894 SpectraRegion INFO: DE result key: ((6,), (0, 1, 2, 3, 4, 5, 7, 8, 9))
2020-09-30 22:46:39,320 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 2655)
2020-09-30 22:46:39,323 SpectraRegion INFO: DE Sample DataFrame ready. Shape (2655, 3)
2020-09-30 22:46:39,328 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:46:40,637 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((6,), (0, 1, 2, 3, 4, 5, 7, 8, 9))
2020-09-30 22:46:40,637 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:46:50,310 SpectraRegion INFO: DE-test (rank) finished. Results available: ((6,), (0, 1, 2, 3, 4, 5, 7, 8, 9))
2020-09-30 22:46:50,313 SpectraRegion INFO: DE result for case ((6,), (0, 1, 2, 3, 4, 5, 7, 8, 9)) with (17900, 7) results
2020-09-30 22:46:50,314 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:46:50,317 SpectraRegion INFO: DE result for case ((6,), (0, 1, 2, 3, 4, 5, 7, 8, 9)) with (2053, 7) results (filtered)
2020-09-30 22:46:50,380 SpectraRegion INFO: Created matrices with shape (27, 17900) and (2628, 17900) (target, bg)
2020-09-30 22:46:56,745 SpectraRegion INFO: DE result for case ((6,), (0, 1, 2, 3, 4, 5, 7, 8, 9)) with (17900, 7) results
2020-09-30 22:46:56,746 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:46:56,749 SpectraRegion INFO: DE result for case ((6,), (0, 1, 2, 3, 4, 5, 7, 8, 9)) with (2058, 7) results (filtered)
2020-09-30 22:46:56,809 SpectraRegion INFO: Created matrices with shape (27, 17900) and (2628, 17900) (target, bg)
2020-09-30 22:47:02,995 SpectraRegion INFO: DE data for case: [1]
2020-09-30 22:47:02,996 SpectraRegion INFO: DE data for control: [0, 5, 7, 9, 3, 8, 2, 4, 6]
2020-09-30 22:47:02,997 SpectraRegion INFO: Running [1] against [0, 5, 7, 9, 3, 8, 2, 4, 6]
2020-09-30 22:47:02,997 SpectraRegion INFO: DE result key: ((1,), (0, 2, 3, 4, 5, 6, 7, 8, 9))
2020-09-30 22:47:06,106 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 2655)
2020-09-30 22:47:06,109 SpectraRegion INFO: DE Sample DataFrame ready. Shape (2655, 3)
2020-09-30 22:47:06,113 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:47:07,177 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((1,), (0, 2, 3, 4, 5, 6, 7, 8, 9))
2020-09-30 22:47:07,178 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:47:17,169 SpectraRegion INFO: DE-test (rank) finished. Results available: ((1,), (0, 2, 3, 4, 5, 6, 7, 8, 9))
2020-09-30 22:47:17,173 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 22:47:17,174 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:47:17,177 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 6, 7, 8, 9)) with (3036, 7) results (filtered)
2020-09-30 22:47:17,239 SpectraRegion INFO: Created matrices with shape (22, 17900) and (2633, 17900) (target, bg)
2020-09-30 22:47:27,123 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 22:47:27,124 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:47:27,127 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 6, 7, 8, 9)) with (3039, 7) results (filtered)
2020-09-30 22:47:27,186 SpectraRegion INFO: Created matrices with shape (22, 17900) and (2633, 17900) (target, bg)
Fetching region range
Fetching region shape
Found region 1 with shape (43, 56, 17900)
Fetching region spectra
3% (92 of 2408) | | Elapsed Time: 0:00:00 ETA: 0:00:03
Processing regionID 1 for basename slideD
100% (2408 of 2408) |####################| Elapsed Time: 0:00:04 Time: 0:00:04
100% (2408 of 2408) |####################| Elapsed Time: 0:00:00 Time: 0:00:00
Started Log Value: 0.17063884288072587
100% (43 of 43) |########################| Elapsed Time: 0:00:00 Time: 0:00:00
Got 2408 median-enabled pixels
5-Number stats for medians: (2408, 2408, 0.3039763115205163, 0.5889737648019792, 0.6580512343895084, 0.7246390240951075, 1.0161616910876121)
Started Log Value: 0.2648863598704338
Collecting fold changes
100% (43 of 43) |########################| Elapsed Time: 0:00:15 Time: 0:00:15
Got a total of 43103200 fold changes
Median elements [21551600]
Median elements
Global Median 0.64556
2020-09-30 22:48:00,321 SpectraRegion INFO: dimensions inputarray: 17900
2020-09-30 22:48:00,322 SpectraRegion INFO: Creating C++ obj
2020-09-30 22:48:00,322 SpectraRegion INFO: 17900 (43, 56, 17900)
2020-09-30 22:48:00,323 SpectraRegion INFO: dimensions 17900
2020-09-30 22:48:00,324 SpectraRegion INFO: input dimensions (43, 56, 17900)
2020-09-30 22:48:00,325 SpectraRegion INFO: Switching to dot mode
2020-09-30 22:48:00,380 SpectraRegion INFO: Starting calc similarity c++
2020-09-30 22:48:39,976 SpectraRegion INFO: outclust dimensions (2408, 2408)
2020-09-30 22:48:39,991 SpectraRegion INFO: Calculating spectra similarity
2020-09-30 22:48:40,029 SpectraRegion INFO: Calculating spectra similarity done
2020-09-30 22:48:40,030 SpectraRegion INFO: Calculating dist pixel map
2020-09-30 22:49:11,429 SpectraRegion INFO: Calculating dist pixel map done
2020-09-30 22:49:11,448 SpectraRegion INFO: Calculating clusters
2020-09-30 22:49:11,559 SpectraRegion INFO: Calculating clusters done
2020-09-30 22:49:11,610 SpectraRegion INFO: Calculating clusters saved
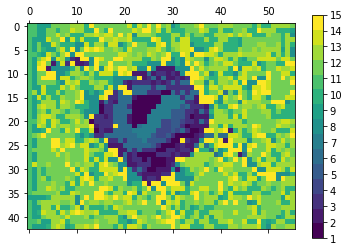
2020-09-30 22:49:11,718 SpectraRegion INFO: Assigning clusters to background: {8, 9, 10, 11, 12, 13, 14}
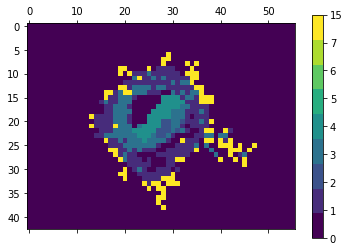
2020-09-30 22:49:11,815 SpectraRegion INFO: DE data for case: [15]
2020-09-30 22:49:11,816 SpectraRegion INFO: DE data for control: [2, 6, 3, 1, 7, 5, 4]
2020-09-30 22:49:11,817 SpectraRegion INFO: Running [15] against [2, 6, 3, 1, 7, 5, 4]
2020-09-30 22:49:11,817 SpectraRegion INFO: DE result key: ((15,), (1, 2, 3, 4, 5, 6, 7))
2020-09-30 22:49:11,998 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 485)
2020-09-30 22:49:12,000 SpectraRegion INFO: DE Sample DataFrame ready. Shape (485, 3)
2020-09-30 22:49:12,004 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:49:12,165 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((15,), (1, 2, 3, 4, 5, 6, 7))
2020-09-30 22:49:12,166 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:49:16,161 SpectraRegion INFO: DE-test (rank) finished. Results available: ((15,), (1, 2, 3, 4, 5, 6, 7))
2020-09-30 22:49:16,163 SpectraRegion INFO: DE result for case ((15,), (1, 2, 3, 4, 5, 6, 7)) with (17900, 7) results
2020-09-30 22:49:16,164 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:49:16,167 SpectraRegion INFO: DE result for case ((15,), (1, 2, 3, 4, 5, 6, 7)) with (569, 7) results (filtered)
2020-09-30 22:49:16,182 SpectraRegion INFO: Created matrices with shape (91, 17900) and (394, 17900) (target, bg)
2020-09-30 22:49:17,585 SpectraRegion INFO: DE result for case ((15,), (1, 2, 3, 4, 5, 6, 7)) with (17900, 7) results
2020-09-30 22:49:17,585 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:49:17,588 SpectraRegion INFO: DE result for case ((15,), (1, 2, 3, 4, 5, 6, 7)) with (569, 7) results (filtered)
2020-09-30 22:49:17,602 SpectraRegion INFO: Created matrices with shape (91, 17900) and (394, 17900) (target, bg)
2020-09-30 22:49:18,996 SpectraRegion INFO: DE data for case: [2]
2020-09-30 22:49:18,997 SpectraRegion INFO: DE data for control: [15, 6, 3, 1, 7, 5, 4]
2020-09-30 22:49:18,997 SpectraRegion INFO: Running [2] against [15, 6, 3, 1, 7, 5, 4]
2020-09-30 22:49:18,998 SpectraRegion INFO: DE result key: ((2,), (1, 3, 4, 5, 6, 7, 15))
2020-09-30 22:49:19,183 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 485)
2020-09-30 22:49:19,186 SpectraRegion INFO: DE Sample DataFrame ready. Shape (485, 3)
2020-09-30 22:49:19,190 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:49:19,350 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((2,), (1, 3, 4, 5, 6, 7, 15))
2020-09-30 22:49:19,351 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:49:23,360 SpectraRegion INFO: DE-test (rank) finished. Results available: ((2,), (1, 3, 4, 5, 6, 7, 15))
2020-09-30 22:49:23,362 SpectraRegion INFO: DE result for case ((2,), (1, 3, 4, 5, 6, 7, 15)) with (17900, 7) results
2020-09-30 22:49:23,363 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:49:23,366 SpectraRegion INFO: DE result for case ((2,), (1, 3, 4, 5, 6, 7, 15)) with (140, 7) results (filtered)
2020-09-30 22:49:23,382 SpectraRegion INFO: Created matrices with shape (79, 17900) and (406, 17900) (target, bg)
2020-09-30 22:49:23,548 SpectraRegion INFO: DE result for case ((2,), (1, 3, 4, 5, 6, 7, 15)) with (17900, 7) results
2020-09-30 22:49:23,549 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:49:23,552 SpectraRegion INFO: DE result for case ((2,), (1, 3, 4, 5, 6, 7, 15)) with (137, 7) results (filtered)
2020-09-30 22:49:23,568 SpectraRegion INFO: Created matrices with shape (79, 17900) and (406, 17900) (target, bg)
2020-09-30 22:49:23,726 SpectraRegion INFO: DE data for case: [6]
2020-09-30 22:49:23,727 SpectraRegion INFO: DE data for control: [15, 2, 3, 1, 7, 5, 4]
2020-09-30 22:49:23,728 SpectraRegion INFO: Running [6] against [15, 2, 3, 1, 7, 5, 4]
2020-09-30 22:49:23,728 SpectraRegion INFO: DE result key: ((6,), (1, 2, 3, 4, 5, 7, 15))
2020-09-30 22:49:23,907 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 485)
2020-09-30 22:49:23,910 SpectraRegion INFO: DE Sample DataFrame ready. Shape (485, 3)
2020-09-30 22:49:23,914 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:49:24,075 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((6,), (1, 2, 3, 4, 5, 7, 15))
2020-09-30 22:49:24,076 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:49:28,211 SpectraRegion INFO: DE-test (rank) finished. Results available: ((6,), (1, 2, 3, 4, 5, 7, 15))
2020-09-30 22:49:28,213 SpectraRegion INFO: DE result for case ((6,), (1, 2, 3, 4, 5, 7, 15)) with (17900, 7) results
2020-09-30 22:49:28,214 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:49:28,217 SpectraRegion INFO: DE result for case ((6,), (1, 2, 3, 4, 5, 7, 15)) with (291, 7) results (filtered)
2020-09-30 22:49:28,235 SpectraRegion INFO: Created matrices with shape (86, 17900) and (399, 17900) (target, bg)
2020-09-30 22:49:29,050 SpectraRegion INFO: DE result for case ((6,), (1, 2, 3, 4, 5, 7, 15)) with (17900, 7) results
2020-09-30 22:49:29,051 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:49:29,054 SpectraRegion INFO: DE result for case ((6,), (1, 2, 3, 4, 5, 7, 15)) with (290, 7) results (filtered)
2020-09-30 22:49:29,073 SpectraRegion INFO: Created matrices with shape (86, 17900) and (399, 17900) (target, bg)
2020-09-30 22:49:29,867 SpectraRegion INFO: DE data for case: [3]
2020-09-30 22:49:29,868 SpectraRegion INFO: DE data for control: [15, 2, 6, 1, 7, 5, 4]
2020-09-30 22:49:29,868 SpectraRegion INFO: Running [3] against [15, 2, 6, 1, 7, 5, 4]
2020-09-30 22:49:29,869 SpectraRegion INFO: DE result key: ((3,), (1, 2, 4, 5, 6, 7, 15))
2020-09-30 22:49:30,048 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 485)
2020-09-30 22:49:30,051 SpectraRegion INFO: DE Sample DataFrame ready. Shape (485, 3)
2020-09-30 22:49:30,054 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:49:30,218 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((3,), (1, 2, 4, 5, 6, 7, 15))
2020-09-30 22:49:30,218 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:49:34,366 SpectraRegion INFO: DE-test (rank) finished. Results available: ((3,), (1, 2, 4, 5, 6, 7, 15))
2020-09-30 22:49:34,371 SpectraRegion INFO: DE result for case ((3,), (1, 2, 4, 5, 6, 7, 15)) with (17900, 7) results
2020-09-30 22:49:34,372 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:49:34,377 SpectraRegion INFO: DE result for case ((3,), (1, 2, 4, 5, 6, 7, 15)) with (42, 7) results (filtered)
2020-09-30 22:49:34,400 SpectraRegion INFO: Created matrices with shape (99, 17900) and (386, 17900) (target, bg)
2020-09-30 22:49:34,447 SpectraRegion INFO: DE result for case ((3,), (1, 2, 4, 5, 6, 7, 15)) with (17900, 7) results
2020-09-30 22:49:34,448 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:49:34,450 SpectraRegion INFO: DE result for case ((3,), (1, 2, 4, 5, 6, 7, 15)) with (21, 7) results (filtered)
2020-09-30 22:49:34,469 SpectraRegion INFO: Created matrices with shape (99, 17900) and (386, 17900) (target, bg)
2020-09-30 22:49:34,509 SpectraRegion INFO: DE data for case: [1]
2020-09-30 22:49:34,510 SpectraRegion INFO: DE data for control: [15, 2, 6, 3, 7, 5, 4]
2020-09-30 22:49:34,511 SpectraRegion INFO: Running [1] against [15, 2, 6, 3, 7, 5, 4]
2020-09-30 22:49:34,512 SpectraRegion INFO: DE result key: ((1,), (2, 3, 4, 5, 6, 7, 15))
2020-09-30 22:49:34,703 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 485)
2020-09-30 22:49:34,706 SpectraRegion INFO: DE Sample DataFrame ready. Shape (485, 3)
2020-09-30 22:49:34,711 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:49:34,894 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((1,), (2, 3, 4, 5, 6, 7, 15))
2020-09-30 22:49:34,895 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:49:38,951 SpectraRegion INFO: DE-test (rank) finished. Results available: ((1,), (2, 3, 4, 5, 6, 7, 15))
2020-09-30 22:49:38,952 SpectraRegion INFO: DE result for case ((1,), (2, 3, 4, 5, 6, 7, 15)) with (17900, 7) results
2020-09-30 22:49:38,953 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:49:38,956 SpectraRegion INFO: DE result for case ((1,), (2, 3, 4, 5, 6, 7, 15)) with (778, 7) results (filtered)
2020-09-30 22:49:38,971 SpectraRegion INFO: Created matrices with shape (61, 17900) and (424, 17900) (target, bg)
2020-09-30 22:49:40,992 SpectraRegion INFO: DE result for case ((1,), (2, 3, 4, 5, 6, 7, 15)) with (17900, 7) results
2020-09-30 22:49:40,992 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:49:40,996 SpectraRegion INFO: DE result for case ((1,), (2, 3, 4, 5, 6, 7, 15)) with (766, 7) results (filtered)
2020-09-30 22:49:41,013 SpectraRegion INFO: Created matrices with shape (61, 17900) and (424, 17900) (target, bg)
2020-09-30 22:49:42,987 SpectraRegion INFO: DE data for case: [7]
2020-09-30 22:49:42,988 SpectraRegion INFO: DE data for control: [15, 2, 6, 3, 1, 5, 4]
2020-09-30 22:49:42,988 SpectraRegion INFO: Running [7] against [15, 2, 6, 3, 1, 5, 4]
2020-09-30 22:49:42,989 SpectraRegion INFO: DE result key: ((7,), (1, 2, 3, 4, 5, 6, 15))
2020-09-30 22:49:43,183 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 485)
2020-09-30 22:49:43,189 SpectraRegion INFO: DE Sample DataFrame ready. Shape (485, 3)
2020-09-30 22:49:43,198 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:49:43,371 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((7,), (1, 2, 3, 4, 5, 6, 15))
2020-09-30 22:49:43,371 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:49:47,358 SpectraRegion INFO: DE-test (rank) finished. Results available: ((7,), (1, 2, 3, 4, 5, 6, 15))
2020-09-30 22:49:47,360 SpectraRegion INFO: DE result for case ((7,), (1, 2, 3, 4, 5, 6, 15)) with (17900, 7) results
2020-09-30 22:49:47,360 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:49:47,363 SpectraRegion INFO: DE result for case ((7,), (1, 2, 3, 4, 5, 6, 15)) with (1026, 7) results (filtered)
2020-09-30 22:49:47,378 SpectraRegion INFO: Created matrices with shape (39, 17900) and (446, 17900) (target, bg)
2020-09-30 22:49:49,818 SpectraRegion INFO: DE result for case ((7,), (1, 2, 3, 4, 5, 6, 15)) with (17900, 7) results
2020-09-30 22:49:49,819 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:49:49,822 SpectraRegion INFO: DE result for case ((7,), (1, 2, 3, 4, 5, 6, 15)) with (1027, 7) results (filtered)
2020-09-30 22:49:49,837 SpectraRegion INFO: Created matrices with shape (39, 17900) and (446, 17900) (target, bg)
2020-09-30 22:49:52,279 SpectraRegion INFO: DE data for case: [5]
2020-09-30 22:49:52,279 SpectraRegion INFO: DE data for control: [15, 2, 6, 3, 1, 7, 4]
2020-09-30 22:49:52,280 SpectraRegion INFO: Running [5] against [15, 2, 6, 3, 1, 7, 4]
2020-09-30 22:49:52,280 SpectraRegion INFO: DE result key: ((5,), (1, 2, 3, 4, 6, 7, 15))
2020-09-30 22:49:52,460 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 485)
2020-09-30 22:49:52,462 SpectraRegion INFO: DE Sample DataFrame ready. Shape (485, 3)
2020-09-30 22:49:52,467 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:49:52,631 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((5,), (1, 2, 3, 4, 6, 7, 15))
2020-09-30 22:49:52,631 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:49:56,658 SpectraRegion INFO: DE-test (rank) finished. Results available: ((5,), (1, 2, 3, 4, 6, 7, 15))
2020-09-30 22:49:56,660 SpectraRegion INFO: DE result for case ((5,), (1, 2, 3, 4, 6, 7, 15)) with (17900, 7) results
2020-09-30 22:49:56,661 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:49:56,663 SpectraRegion INFO: DE result for case ((5,), (1, 2, 3, 4, 6, 7, 15)) with (1536, 7) results (filtered)
2020-09-30 22:49:56,677 SpectraRegion INFO: Created matrices with shape (19, 17900) and (466, 17900) (target, bg)
2020-09-30 22:50:00,203 SpectraRegion INFO: DE result for case ((5,), (1, 2, 3, 4, 6, 7, 15)) with (17900, 7) results
2020-09-30 22:50:00,204 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:50:00,207 SpectraRegion INFO: DE result for case ((5,), (1, 2, 3, 4, 6, 7, 15)) with (1536, 7) results (filtered)
2020-09-30 22:50:00,223 SpectraRegion INFO: Created matrices with shape (19, 17900) and (466, 17900) (target, bg)
2020-09-30 22:50:03,371 SpectraRegion INFO: DE data for case: [4]
2020-09-30 22:50:03,372 SpectraRegion INFO: DE data for control: [15, 2, 6, 3, 1, 7, 5]
2020-09-30 22:50:03,372 SpectraRegion INFO: Running [4] against [15, 2, 6, 3, 1, 7, 5]
2020-09-30 22:50:03,373 SpectraRegion INFO: DE result key: ((4,), (1, 2, 3, 5, 6, 7, 15))
2020-09-30 22:50:03,552 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 485)
2020-09-30 22:50:03,554 SpectraRegion INFO: DE Sample DataFrame ready. Shape (485, 3)
2020-09-30 22:50:03,557 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:50:03,729 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((4,), (1, 2, 3, 5, 6, 7, 15))
2020-09-30 22:50:03,729 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:50:07,893 SpectraRegion INFO: DE-test (rank) finished. Results available: ((4,), (1, 2, 3, 5, 6, 7, 15))
2020-09-30 22:50:07,895 SpectraRegion INFO: DE result for case ((4,), (1, 2, 3, 5, 6, 7, 15)) with (17900, 7) results
2020-09-30 22:50:07,896 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:50:07,899 SpectraRegion INFO: DE result for case ((4,), (1, 2, 3, 5, 6, 7, 15)) with (419, 7) results (filtered)
2020-09-30 22:50:07,913 SpectraRegion INFO: Created matrices with shape (11, 17900) and (474, 17900) (target, bg)
2020-09-30 22:50:09,207 SpectraRegion INFO: DE result for case ((4,), (1, 2, 3, 5, 6, 7, 15)) with (17900, 7) results
2020-09-30 22:50:09,208 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:50:09,211 SpectraRegion INFO: DE result for case ((4,), (1, 2, 3, 5, 6, 7, 15)) with (354, 7) results (filtered)
2020-09-30 22:50:09,227 SpectraRegion INFO: Created matrices with shape (11, 17900) and (474, 17900) (target, bg)
2020-09-30 22:50:10,123 SpectraRegion INFO: DE data for case: [0]
2020-09-30 22:50:10,124 SpectraRegion INFO: DE data for control: [15, 2, 6, 3, 1, 7, 5, 4]
2020-09-30 22:50:10,124 SpectraRegion INFO: Running [0] against [15, 2, 6, 3, 1, 7, 5, 4]
2020-09-30 22:50:10,125 SpectraRegion INFO: DE result key: ((0,), (1, 2, 3, 4, 5, 6, 7, 15))
2020-09-30 22:50:12,878 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 2408)
2020-09-30 22:50:12,881 SpectraRegion INFO: DE Sample DataFrame ready. Shape (2408, 3)
2020-09-30 22:50:12,887 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:50:13,890 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((0,), (1, 2, 3, 4, 5, 6, 7, 15))
2020-09-30 22:50:13,890 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:50:22,877 SpectraRegion INFO: DE-test (rank) finished. Results available: ((0,), (1, 2, 3, 4, 5, 6, 7, 15))
2020-09-30 22:50:22,880 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 6, 7, 15)) with (17900, 7) results
2020-09-30 22:50:22,881 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:50:22,885 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 6, 7, 15)) with (1086, 7) results (filtered)
2020-09-30 22:50:22,945 SpectraRegion INFO: Created matrices with shape (1923, 17900) and (485, 17900) (target, bg)
2020-09-30 22:50:26,042 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 6, 7, 15)) with (17900, 7) results
2020-09-30 22:50:26,043 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:50:26,046 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 6, 7, 15)) with (1086, 7) results (filtered)
2020-09-30 22:50:26,107 SpectraRegion INFO: Created matrices with shape (1923, 17900) and (485, 17900) (target, bg)
2020-09-30 22:50:29,195 SpectraRegion INFO: DE data for case: [15]
2020-09-30 22:50:29,195 SpectraRegion INFO: DE data for control: [0, 2, 6, 3, 1, 7, 5, 4]
2020-09-30 22:50:29,196 SpectraRegion INFO: Running [15] against [0, 2, 6, 3, 1, 7, 5, 4]
2020-09-30 22:50:29,196 SpectraRegion INFO: DE result key: ((15,), (0, 1, 2, 3, 4, 5, 6, 7))
2020-09-30 22:50:31,998 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 2408)
2020-09-30 22:50:32,002 SpectraRegion INFO: DE Sample DataFrame ready. Shape (2408, 3)
2020-09-30 22:50:32,005 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:50:32,936 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((15,), (0, 1, 2, 3, 4, 5, 6, 7))
2020-09-30 22:50:32,937 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:50:42,240 SpectraRegion INFO: DE-test (rank) finished. Results available: ((15,), (0, 1, 2, 3, 4, 5, 6, 7))
2020-09-30 22:50:42,244 SpectraRegion INFO: DE result for case ((15,), (0, 1, 2, 3, 4, 5, 6, 7)) with (17900, 7) results
2020-09-30 22:50:42,245 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:50:42,247 SpectraRegion INFO: DE result for case ((15,), (0, 1, 2, 3, 4, 5, 6, 7)) with (12, 7) results (filtered)
2020-09-30 22:50:42,308 SpectraRegion INFO: Created matrices with shape (91, 17900) and (2317, 17900) (target, bg)
2020-09-30 22:50:42,326 SpectraRegion INFO: DE result for case ((15,), (0, 1, 2, 3, 4, 5, 6, 7)) with (17900, 7) results
2020-09-30 22:50:42,327 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:50:42,329 SpectraRegion INFO: DE result for case ((15,), (0, 1, 2, 3, 4, 5, 6, 7)) with (12, 7) results (filtered)
2020-09-30 22:50:42,613 SpectraRegion INFO: Created matrices with shape (91, 17900) and (2317, 17900) (target, bg)
2020-09-30 22:50:42,633 SpectraRegion INFO: DE data for case: [2]
2020-09-30 22:50:42,633 SpectraRegion INFO: DE data for control: [0, 15, 6, 3, 1, 7, 5, 4]
2020-09-30 22:50:42,634 SpectraRegion INFO: Running [2] against [0, 15, 6, 3, 1, 7, 5, 4]
2020-09-30 22:50:42,634 SpectraRegion INFO: DE result key: ((2,), (0, 1, 3, 4, 5, 6, 7, 15))
2020-09-30 22:50:45,730 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 2408)
2020-09-30 22:50:45,733 SpectraRegion INFO: DE Sample DataFrame ready. Shape (2408, 3)
2020-09-30 22:50:45,738 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:50:46,902 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((2,), (0, 1, 3, 4, 5, 6, 7, 15))
2020-09-30 22:50:46,903 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:50:56,000 SpectraRegion INFO: DE-test (rank) finished. Results available: ((2,), (0, 1, 3, 4, 5, 6, 7, 15))
2020-09-30 22:50:56,003 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 6, 7, 15)) with (17900, 7) results
2020-09-30 22:50:56,004 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:50:56,007 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 6, 7, 15)) with (970, 7) results (filtered)
2020-09-30 22:50:56,065 SpectraRegion INFO: Created matrices with shape (79, 17900) and (2329, 17900) (target, bg)
2020-09-30 22:50:59,148 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 6, 7, 15)) with (17900, 7) results
2020-09-30 22:50:59,149 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:50:59,152 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 6, 7, 15)) with (970, 7) results (filtered)
2020-09-30 22:50:59,212 SpectraRegion INFO: Created matrices with shape (79, 17900) and (2329, 17900) (target, bg)
2020-09-30 22:51:02,221 SpectraRegion INFO: DE data for case: [6]
2020-09-30 22:51:02,221 SpectraRegion INFO: DE data for control: [0, 15, 2, 3, 1, 7, 5, 4]
2020-09-30 22:51:02,222 SpectraRegion INFO: Running [6] against [0, 15, 2, 3, 1, 7, 5, 4]
2020-09-30 22:51:02,222 SpectraRegion INFO: DE result key: ((6,), (0, 1, 2, 3, 4, 5, 7, 15))
2020-09-30 22:51:04,916 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 2408)
2020-09-30 22:51:04,920 SpectraRegion INFO: DE Sample DataFrame ready. Shape (2408, 3)
2020-09-30 22:51:04,924 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:51:05,850 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((6,), (0, 1, 2, 3, 4, 5, 7, 15))
2020-09-30 22:51:05,851 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:51:15,062 SpectraRegion INFO: DE-test (rank) finished. Results available: ((6,), (0, 1, 2, 3, 4, 5, 7, 15))
2020-09-30 22:51:15,066 SpectraRegion INFO: DE result for case ((6,), (0, 1, 2, 3, 4, 5, 7, 15)) with (17900, 7) results
2020-09-30 22:51:15,067 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:51:15,070 SpectraRegion INFO: DE result for case ((6,), (0, 1, 2, 3, 4, 5, 7, 15)) with (1032, 7) results (filtered)
2020-09-30 22:51:15,128 SpectraRegion INFO: Created matrices with shape (86, 17900) and (2322, 17900) (target, bg)
2020-09-30 22:51:18,011 SpectraRegion INFO: DE result for case ((6,), (0, 1, 2, 3, 4, 5, 7, 15)) with (17900, 7) results
2020-09-30 22:51:18,011 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:51:18,014 SpectraRegion INFO: DE result for case ((6,), (0, 1, 2, 3, 4, 5, 7, 15)) with (1032, 7) results (filtered)
2020-09-30 22:51:18,142 SpectraRegion INFO: Created matrices with shape (86, 17900) and (2322, 17900) (target, bg)
2020-09-30 22:51:20,886 SpectraRegion INFO: DE data for case: [3]
2020-09-30 22:51:20,887 SpectraRegion INFO: DE data for control: [0, 15, 2, 6, 1, 7, 5, 4]
2020-09-30 22:51:20,888 SpectraRegion INFO: Running [3] against [0, 15, 2, 6, 1, 7, 5, 4]
2020-09-30 22:51:20,888 SpectraRegion INFO: DE result key: ((3,), (0, 1, 2, 4, 5, 6, 7, 15))
2020-09-30 22:51:23,606 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 2408)
2020-09-30 22:51:23,609 SpectraRegion INFO: DE Sample DataFrame ready. Shape (2408, 3)
2020-09-30 22:51:23,614 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:51:24,558 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((3,), (0, 1, 2, 4, 5, 6, 7, 15))
2020-09-30 22:51:24,559 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:51:34,006 SpectraRegion INFO: DE-test (rank) finished. Results available: ((3,), (0, 1, 2, 4, 5, 6, 7, 15))
2020-09-30 22:51:34,010 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 6, 7, 15)) with (17900, 7) results
2020-09-30 22:51:34,010 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:51:34,013 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 6, 7, 15)) with (977, 7) results (filtered)
2020-09-30 22:51:34,072 SpectraRegion INFO: Created matrices with shape (99, 17900) and (2309, 17900) (target, bg)
2020-09-30 22:51:37,148 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 6, 7, 15)) with (17900, 7) results
2020-09-30 22:51:37,149 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:51:37,151 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 6, 7, 15)) with (977, 7) results (filtered)
2020-09-30 22:51:37,211 SpectraRegion INFO: Created matrices with shape (99, 17900) and (2309, 17900) (target, bg)
2020-09-30 22:51:40,206 SpectraRegion INFO: DE data for case: [1]
2020-09-30 22:51:40,207 SpectraRegion INFO: DE data for control: [0, 15, 2, 6, 3, 7, 5, 4]
2020-09-30 22:51:40,208 SpectraRegion INFO: Running [1] against [0, 15, 2, 6, 3, 7, 5, 4]
2020-09-30 22:51:40,208 SpectraRegion INFO: DE result key: ((1,), (0, 2, 3, 4, 5, 6, 7, 15))
2020-09-30 22:51:43,075 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 2408)
2020-09-30 22:51:43,079 SpectraRegion INFO: DE Sample DataFrame ready. Shape (2408, 3)
2020-09-30 22:51:43,084 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:51:44,053 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((1,), (0, 2, 3, 4, 5, 6, 7, 15))
2020-09-30 22:51:44,054 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:51:53,448 SpectraRegion INFO: DE-test (rank) finished. Results available: ((1,), (0, 2, 3, 4, 5, 6, 7, 15))
2020-09-30 22:51:53,452 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 6, 7, 15)) with (17900, 7) results
2020-09-30 22:51:53,453 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:51:53,456 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 6, 7, 15)) with (1944, 7) results (filtered)
2020-09-30 22:51:53,514 SpectraRegion INFO: Created matrices with shape (61, 17900) and (2347, 17900) (target, bg)
2020-09-30 22:51:59,860 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 6, 7, 15)) with (17900, 7) results
2020-09-30 22:51:59,861 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:51:59,863 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 6, 7, 15)) with (1944, 7) results (filtered)
2020-09-30 22:51:59,924 SpectraRegion INFO: Created matrices with shape (61, 17900) and (2347, 17900) (target, bg)
2020-09-30 22:52:06,294 SpectraRegion INFO: DE data for case: [7]
2020-09-30 22:52:06,294 SpectraRegion INFO: DE data for control: [0, 15, 2, 6, 3, 1, 5, 4]
2020-09-30 22:52:06,295 SpectraRegion INFO: Running [7] against [0, 15, 2, 6, 3, 1, 5, 4]
2020-09-30 22:52:06,295 SpectraRegion INFO: DE result key: ((7,), (0, 1, 2, 3, 4, 5, 6, 15))
2020-09-30 22:52:09,056 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 2408)
2020-09-30 22:52:09,059 SpectraRegion INFO: DE Sample DataFrame ready. Shape (2408, 3)
2020-09-30 22:52:09,063 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:52:10,019 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((7,), (0, 1, 2, 3, 4, 5, 6, 15))
2020-09-30 22:52:10,020 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:52:19,420 SpectraRegion INFO: DE-test (rank) finished. Results available: ((7,), (0, 1, 2, 3, 4, 5, 6, 15))
2020-09-30 22:52:19,424 SpectraRegion INFO: DE result for case ((7,), (0, 1, 2, 3, 4, 5, 6, 15)) with (17900, 7) results
2020-09-30 22:52:19,425 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:52:19,427 SpectraRegion INFO: DE result for case ((7,), (0, 1, 2, 3, 4, 5, 6, 15)) with (1882, 7) results (filtered)
2020-09-30 22:52:19,491 SpectraRegion INFO: Created matrices with shape (39, 17900) and (2369, 17900) (target, bg)
2020-09-30 22:52:24,519 SpectraRegion INFO: DE result for case ((7,), (0, 1, 2, 3, 4, 5, 6, 15)) with (17900, 7) results
2020-09-30 22:52:24,520 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:52:24,523 SpectraRegion INFO: DE result for case ((7,), (0, 1, 2, 3, 4, 5, 6, 15)) with (1888, 7) results (filtered)
2020-09-30 22:52:24,583 SpectraRegion INFO: Created matrices with shape (39, 17900) and (2369, 17900) (target, bg)
2020-09-30 22:52:29,611 SpectraRegion INFO: DE data for case: [5]
2020-09-30 22:52:29,612 SpectraRegion INFO: DE data for control: [0, 15, 2, 6, 3, 1, 7, 4]
2020-09-30 22:52:29,612 SpectraRegion INFO: Running [5] against [0, 15, 2, 6, 3, 1, 7, 4]
2020-09-30 22:52:29,613 SpectraRegion INFO: DE result key: ((5,), (0, 1, 2, 3, 4, 6, 7, 15))
2020-09-30 22:52:32,508 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 2408)
2020-09-30 22:52:32,511 SpectraRegion INFO: DE Sample DataFrame ready. Shape (2408, 3)
2020-09-30 22:52:32,516 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:52:33,513 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((5,), (0, 1, 2, 3, 4, 6, 7, 15))
2020-09-30 22:52:33,514 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:52:43,050 SpectraRegion INFO: DE-test (rank) finished. Results available: ((5,), (0, 1, 2, 3, 4, 6, 7, 15))
2020-09-30 22:52:43,054 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 6, 7, 15)) with (17900, 7) results
2020-09-30 22:52:43,055 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:52:43,058 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 6, 7, 15)) with (1840, 7) results (filtered)
2020-09-30 22:52:43,122 SpectraRegion INFO: Created matrices with shape (19, 17900) and (2389, 17900) (target, bg)
2020-09-30 22:52:47,526 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 6, 7, 15)) with (17900, 7) results
2020-09-30 22:52:47,527 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:52:47,531 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 6, 7, 15)) with (1874, 7) results (filtered)
2020-09-30 22:52:47,590 SpectraRegion INFO: Created matrices with shape (19, 17900) and (2389, 17900) (target, bg)
2020-09-30 22:52:51,668 SpectraRegion INFO: DE data for case: [4]
2020-09-30 22:52:51,669 SpectraRegion INFO: DE data for control: [0, 15, 2, 6, 3, 1, 7, 5]
2020-09-30 22:52:51,670 SpectraRegion INFO: Running [4] against [0, 15, 2, 6, 3, 1, 7, 5]
2020-09-30 22:52:51,670 SpectraRegion INFO: DE result key: ((4,), (0, 1, 2, 3, 5, 6, 7, 15))
2020-09-30 22:52:54,271 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 2408)
2020-09-30 22:52:54,274 SpectraRegion INFO: DE Sample DataFrame ready. Shape (2408, 3)
2020-09-30 22:52:54,279 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:52:55,242 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((4,), (0, 1, 2, 3, 5, 6, 7, 15))
2020-09-30 22:52:55,243 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:53:04,497 SpectraRegion INFO: DE-test (rank) finished. Results available: ((4,), (0, 1, 2, 3, 5, 6, 7, 15))
2020-09-30 22:53:04,501 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 6, 7, 15)) with (17900, 7) results
2020-09-30 22:53:04,502 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:53:04,505 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 6, 7, 15)) with (946, 7) results (filtered)
2020-09-30 22:53:04,568 SpectraRegion INFO: Created matrices with shape (11, 17900) and (2397, 17900) (target, bg)
2020-09-30 22:53:07,265 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 6, 7, 15)) with (17900, 7) results
2020-09-30 22:53:07,266 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:53:07,269 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 6, 7, 15)) with (1065, 7) results (filtered)
2020-09-30 22:53:07,327 SpectraRegion INFO: Created matrices with shape (11, 17900) and (2397, 17900) (target, bg)
slided_0.mass_dabest(pw.protein2mass.get("Tmsb4x"))
slided_1.mass_dabest(pw.protein2mass.get("Tmsb4x"))
slided_4, slided4_mgenes, slided4_mgenes_bg = process_imzeregion(imze=imze, regionID= 4, basename="slideD")
slided_5, slided5_mgenes, slided5_mgenes_bg = process_imzeregion(imze=imze, regionID= 5, basename="slideD")
Fetching region range
Fetching region shape
Found region 4 with shape (49, 56, 17900)
Fetching region spectra
2% (70 of 2744) | | Elapsed Time: 0:00:00 ETA: 0:00:04
Processing regionID 4 for basename slideD
100% (2744 of 2744) |####################| Elapsed Time: 0:00:04 Time: 0:00:04
100% (2744 of 2744) |####################| Elapsed Time: 0:00:00 Time: 0:00:00
Started Log Value: 0.22751572877168655
100% (49 of 49) |########################| Elapsed Time: 0:00:00 Time: 0:00:00
Got 2744 median-enabled pixels
5-Number stats for medians: (2744, 2744, 0.29120835853070265, 0.5888907621230302, 0.660637862228705, 0.7270544871043271, 0.9875144181541924)
Started Log Value: 0.3518995396792889
Collecting fold changes
100% (49 of 49) |########################| Elapsed Time: 0:00:22 Time: 0:00:22
Got a total of 49117600 fold changes
Median elements [24558800]
Median elements
Global Median 0.64784
2020-09-30 22:53:40,667 SpectraRegion INFO: dimensions inputarray: 17900
2020-09-30 22:53:40,667 SpectraRegion INFO: Creating C++ obj
2020-09-30 22:53:40,668 SpectraRegion INFO: 17900 (49, 56, 17900)
2020-09-30 22:53:40,668 SpectraRegion INFO: dimensions 17900
2020-09-30 22:53:40,669 SpectraRegion INFO: input dimensions (49, 56, 17900)
2020-09-30 22:53:40,669 SpectraRegion INFO: Switching to dot mode
2020-09-30 22:53:40,713 SpectraRegion INFO: Starting calc similarity c++
2020-09-30 22:54:36,001 SpectraRegion INFO: outclust dimensions (2744, 2744)
2020-09-30 22:54:36,002 SpectraRegion INFO: Calculating spectra similarity
2020-09-30 22:54:36,069 SpectraRegion INFO: Calculating spectra similarity done
2020-09-30 22:54:36,075 SpectraRegion INFO: Calculating dist pixel map
2020-09-30 22:55:15,270 SpectraRegion INFO: Calculating dist pixel map done
2020-09-30 22:55:15,293 SpectraRegion INFO: Calculating clusters
2020-09-30 22:55:15,505 SpectraRegion INFO: Calculating clusters done
2020-09-30 22:55:15,521 SpectraRegion INFO: Calculating clusters saved
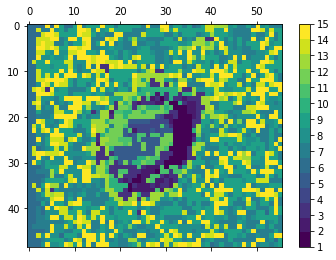
2020-09-30 22:55:15,622 SpectraRegion INFO: Assigning clusters to background: {6, 7, 8, 9, 14, 15}
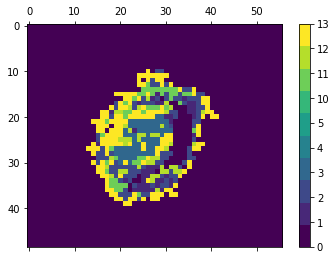
2020-09-30 22:55:15,720 SpectraRegion INFO: DE data for case: [13]
2020-09-30 22:55:15,721 SpectraRegion INFO: DE data for control: [3, 10, 12, 2, 11, 1, 5, 4]
2020-09-30 22:55:15,721 SpectraRegion INFO: Running [13] against [3, 10, 12, 2, 11, 1, 5, 4]
2020-09-30 22:55:15,722 SpectraRegion INFO: DE result key: ((13,), (1, 2, 3, 4, 5, 10, 11, 12))
2020-09-30 22:55:15,938 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 526)
2020-09-30 22:55:15,941 SpectraRegion INFO: DE Sample DataFrame ready. Shape (526, 3)
2020-09-30 22:55:15,945 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:55:16,106 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((13,), (1, 2, 3, 4, 5, 10, 11, 12))
2020-09-30 22:55:16,106 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:55:20,246 SpectraRegion INFO: DE-test (rank) finished. Results available: ((13,), (1, 2, 3, 4, 5, 10, 11, 12))
2020-09-30 22:55:20,249 SpectraRegion INFO: DE result for case ((13,), (1, 2, 3, 4, 5, 10, 11, 12)) with (17900, 7) results
2020-09-30 22:55:20,249 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:55:20,252 SpectraRegion INFO: DE result for case ((13,), (1, 2, 3, 4, 5, 10, 11, 12)) with (741, 7) results (filtered)
2020-09-30 22:55:20,273 SpectraRegion INFO: Created matrices with shape (101, 17900) and (425, 17900) (target, bg)
2020-09-30 22:55:21,894 SpectraRegion INFO: DE result for case ((13,), (1, 2, 3, 4, 5, 10, 11, 12)) with (17900, 7) results
2020-09-30 22:55:21,895 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:55:21,898 SpectraRegion INFO: DE result for case ((13,), (1, 2, 3, 4, 5, 10, 11, 12)) with (740, 7) results (filtered)
2020-09-30 22:55:21,918 SpectraRegion INFO: Created matrices with shape (101, 17900) and (425, 17900) (target, bg)
2020-09-30 22:55:23,531 SpectraRegion INFO: DE data for case: [3]
2020-09-30 22:55:23,532 SpectraRegion INFO: DE data for control: [13, 10, 12, 2, 11, 1, 5, 4]
2020-09-30 22:55:23,532 SpectraRegion INFO: Running [3] against [13, 10, 12, 2, 11, 1, 5, 4]
2020-09-30 22:55:23,533 SpectraRegion INFO: DE result key: ((3,), (1, 2, 4, 5, 10, 11, 12, 13))
2020-09-30 22:55:23,738 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 526)
2020-09-30 22:55:23,741 SpectraRegion INFO: DE Sample DataFrame ready. Shape (526, 3)
2020-09-30 22:55:23,744 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:55:23,910 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((3,), (1, 2, 4, 5, 10, 11, 12, 13))
2020-09-30 22:55:23,911 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:55:28,027 SpectraRegion INFO: DE-test (rank) finished. Results available: ((3,), (1, 2, 4, 5, 10, 11, 12, 13))
2020-09-30 22:55:28,029 SpectraRegion INFO: DE result for case ((3,), (1, 2, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 22:55:28,030 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:55:28,033 SpectraRegion INFO: DE result for case ((3,), (1, 2, 4, 5, 10, 11, 12, 13)) with (125, 7) results (filtered)
2020-09-30 22:55:28,054 SpectraRegion INFO: Created matrices with shape (102, 17900) and (424, 17900) (target, bg)
2020-09-30 22:55:28,178 SpectraRegion INFO: DE result for case ((3,), (1, 2, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 22:55:28,179 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:55:28,182 SpectraRegion INFO: DE result for case ((3,), (1, 2, 4, 5, 10, 11, 12, 13)) with (79, 7) results (filtered)
2020-09-30 22:55:28,203 SpectraRegion INFO: Created matrices with shape (102, 17900) and (424, 17900) (target, bg)
2020-09-30 22:55:28,293 SpectraRegion INFO: DE data for case: [10]
2020-09-30 22:55:28,294 SpectraRegion INFO: DE data for control: [13, 3, 12, 2, 11, 1, 5, 4]
2020-09-30 22:55:28,295 SpectraRegion INFO: Running [10] against [13, 3, 12, 2, 11, 1, 5, 4]
2020-09-30 22:55:28,295 SpectraRegion INFO: DE result key: ((10,), (1, 2, 3, 4, 5, 11, 12, 13))
2020-09-30 22:55:28,506 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 526)
2020-09-30 22:55:28,509 SpectraRegion INFO: DE Sample DataFrame ready. Shape (526, 3)
2020-09-30 22:55:28,512 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:55:28,679 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((10,), (1, 2, 3, 4, 5, 11, 12, 13))
2020-09-30 22:55:28,679 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:55:32,791 SpectraRegion INFO: DE-test (rank) finished. Results available: ((10,), (1, 2, 3, 4, 5, 11, 12, 13))
2020-09-30 22:55:32,793 SpectraRegion INFO: DE result for case ((10,), (1, 2, 3, 4, 5, 11, 12, 13)) with (17900, 7) results
2020-09-30 22:55:32,794 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:55:32,797 SpectraRegion INFO: DE result for case ((10,), (1, 2, 3, 4, 5, 11, 12, 13)) with (267, 7) results (filtered)
2020-09-30 22:55:32,814 SpectraRegion INFO: Created matrices with shape (49, 17900) and (477, 17900) (target, bg)
2020-09-30 22:55:33,482 SpectraRegion INFO: DE result for case ((10,), (1, 2, 3, 4, 5, 11, 12, 13)) with (17900, 7) results
2020-09-30 22:55:33,482 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:55:33,485 SpectraRegion INFO: DE result for case ((10,), (1, 2, 3, 4, 5, 11, 12, 13)) with (203, 7) results (filtered)
2020-09-30 22:55:33,502 SpectraRegion INFO: Created matrices with shape (49, 17900) and (477, 17900) (target, bg)
2020-09-30 22:55:34,092 SpectraRegion INFO: DE data for case: [12]
2020-09-30 22:55:34,093 SpectraRegion INFO: DE data for control: [13, 3, 10, 2, 11, 1, 5, 4]
2020-09-30 22:55:34,093 SpectraRegion INFO: Running [12] against [13, 3, 10, 2, 11, 1, 5, 4]
2020-09-30 22:55:34,094 SpectraRegion INFO: DE result key: ((12,), (1, 2, 3, 4, 5, 10, 11, 13))
2020-09-30 22:55:34,304 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 526)
2020-09-30 22:55:34,306 SpectraRegion INFO: DE Sample DataFrame ready. Shape (526, 3)
2020-09-30 22:55:34,310 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:55:34,481 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((12,), (1, 2, 3, 4, 5, 10, 11, 13))
2020-09-30 22:55:34,482 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:55:38,592 SpectraRegion INFO: DE-test (rank) finished. Results available: ((12,), (1, 2, 3, 4, 5, 10, 11, 13))
2020-09-30 22:55:38,594 SpectraRegion INFO: DE result for case ((12,), (1, 2, 3, 4, 5, 10, 11, 13)) with (17900, 7) results
2020-09-30 22:55:38,594 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:55:38,598 SpectraRegion INFO: DE result for case ((12,), (1, 2, 3, 4, 5, 10, 11, 13)) with (1249, 7) results (filtered)
2020-09-30 22:55:38,616 SpectraRegion INFO: Created matrices with shape (61, 17900) and (465, 17900) (target, bg)
2020-09-30 22:55:41,678 SpectraRegion INFO: DE result for case ((12,), (1, 2, 3, 4, 5, 10, 11, 13)) with (17900, 7) results
2020-09-30 22:55:41,679 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:55:41,682 SpectraRegion INFO: DE result for case ((12,), (1, 2, 3, 4, 5, 10, 11, 13)) with (1243, 7) results (filtered)
2020-09-30 22:55:41,698 SpectraRegion INFO: Created matrices with shape (61, 17900) and (465, 17900) (target, bg)
2020-09-30 22:55:44,749 SpectraRegion INFO: DE data for case: [2]
2020-09-30 22:55:44,749 SpectraRegion INFO: DE data for control: [13, 3, 10, 12, 11, 1, 5, 4]
2020-09-30 22:55:44,750 SpectraRegion INFO: Running [2] against [13, 3, 10, 12, 11, 1, 5, 4]
2020-09-30 22:55:44,750 SpectraRegion INFO: DE result key: ((2,), (1, 3, 4, 5, 10, 11, 12, 13))
2020-09-30 22:55:44,961 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 526)
2020-09-30 22:55:44,964 SpectraRegion INFO: DE Sample DataFrame ready. Shape (526, 3)
2020-09-30 22:55:44,967 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:55:45,135 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((2,), (1, 3, 4, 5, 10, 11, 12, 13))
2020-09-30 22:55:45,136 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:55:49,287 SpectraRegion INFO: DE-test (rank) finished. Results available: ((2,), (1, 3, 4, 5, 10, 11, 12, 13))
2020-09-30 22:55:49,289 SpectraRegion INFO: DE result for case ((2,), (1, 3, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 22:55:49,290 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:55:49,293 SpectraRegion INFO: DE result for case ((2,), (1, 3, 4, 5, 10, 11, 12, 13)) with (659, 7) results (filtered)
2020-09-30 22:55:49,310 SpectraRegion INFO: Created matrices with shape (45, 17900) and (481, 17900) (target, bg)
2020-09-30 22:55:51,218 SpectraRegion INFO: DE result for case ((2,), (1, 3, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 22:55:51,219 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:55:51,222 SpectraRegion INFO: DE result for case ((2,), (1, 3, 4, 5, 10, 11, 12, 13)) with (601, 7) results (filtered)
2020-09-30 22:55:51,239 SpectraRegion INFO: Created matrices with shape (45, 17900) and (481, 17900) (target, bg)
2020-09-30 22:55:53,000 SpectraRegion INFO: DE data for case: [11]
2020-09-30 22:55:53,001 SpectraRegion INFO: DE data for control: [13, 3, 10, 12, 2, 1, 5, 4]
2020-09-30 22:55:53,002 SpectraRegion INFO: Running [11] against [13, 3, 10, 12, 2, 1, 5, 4]
2020-09-30 22:55:53,002 SpectraRegion INFO: DE result key: ((11,), (1, 2, 3, 4, 5, 10, 12, 13))
2020-09-30 22:55:53,213 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 526)
2020-09-30 22:55:53,216 SpectraRegion INFO: DE Sample DataFrame ready. Shape (526, 3)
2020-09-30 22:55:53,220 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:55:53,390 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((11,), (1, 2, 3, 4, 5, 10, 12, 13))
2020-09-30 22:55:53,391 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:55:57,529 SpectraRegion INFO: DE-test (rank) finished. Results available: ((11,), (1, 2, 3, 4, 5, 10, 12, 13))
2020-09-30 22:55:57,531 SpectraRegion INFO: DE result for case ((11,), (1, 2, 3, 4, 5, 10, 12, 13)) with (17900, 7) results
2020-09-30 22:55:57,532 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:55:57,534 SpectraRegion INFO: DE result for case ((11,), (1, 2, 3, 4, 5, 10, 12, 13)) with (122, 7) results (filtered)
2020-09-30 22:55:57,551 SpectraRegion INFO: Created matrices with shape (45, 17900) and (481, 17900) (target, bg)
2020-09-30 22:55:58,083 SpectraRegion INFO: DE result for case ((11,), (1, 2, 3, 4, 5, 10, 12, 13)) with (17900, 7) results
2020-09-30 22:55:58,084 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:55:58,086 SpectraRegion INFO: DE result for case ((11,), (1, 2, 3, 4, 5, 10, 12, 13)) with (100, 7) results (filtered)
2020-09-30 22:55:58,104 SpectraRegion INFO: Created matrices with shape (45, 17900) and (481, 17900) (target, bg)
2020-09-30 22:55:58,499 SpectraRegion INFO: DE data for case: [1]
2020-09-30 22:55:58,500 SpectraRegion INFO: DE data for control: [13, 3, 10, 12, 2, 11, 5, 4]
2020-09-30 22:55:58,500 SpectraRegion INFO: Running [1] against [13, 3, 10, 12, 2, 11, 5, 4]
2020-09-30 22:55:58,501 SpectraRegion INFO: DE result key: ((1,), (2, 3, 4, 5, 10, 11, 12, 13))
2020-09-30 22:55:58,715 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 526)
2020-09-30 22:55:58,718 SpectraRegion INFO: DE Sample DataFrame ready. Shape (526, 3)
2020-09-30 22:55:58,721 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:55:58,893 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((1,), (2, 3, 4, 5, 10, 11, 12, 13))
2020-09-30 22:55:58,894 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:56:03,025 SpectraRegion INFO: DE-test (rank) finished. Results available: ((1,), (2, 3, 4, 5, 10, 11, 12, 13))
2020-09-30 22:56:03,028 SpectraRegion INFO: DE result for case ((1,), (2, 3, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 22:56:03,028 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:56:03,032 SpectraRegion INFO: DE result for case ((1,), (2, 3, 4, 5, 10, 11, 12, 13)) with (525, 7) results (filtered)
2020-09-30 22:56:03,049 SpectraRegion INFO: Created matrices with shape (57, 17900) and (469, 17900) (target, bg)
2020-09-30 22:56:04,155 SpectraRegion INFO: DE result for case ((1,), (2, 3, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 22:56:04,156 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:56:04,159 SpectraRegion INFO: DE result for case ((1,), (2, 3, 4, 5, 10, 11, 12, 13)) with (471, 7) results (filtered)
2020-09-30 22:56:04,177 SpectraRegion INFO: Created matrices with shape (57, 17900) and (469, 17900) (target, bg)
2020-09-30 22:56:05,192 SpectraRegion INFO: DE data for case: [5]
2020-09-30 22:56:05,192 SpectraRegion INFO: DE data for control: [13, 3, 10, 12, 2, 11, 1, 4]
2020-09-30 22:56:05,193 SpectraRegion INFO: Running [5] against [13, 3, 10, 12, 2, 11, 1, 4]
2020-09-30 22:56:05,193 SpectraRegion INFO: DE result key: ((5,), (1, 2, 3, 4, 10, 11, 12, 13))
2020-09-30 22:56:05,408 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 526)
2020-09-30 22:56:05,411 SpectraRegion INFO: DE Sample DataFrame ready. Shape (526, 3)
2020-09-30 22:56:05,414 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:56:05,588 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((5,), (1, 2, 3, 4, 10, 11, 12, 13))
2020-09-30 22:56:05,588 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:56:09,716 SpectraRegion INFO: DE-test (rank) finished. Results available: ((5,), (1, 2, 3, 4, 10, 11, 12, 13))
2020-09-30 22:56:09,718 SpectraRegion INFO: DE result for case ((5,), (1, 2, 3, 4, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 22:56:09,719 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:56:09,722 SpectraRegion INFO: DE result for case ((5,), (1, 2, 3, 4, 10, 11, 12, 13)) with (544, 7) results (filtered)
2020-09-30 22:56:09,739 SpectraRegion INFO: Created matrices with shape (48, 17900) and (478, 17900) (target, bg)
2020-09-30 22:56:11,287 SpectraRegion INFO: DE result for case ((5,), (1, 2, 3, 4, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 22:56:11,288 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:56:11,291 SpectraRegion INFO: DE result for case ((5,), (1, 2, 3, 4, 10, 11, 12, 13)) with (537, 7) results (filtered)
2020-09-30 22:56:11,306 SpectraRegion INFO: Created matrices with shape (48, 17900) and (478, 17900) (target, bg)
2020-09-30 22:56:12,824 SpectraRegion INFO: DE data for case: [4]
2020-09-30 22:56:12,824 SpectraRegion INFO: DE data for control: [13, 3, 10, 12, 2, 11, 1, 5]
2020-09-30 22:56:12,825 SpectraRegion INFO: Running [4] against [13, 3, 10, 12, 2, 11, 1, 5]
2020-09-30 22:56:12,825 SpectraRegion INFO: DE result key: ((4,), (1, 2, 3, 5, 10, 11, 12, 13))
2020-09-30 22:56:13,040 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 526)
2020-09-30 22:56:13,043 SpectraRegion INFO: DE Sample DataFrame ready. Shape (526, 3)
2020-09-30 22:56:13,046 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:56:13,219 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((4,), (1, 2, 3, 5, 10, 11, 12, 13))
2020-09-30 22:56:13,220 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:56:17,378 SpectraRegion INFO: DE-test (rank) finished. Results available: ((4,), (1, 2, 3, 5, 10, 11, 12, 13))
2020-09-30 22:56:17,380 SpectraRegion INFO: DE result for case ((4,), (1, 2, 3, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 22:56:17,381 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:56:17,384 SpectraRegion INFO: DE result for case ((4,), (1, 2, 3, 5, 10, 11, 12, 13)) with (1615, 7) results (filtered)
2020-09-30 22:56:17,402 SpectraRegion INFO: Created matrices with shape (18, 17900) and (508, 17900) (target, bg)
2020-09-30 22:56:21,995 SpectraRegion INFO: DE result for case ((4,), (1, 2, 3, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 22:56:21,995 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:56:21,998 SpectraRegion INFO: DE result for case ((4,), (1, 2, 3, 5, 10, 11, 12, 13)) with (1443, 7) results (filtered)
2020-09-30 22:56:22,014 SpectraRegion INFO: Created matrices with shape (18, 17900) and (508, 17900) (target, bg)
2020-09-30 22:56:26,368 SpectraRegion INFO: DE data for case: [0]
2020-09-30 22:56:26,369 SpectraRegion INFO: DE data for control: [13, 3, 10, 12, 2, 11, 1, 5, 4]
2020-09-30 22:56:26,370 SpectraRegion INFO: Running [0] against [13, 3, 10, 12, 2, 11, 1, 5, 4]
2020-09-30 22:56:26,370 SpectraRegion INFO: DE result key: ((0,), (1, 2, 3, 4, 5, 10, 11, 12, 13))
2020-09-30 22:56:29,814 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 2744)
2020-09-30 22:56:29,817 SpectraRegion INFO: DE Sample DataFrame ready. Shape (2744, 3)
2020-09-30 22:56:29,821 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:56:31,253 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((0,), (1, 2, 3, 4, 5, 10, 11, 12, 13))
2020-09-30 22:56:31,254 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:56:41,518 SpectraRegion INFO: DE-test (rank) finished. Results available: ((0,), (1, 2, 3, 4, 5, 10, 11, 12, 13))
2020-09-30 22:56:41,522 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 22:56:41,523 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:56:41,526 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 10, 11, 12, 13)) with (1491, 7) results (filtered)
2020-09-30 22:56:41,595 SpectraRegion INFO: Created matrices with shape (2218, 17900) and (526, 17900) (target, bg)
2020-09-30 22:56:46,150 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 22:56:46,150 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:56:46,154 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 10, 11, 12, 13)) with (1491, 7) results (filtered)
2020-09-30 22:56:46,222 SpectraRegion INFO: Created matrices with shape (2218, 17900) and (526, 17900) (target, bg)
2020-09-30 22:56:50,742 SpectraRegion INFO: DE data for case: [13]
2020-09-30 22:56:50,744 SpectraRegion INFO: DE data for control: [0, 3, 10, 12, 2, 11, 1, 5, 4]
2020-09-30 22:56:50,745 SpectraRegion INFO: Running [13] against [0, 3, 10, 12, 2, 11, 1, 5, 4]
2020-09-30 22:56:50,746 SpectraRegion INFO: DE result key: ((13,), (0, 1, 2, 3, 4, 5, 10, 11, 12))
2020-09-30 22:56:54,236 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 2744)
2020-09-30 22:56:54,239 SpectraRegion INFO: DE Sample DataFrame ready. Shape (2744, 3)
2020-09-30 22:56:54,244 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:56:55,454 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((13,), (0, 1, 2, 3, 4, 5, 10, 11, 12))
2020-09-30 22:56:55,455 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:57:05,806 SpectraRegion INFO: DE-test (rank) finished. Results available: ((13,), (0, 1, 2, 3, 4, 5, 10, 11, 12))
2020-09-30 22:57:05,809 SpectraRegion INFO: DE result for case ((13,), (0, 1, 2, 3, 4, 5, 10, 11, 12)) with (17900, 7) results
2020-09-30 22:57:05,810 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:57:05,813 SpectraRegion INFO: DE result for case ((13,), (0, 1, 2, 3, 4, 5, 10, 11, 12)) with (89, 7) results (filtered)
2020-09-30 22:57:05,888 SpectraRegion INFO: Created matrices with shape (101, 17900) and (2643, 17900) (target, bg)
2020-09-30 22:57:06,147 SpectraRegion INFO: DE result for case ((13,), (0, 1, 2, 3, 4, 5, 10, 11, 12)) with (17900, 7) results
2020-09-30 22:57:06,148 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:57:06,151 SpectraRegion INFO: DE result for case ((13,), (0, 1, 2, 3, 4, 5, 10, 11, 12)) with (89, 7) results (filtered)
2020-09-30 22:57:06,220 SpectraRegion INFO: Created matrices with shape (101, 17900) and (2643, 17900) (target, bg)
2020-09-30 22:57:06,451 SpectraRegion INFO: DE data for case: [3]
2020-09-30 22:57:06,452 SpectraRegion INFO: DE data for control: [0, 13, 10, 12, 2, 11, 1, 5, 4]
2020-09-30 22:57:06,453 SpectraRegion INFO: Running [3] against [0, 13, 10, 12, 2, 11, 1, 5, 4]
2020-09-30 22:57:06,453 SpectraRegion INFO: DE result key: ((3,), (0, 1, 2, 4, 5, 10, 11, 12, 13))
2020-09-30 22:57:10,415 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 2744)
2020-09-30 22:57:10,418 SpectraRegion INFO: DE Sample DataFrame ready. Shape (2744, 3)
2020-09-30 22:57:10,423 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:57:11,718 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((3,), (0, 1, 2, 4, 5, 10, 11, 12, 13))
2020-09-30 22:57:11,718 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:57:22,495 SpectraRegion INFO: DE-test (rank) finished. Results available: ((3,), (0, 1, 2, 4, 5, 10, 11, 12, 13))
2020-09-30 22:57:22,500 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 22:57:22,500 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:57:22,504 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 10, 11, 12, 13)) with (1138, 7) results (filtered)
2020-09-30 22:57:22,576 SpectraRegion INFO: Created matrices with shape (102, 17900) and (2642, 17900) (target, bg)
2020-09-30 22:57:25,925 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 22:57:25,926 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:57:25,929 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 10, 11, 12, 13)) with (1138, 7) results (filtered)
2020-09-30 22:57:25,999 SpectraRegion INFO: Created matrices with shape (102, 17900) and (2642, 17900) (target, bg)
2020-09-30 22:57:29,305 SpectraRegion INFO: DE data for case: [10]
2020-09-30 22:57:29,305 SpectraRegion INFO: DE data for control: [0, 13, 3, 12, 2, 11, 1, 5, 4]
2020-09-30 22:57:29,306 SpectraRegion INFO: Running [10] against [0, 13, 3, 12, 2, 11, 1, 5, 4]
2020-09-30 22:57:29,306 SpectraRegion INFO: DE result key: ((10,), (0, 1, 2, 3, 4, 5, 11, 12, 13))
2020-09-30 22:57:32,863 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 2744)
2020-09-30 22:57:32,866 SpectraRegion INFO: DE Sample DataFrame ready. Shape (2744, 3)
2020-09-30 22:57:32,870 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:57:33,969 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((10,), (0, 1, 2, 3, 4, 5, 11, 12, 13))
2020-09-30 22:57:33,970 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:57:44,332 SpectraRegion INFO: DE-test (rank) finished. Results available: ((10,), (0, 1, 2, 3, 4, 5, 11, 12, 13))
2020-09-30 22:57:44,338 SpectraRegion INFO: DE result for case ((10,), (0, 1, 2, 3, 4, 5, 11, 12, 13)) with (17900, 7) results
2020-09-30 22:57:44,338 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:57:44,342 SpectraRegion INFO: DE result for case ((10,), (0, 1, 2, 3, 4, 5, 11, 12, 13)) with (868, 7) results (filtered)
2020-09-30 22:57:44,414 SpectraRegion INFO: Created matrices with shape (49, 17900) and (2695, 17900) (target, bg)
2020-09-30 22:57:46,757 SpectraRegion INFO: DE result for case ((10,), (0, 1, 2, 3, 4, 5, 11, 12, 13)) with (17900, 7) results
2020-09-30 22:57:46,758 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:57:46,762 SpectraRegion INFO: DE result for case ((10,), (0, 1, 2, 3, 4, 5, 11, 12, 13)) with (868, 7) results (filtered)
2020-09-30 22:57:46,840 SpectraRegion INFO: Created matrices with shape (49, 17900) and (2695, 17900) (target, bg)
2020-09-30 22:57:49,150 SpectraRegion INFO: DE data for case: [12]
2020-09-30 22:57:49,151 SpectraRegion INFO: DE data for control: [0, 13, 3, 10, 2, 11, 1, 5, 4]
2020-09-30 22:57:49,151 SpectraRegion INFO: Running [12] against [0, 13, 3, 10, 2, 11, 1, 5, 4]
2020-09-30 22:57:49,152 SpectraRegion INFO: DE result key: ((12,), (0, 1, 2, 3, 4, 5, 10, 11, 13))
2020-09-30 22:57:53,146 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 2744)
2020-09-30 22:57:53,150 SpectraRegion INFO: DE Sample DataFrame ready. Shape (2744, 3)
2020-09-30 22:57:53,155 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:57:54,389 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((12,), (0, 1, 2, 3, 4, 5, 10, 11, 13))
2020-09-30 22:57:54,390 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:58:04,808 SpectraRegion INFO: DE-test (rank) finished. Results available: ((12,), (0, 1, 2, 3, 4, 5, 10, 11, 13))
2020-09-30 22:58:04,812 SpectraRegion INFO: DE result for case ((12,), (0, 1, 2, 3, 4, 5, 10, 11, 13)) with (17900, 7) results
2020-09-30 22:58:04,812 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:58:04,815 SpectraRegion INFO: DE result for case ((12,), (0, 1, 2, 3, 4, 5, 10, 11, 13)) with (1575, 7) results (filtered)
2020-09-30 22:58:04,886 SpectraRegion INFO: Created matrices with shape (61, 17900) and (2683, 17900) (target, bg)
2020-09-30 22:58:08,856 SpectraRegion INFO: DE result for case ((12,), (0, 1, 2, 3, 4, 5, 10, 11, 13)) with (17900, 7) results
2020-09-30 22:58:08,857 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:58:08,860 SpectraRegion INFO: DE result for case ((12,), (0, 1, 2, 3, 4, 5, 10, 11, 13)) with (1574, 7) results (filtered)
2020-09-30 22:58:08,929 SpectraRegion INFO: Created matrices with shape (61, 17900) and (2683, 17900) (target, bg)
2020-09-30 22:58:12,785 SpectraRegion INFO: DE data for case: [2]
2020-09-30 22:58:12,786 SpectraRegion INFO: DE data for control: [0, 13, 3, 10, 12, 11, 1, 5, 4]
2020-09-30 22:58:12,787 SpectraRegion INFO: Running [2] against [0, 13, 3, 10, 12, 11, 1, 5, 4]
2020-09-30 22:58:12,787 SpectraRegion INFO: DE result key: ((2,), (0, 1, 3, 4, 5, 10, 11, 12, 13))
2020-09-30 22:58:16,534 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 2744)
2020-09-30 22:58:16,537 SpectraRegion INFO: DE Sample DataFrame ready. Shape (2744, 3)
2020-09-30 22:58:16,542 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:58:17,705 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((2,), (0, 1, 3, 4, 5, 10, 11, 12, 13))
2020-09-30 22:58:17,706 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:58:28,168 SpectraRegion INFO: DE-test (rank) finished. Results available: ((2,), (0, 1, 3, 4, 5, 10, 11, 12, 13))
2020-09-30 22:58:28,171 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 22:58:28,172 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:58:28,176 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 10, 11, 12, 13)) with (2184, 7) results (filtered)
2020-09-30 22:58:28,248 SpectraRegion INFO: Created matrices with shape (45, 17900) and (2699, 17900) (target, bg)
2020-09-30 22:58:35,970 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 22:58:35,971 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:58:35,973 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 10, 11, 12, 13)) with (2184, 7) results (filtered)
2020-09-30 22:58:36,039 SpectraRegion INFO: Created matrices with shape (45, 17900) and (2699, 17900) (target, bg)
2020-09-30 22:58:43,729 SpectraRegion INFO: DE data for case: [11]
2020-09-30 22:58:43,729 SpectraRegion INFO: DE data for control: [0, 13, 3, 10, 12, 2, 1, 5, 4]
2020-09-30 22:58:43,730 SpectraRegion INFO: Running [11] against [0, 13, 3, 10, 12, 2, 1, 5, 4]
2020-09-30 22:58:43,730 SpectraRegion INFO: DE result key: ((11,), (0, 1, 2, 3, 4, 5, 10, 12, 13))
2020-09-30 22:58:47,384 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 2744)
2020-09-30 22:58:47,387 SpectraRegion INFO: DE Sample DataFrame ready. Shape (2744, 3)
2020-09-30 22:58:47,392 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:58:48,515 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((11,), (0, 1, 2, 3, 4, 5, 10, 12, 13))
2020-09-30 22:58:48,515 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:58:59,200 SpectraRegion INFO: DE-test (rank) finished. Results available: ((11,), (0, 1, 2, 3, 4, 5, 10, 12, 13))
2020-09-30 22:58:59,204 SpectraRegion INFO: DE result for case ((11,), (0, 1, 2, 3, 4, 5, 10, 12, 13)) with (17900, 7) results
2020-09-30 22:58:59,205 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:58:59,208 SpectraRegion INFO: DE result for case ((11,), (0, 1, 2, 3, 4, 5, 10, 12, 13)) with (1552, 7) results (filtered)
2020-09-30 22:58:59,278 SpectraRegion INFO: Created matrices with shape (45, 17900) and (2699, 17900) (target, bg)
2020-09-30 22:59:03,809 SpectraRegion INFO: DE result for case ((11,), (0, 1, 2, 3, 4, 5, 10, 12, 13)) with (17900, 7) results
2020-09-30 22:59:03,810 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:59:03,812 SpectraRegion INFO: DE result for case ((11,), (0, 1, 2, 3, 4, 5, 10, 12, 13)) with (1554, 7) results (filtered)
2020-09-30 22:59:03,880 SpectraRegion INFO: Created matrices with shape (45, 17900) and (2699, 17900) (target, bg)
2020-09-30 22:59:08,409 SpectraRegion INFO: DE data for case: [1]
2020-09-30 22:59:08,409 SpectraRegion INFO: DE data for control: [0, 13, 3, 10, 12, 2, 11, 5, 4]
2020-09-30 22:59:08,410 SpectraRegion INFO: Running [1] against [0, 13, 3, 10, 12, 2, 11, 5, 4]
2020-09-30 22:59:08,410 SpectraRegion INFO: DE result key: ((1,), (0, 2, 3, 4, 5, 10, 11, 12, 13))
2020-09-30 22:59:11,913 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 2744)
2020-09-30 22:59:11,917 SpectraRegion INFO: DE Sample DataFrame ready. Shape (2744, 3)
2020-09-30 22:59:11,923 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:59:13,055 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((1,), (0, 2, 3, 4, 5, 10, 11, 12, 13))
2020-09-30 22:59:13,056 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:59:23,488 SpectraRegion INFO: DE-test (rank) finished. Results available: ((1,), (0, 2, 3, 4, 5, 10, 11, 12, 13))
2020-09-30 22:59:23,492 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 22:59:23,492 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:59:23,496 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 10, 11, 12, 13)) with (2040, 7) results (filtered)
2020-09-30 22:59:23,568 SpectraRegion INFO: Created matrices with shape (57, 17900) and (2687, 17900) (target, bg)
2020-09-30 22:59:30,593 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 22:59:30,594 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:59:30,597 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 10, 11, 12, 13)) with (2038, 7) results (filtered)
2020-09-30 22:59:30,666 SpectraRegion INFO: Created matrices with shape (57, 17900) and (2687, 17900) (target, bg)
2020-09-30 22:59:37,496 SpectraRegion INFO: DE data for case: [5]
2020-09-30 22:59:37,497 SpectraRegion INFO: DE data for control: [0, 13, 3, 10, 12, 2, 11, 1, 4]
2020-09-30 22:59:37,497 SpectraRegion INFO: Running [5] against [0, 13, 3, 10, 12, 2, 11, 1, 4]
2020-09-30 22:59:37,498 SpectraRegion INFO: DE result key: ((5,), (0, 1, 2, 3, 4, 10, 11, 12, 13))
2020-09-30 22:59:40,899 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 2744)
2020-09-30 22:59:40,902 SpectraRegion INFO: DE Sample DataFrame ready. Shape (2744, 3)
2020-09-30 22:59:40,907 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 22:59:42,048 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((5,), (0, 1, 2, 3, 4, 10, 11, 12, 13))
2020-09-30 22:59:42,049 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 22:59:52,418 SpectraRegion INFO: DE-test (rank) finished. Results available: ((5,), (0, 1, 2, 3, 4, 10, 11, 12, 13))
2020-09-30 22:59:52,421 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 22:59:52,422 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:59:52,425 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 10, 11, 12, 13)) with (1661, 7) results (filtered)
2020-09-30 22:59:52,496 SpectraRegion INFO: Created matrices with shape (48, 17900) and (2696, 17900) (target, bg)
2020-09-30 22:59:57,205 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 22:59:57,205 SpectraRegion INFO: DE result logFC inversed
2020-09-30 22:59:57,210 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 10, 11, 12, 13)) with (1659, 7) results (filtered)
2020-09-30 22:59:57,303 SpectraRegion INFO: Created matrices with shape (48, 17900) and (2696, 17900) (target, bg)
2020-09-30 23:00:01,937 SpectraRegion INFO: DE data for case: [4]
2020-09-30 23:00:01,937 SpectraRegion INFO: DE data for control: [0, 13, 3, 10, 12, 2, 11, 1, 5]
2020-09-30 23:00:01,938 SpectraRegion INFO: Running [4] against [0, 13, 3, 10, 12, 2, 11, 1, 5]
2020-09-30 23:00:01,938 SpectraRegion INFO: DE result key: ((4,), (0, 1, 2, 3, 5, 10, 11, 12, 13))
2020-09-30 23:00:05,739 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 2744)
2020-09-30 23:00:05,743 SpectraRegion INFO: DE Sample DataFrame ready. Shape (2744, 3)
2020-09-30 23:00:05,749 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 23:00:06,947 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((4,), (0, 1, 2, 3, 5, 10, 11, 12, 13))
2020-09-30 23:00:06,948 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 23:00:17,546 SpectraRegion INFO: DE-test (rank) finished. Results available: ((4,), (0, 1, 2, 3, 5, 10, 11, 12, 13))
2020-09-30 23:00:17,550 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 23:00:17,551 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:00:17,554 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 10, 11, 12, 13)) with (2953, 7) results (filtered)
2020-09-30 23:00:17,621 SpectraRegion INFO: Created matrices with shape (18, 17900) and (2726, 17900) (target, bg)
2020-09-30 23:00:27,137 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 23:00:27,138 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:00:27,141 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 10, 11, 12, 13)) with (2920, 7) results (filtered)
2020-09-30 23:00:27,210 SpectraRegion INFO: Created matrices with shape (18, 17900) and (2726, 17900) (target, bg)
Fetching region range
Fetching region shape
Found region 5 with shape (52, 59, 17900)
Fetching region spectra
2% (78 of 3068) | | Elapsed Time: 0:00:00 ETA: 0:00:05
Processing regionID 5 for basename slideD
100% (3068 of 3068) |####################| Elapsed Time: 0:00:05 Time: 0:00:05
100% (3068 of 3068) |####################| Elapsed Time: 0:00:00 Time: 0:00:00
Started Log Value: 0.22961045652627946
100% (52 of 52) |########################| Elapsed Time: 0:00:00 Time: 0:00:00
Got 3068 median-enabled pixels
5-Number stats for medians: (3068, 3068, 0.3318104865334491, 0.5890882557317825, 0.6596343025206233, 0.728007829747179, 0.9846777519403345)
Started Log Value: 0.35571027994155885
Collecting fold changes
100% (52 of 52) |########################| Elapsed Time: 0:00:24 Time: 0:00:24
Got a total of 54917200 fold changes
Median elements [27458600]
Median elements
Global Median 0.64714
2020-09-30 23:01:10,064 SpectraRegion INFO: dimensions inputarray: 17900
2020-09-30 23:01:10,065 SpectraRegion INFO: Creating C++ obj
2020-09-30 23:01:10,066 SpectraRegion INFO: 17900 (52, 59, 17900)
2020-09-30 23:01:10,066 SpectraRegion INFO: dimensions 17900
2020-09-30 23:01:10,066 SpectraRegion INFO: input dimensions (52, 59, 17900)
2020-09-30 23:01:10,067 SpectraRegion INFO: Switching to dot mode
2020-09-30 23:01:10,148 SpectraRegion INFO: Starting calc similarity c++
2020-09-30 23:02:17,213 SpectraRegion INFO: outclust dimensions (3068, 3068)
2020-09-30 23:02:17,215 SpectraRegion INFO: Calculating spectra similarity
2020-09-30 23:02:17,337 SpectraRegion INFO: Calculating spectra similarity done
2020-09-30 23:02:17,338 SpectraRegion INFO: Calculating dist pixel map
2020-09-30 23:03:06,611 SpectraRegion INFO: Calculating dist pixel map done
2020-09-30 23:03:06,647 SpectraRegion INFO: Calculating clusters
2020-09-30 23:03:06,866 SpectraRegion INFO: Calculating clusters done
2020-09-30 23:03:06,885 SpectraRegion INFO: Calculating clusters saved
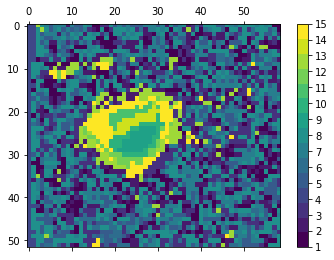
2020-09-30 23:03:06,991 SpectraRegion INFO: Assigning clusters to background: {1, 2, 3, 4, 5, 6, 7, 8, 13}
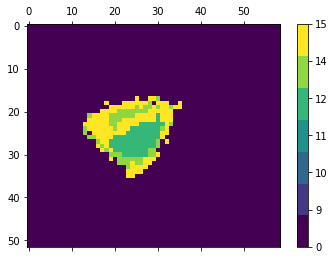
2020-09-30 23:03:07,090 SpectraRegion INFO: DE data for case: [15]
2020-09-30 23:03:07,090 SpectraRegion INFO: DE data for control: [12, 11, 14, 10, 9]
2020-09-30 23:03:07,091 SpectraRegion INFO: Running [15] against [12, 11, 14, 10, 9]
2020-09-30 23:03:07,092 SpectraRegion INFO: DE result key: ((15,), (9, 10, 11, 12, 14))
2020-09-30 23:03:07,187 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 253)
2020-09-30 23:03:07,189 SpectraRegion INFO: DE Sample DataFrame ready. Shape (253, 3)
2020-09-30 23:03:07,192 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 23:03:07,287 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((15,), (9, 10, 11, 12, 14))
2020-09-30 23:03:07,288 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 23:03:10,759 SpectraRegion INFO: DE-test (rank) finished. Results available: ((15,), (9, 10, 11, 12, 14))
2020-09-30 23:03:10,762 SpectraRegion INFO: DE result for case ((15,), (9, 10, 11, 12, 14)) with (17900, 7) results
2020-09-30 23:03:10,763 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:03:10,766 SpectraRegion INFO: DE result for case ((15,), (9, 10, 11, 12, 14)) with (367, 7) results (filtered)
2020-09-30 23:03:10,778 SpectraRegion INFO: Created matrices with shape (98, 17900) and (155, 17900) (target, bg)
2020-09-30 23:03:11,526 SpectraRegion INFO: DE result for case ((15,), (9, 10, 11, 12, 14)) with (17900, 7) results
2020-09-30 23:03:11,526 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:03:11,529 SpectraRegion INFO: DE result for case ((15,), (9, 10, 11, 12, 14)) with (365, 7) results (filtered)
2020-09-30 23:03:11,541 SpectraRegion INFO: Created matrices with shape (98, 17900) and (155, 17900) (target, bg)
2020-09-30 23:03:12,289 SpectraRegion INFO: DE data for case: [12]
2020-09-30 23:03:12,290 SpectraRegion INFO: DE data for control: [15, 11, 14, 10, 9]
2020-09-30 23:03:12,290 SpectraRegion INFO: Running [12] against [15, 11, 14, 10, 9]
2020-09-30 23:03:12,291 SpectraRegion INFO: DE result key: ((12,), (9, 10, 11, 14, 15))
2020-09-30 23:03:12,381 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 253)
2020-09-30 23:03:12,383 SpectraRegion INFO: DE Sample DataFrame ready. Shape (253, 3)
2020-09-30 23:03:12,387 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 23:03:12,481 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((12,), (9, 10, 11, 14, 15))
2020-09-30 23:03:12,482 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 23:03:15,982 SpectraRegion INFO: DE-test (rank) finished. Results available: ((12,), (9, 10, 11, 14, 15))
2020-09-30 23:03:15,984 SpectraRegion INFO: DE result for case ((12,), (9, 10, 11, 14, 15)) with (17900, 7) results
2020-09-30 23:03:15,984 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:03:15,987 SpectraRegion INFO: DE result for case ((12,), (9, 10, 11, 14, 15)) with (293, 7) results (filtered)
2020-09-30 23:03:15,998 SpectraRegion INFO: Created matrices with shape (33, 17900) and (220, 17900) (target, bg)
2020-09-30 23:03:16,291 SpectraRegion INFO: DE result for case ((12,), (9, 10, 11, 14, 15)) with (17900, 7) results
2020-09-30 23:03:16,292 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:03:16,295 SpectraRegion INFO: DE result for case ((12,), (9, 10, 11, 14, 15)) with (223, 7) results (filtered)
2020-09-30 23:03:16,306 SpectraRegion INFO: Created matrices with shape (33, 17900) and (220, 17900) (target, bg)
2020-09-30 23:03:16,505 SpectraRegion INFO: DE data for case: [11]
2020-09-30 23:03:16,506 SpectraRegion INFO: DE data for control: [15, 12, 14, 10, 9]
2020-09-30 23:03:16,506 SpectraRegion INFO: Running [11] against [15, 12, 14, 10, 9]
2020-09-30 23:03:16,507 SpectraRegion INFO: DE result key: ((11,), (9, 10, 12, 14, 15))
2020-09-30 23:03:16,595 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 253)
2020-09-30 23:03:16,598 SpectraRegion INFO: DE Sample DataFrame ready. Shape (253, 3)
2020-09-30 23:03:16,602 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 23:03:16,703 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((11,), (9, 10, 12, 14, 15))
2020-09-30 23:03:16,704 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 23:03:20,167 SpectraRegion INFO: DE-test (rank) finished. Results available: ((11,), (9, 10, 12, 14, 15))
2020-09-30 23:03:20,169 SpectraRegion INFO: DE result for case ((11,), (9, 10, 12, 14, 15)) with (17900, 7) results
2020-09-30 23:03:20,170 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:03:20,174 SpectraRegion INFO: DE result for case ((11,), (9, 10, 12, 14, 15)) with (2113, 7) results (filtered)
2020-09-30 23:03:20,185 SpectraRegion INFO: Created matrices with shape (24, 17900) and (229, 17900) (target, bg)
2020-09-30 23:03:25,540 SpectraRegion INFO: DE result for case ((11,), (9, 10, 12, 14, 15)) with (17900, 7) results
2020-09-30 23:03:25,541 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:03:25,544 SpectraRegion INFO: DE result for case ((11,), (9, 10, 12, 14, 15)) with (1869, 7) results (filtered)
2020-09-30 23:03:25,554 SpectraRegion INFO: Created matrices with shape (24, 17900) and (229, 17900) (target, bg)
2020-09-30 23:03:30,401 SpectraRegion INFO: DE data for case: [14]
2020-09-30 23:03:30,402 SpectraRegion INFO: DE data for control: [15, 12, 11, 10, 9]
2020-09-30 23:03:30,402 SpectraRegion INFO: Running [14] against [15, 12, 11, 10, 9]
2020-09-30 23:03:30,403 SpectraRegion INFO: DE result key: ((14,), (9, 10, 11, 12, 15))
2020-09-30 23:03:30,494 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 253)
2020-09-30 23:03:30,496 SpectraRegion INFO: DE Sample DataFrame ready. Shape (253, 3)
2020-09-30 23:03:30,501 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 23:03:30,603 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((14,), (9, 10, 11, 12, 15))
2020-09-30 23:03:30,604 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 23:03:34,078 SpectraRegion INFO: DE-test (rank) finished. Results available: ((14,), (9, 10, 11, 12, 15))
2020-09-30 23:03:34,080 SpectraRegion INFO: DE result for case ((14,), (9, 10, 11, 12, 15)) with (17900, 7) results
2020-09-30 23:03:34,080 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:03:34,084 SpectraRegion INFO: DE result for case ((14,), (9, 10, 11, 12, 15)) with (952, 7) results (filtered)
2020-09-30 23:03:34,096 SpectraRegion INFO: Created matrices with shape (28, 17900) and (225, 17900) (target, bg)
2020-09-30 23:03:36,481 SpectraRegion INFO: DE result for case ((14,), (9, 10, 11, 12, 15)) with (17900, 7) results
2020-09-30 23:03:36,482 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:03:36,485 SpectraRegion INFO: DE result for case ((14,), (9, 10, 11, 12, 15)) with (854, 7) results (filtered)
2020-09-30 23:03:36,498 SpectraRegion INFO: Created matrices with shape (28, 17900) and (225, 17900) (target, bg)
2020-09-30 23:03:38,597 SpectraRegion INFO: DE data for case: [10]
2020-09-30 23:03:38,598 SpectraRegion INFO: DE data for control: [15, 12, 11, 14, 9]
2020-09-30 23:03:38,599 SpectraRegion INFO: Running [10] against [15, 12, 11, 14, 9]
2020-09-30 23:03:38,599 SpectraRegion INFO: DE result key: ((10,), (9, 11, 12, 14, 15))
2020-09-30 23:03:38,688 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 253)
2020-09-30 23:03:38,690 SpectraRegion INFO: DE Sample DataFrame ready. Shape (253, 3)
2020-09-30 23:03:38,694 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 23:03:38,795 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((10,), (9, 11, 12, 14, 15))
2020-09-30 23:03:38,796 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 23:03:42,277 SpectraRegion INFO: DE-test (rank) finished. Results available: ((10,), (9, 11, 12, 14, 15))
2020-09-30 23:03:42,279 SpectraRegion INFO: DE result for case ((10,), (9, 11, 12, 14, 15)) with (17900, 7) results
2020-09-30 23:03:42,280 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:03:42,283 SpectraRegion INFO: DE result for case ((10,), (9, 11, 12, 14, 15)) with (536, 7) results (filtered)
2020-09-30 23:03:42,294 SpectraRegion INFO: Created matrices with shape (36, 17900) and (217, 17900) (target, bg)
2020-09-30 23:03:43,513 SpectraRegion INFO: DE result for case ((10,), (9, 11, 12, 14, 15)) with (17900, 7) results
2020-09-30 23:03:43,514 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:03:43,517 SpectraRegion INFO: DE result for case ((10,), (9, 11, 12, 14, 15)) with (540, 7) results (filtered)
2020-09-30 23:03:43,528 SpectraRegion INFO: Created matrices with shape (36, 17900) and (217, 17900) (target, bg)
2020-09-30 23:03:44,758 SpectraRegion INFO: DE data for case: [9]
2020-09-30 23:03:44,758 SpectraRegion INFO: DE data for control: [15, 12, 11, 14, 10]
2020-09-30 23:03:44,759 SpectraRegion INFO: Running [9] against [15, 12, 11, 14, 10]
2020-09-30 23:03:44,759 SpectraRegion INFO: DE result key: ((9,), (10, 11, 12, 14, 15))
2020-09-30 23:03:44,847 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 253)
2020-09-30 23:03:44,849 SpectraRegion INFO: DE Sample DataFrame ready. Shape (253, 3)
2020-09-30 23:03:44,853 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 23:03:44,956 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((9,), (10, 11, 12, 14, 15))
2020-09-30 23:03:44,956 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 23:03:48,437 SpectraRegion INFO: DE-test (rank) finished. Results available: ((9,), (10, 11, 12, 14, 15))
2020-09-30 23:03:48,440 SpectraRegion INFO: DE result for case ((9,), (10, 11, 12, 14, 15)) with (17900, 7) results
2020-09-30 23:03:48,440 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:03:48,443 SpectraRegion INFO: DE result for case ((9,), (10, 11, 12, 14, 15)) with (1249, 7) results (filtered)
2020-09-30 23:03:48,456 SpectraRegion INFO: Created matrices with shape (34, 17900) and (219, 17900) (target, bg)
2020-09-30 23:03:51,827 SpectraRegion INFO: DE result for case ((9,), (10, 11, 12, 14, 15)) with (17900, 7) results
2020-09-30 23:03:51,828 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:03:51,831 SpectraRegion INFO: DE result for case ((9,), (10, 11, 12, 14, 15)) with (1222, 7) results (filtered)
2020-09-30 23:03:51,842 SpectraRegion INFO: Created matrices with shape (34, 17900) and (219, 17900) (target, bg)
2020-09-30 23:03:55,113 SpectraRegion INFO: DE data for case: [0]
2020-09-30 23:03:55,114 SpectraRegion INFO: DE data for control: [15, 12, 11, 14, 10, 9]
2020-09-30 23:03:55,114 SpectraRegion INFO: Running [0] against [15, 12, 11, 14, 10, 9]
2020-09-30 23:03:55,115 SpectraRegion INFO: DE result key: ((0,), (9, 10, 11, 12, 14, 15))
2020-09-30 23:03:59,833 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 3068)
2020-09-30 23:03:59,836 SpectraRegion INFO: DE Sample DataFrame ready. Shape (3068, 3)
2020-09-30 23:03:59,840 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 23:04:01,337 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((0,), (9, 10, 11, 12, 14, 15))
2020-09-30 23:04:01,338 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 23:04:12,758 SpectraRegion INFO: DE-test (rank) finished. Results available: ((0,), (9, 10, 11, 12, 14, 15))
2020-09-30 23:04:12,761 SpectraRegion INFO: DE result for case ((0,), (9, 10, 11, 12, 14, 15)) with (17900, 7) results
2020-09-30 23:04:12,762 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:04:12,765 SpectraRegion INFO: DE result for case ((0,), (9, 10, 11, 12, 14, 15)) with (1125, 7) results (filtered)
2020-09-30 23:04:12,842 SpectraRegion INFO: Created matrices with shape (2815, 17900) and (253, 17900) (target, bg)
2020-09-30 23:04:15,510 SpectraRegion INFO: DE result for case ((0,), (9, 10, 11, 12, 14, 15)) with (17900, 7) results
2020-09-30 23:04:15,511 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:04:15,513 SpectraRegion INFO: DE result for case ((0,), (9, 10, 11, 12, 14, 15)) with (1125, 7) results (filtered)
2020-09-30 23:04:15,591 SpectraRegion INFO: Created matrices with shape (2815, 17900) and (253, 17900) (target, bg)
2020-09-30 23:04:18,172 SpectraRegion INFO: DE data for case: [15]
2020-09-30 23:04:18,172 SpectraRegion INFO: DE data for control: [0, 12, 11, 14, 10, 9]
2020-09-30 23:04:18,173 SpectraRegion INFO: Running [15] against [0, 12, 11, 14, 10, 9]
2020-09-30 23:04:18,173 SpectraRegion INFO: DE result key: ((15,), (0, 9, 10, 11, 12, 14))
2020-09-30 23:04:23,163 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 3068)
2020-09-30 23:04:23,166 SpectraRegion INFO: DE Sample DataFrame ready. Shape (3068, 3)
2020-09-30 23:04:23,171 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 23:04:24,603 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((15,), (0, 9, 10, 11, 12, 14))
2020-09-30 23:04:24,604 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 23:04:36,233 SpectraRegion INFO: DE-test (rank) finished. Results available: ((15,), (0, 9, 10, 11, 12, 14))
2020-09-30 23:04:36,237 SpectraRegion INFO: DE result for case ((15,), (0, 9, 10, 11, 12, 14)) with (17900, 7) results
2020-09-30 23:04:36,238 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:04:36,241 SpectraRegion INFO: DE result for case ((15,), (0, 9, 10, 11, 12, 14)) with (863, 7) results (filtered)
2020-09-30 23:04:36,320 SpectraRegion INFO: Created matrices with shape (98, 17900) and (2970, 17900) (target, bg)
2020-09-30 23:04:38,420 SpectraRegion INFO: DE result for case ((15,), (0, 9, 10, 11, 12, 14)) with (17900, 7) results
2020-09-30 23:04:38,420 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:04:38,423 SpectraRegion INFO: DE result for case ((15,), (0, 9, 10, 11, 12, 14)) with (863, 7) results (filtered)
2020-09-30 23:04:38,505 SpectraRegion INFO: Created matrices with shape (98, 17900) and (2970, 17900) (target, bg)
2020-09-30 23:04:40,574 SpectraRegion INFO: DE data for case: [12]
2020-09-30 23:04:40,575 SpectraRegion INFO: DE data for control: [0, 15, 11, 14, 10, 9]
2020-09-30 23:04:40,576 SpectraRegion INFO: Running [12] against [0, 15, 11, 14, 10, 9]
2020-09-30 23:04:40,577 SpectraRegion INFO: DE result key: ((12,), (0, 9, 10, 11, 14, 15))
2020-09-30 23:04:45,346 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 3068)
2020-09-30 23:04:45,350 SpectraRegion INFO: DE Sample DataFrame ready. Shape (3068, 3)
2020-09-30 23:04:45,355 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 23:04:46,839 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((12,), (0, 9, 10, 11, 14, 15))
2020-09-30 23:04:46,840 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 23:04:58,758 SpectraRegion INFO: DE-test (rank) finished. Results available: ((12,), (0, 9, 10, 11, 14, 15))
2020-09-30 23:04:58,763 SpectraRegion INFO: DE result for case ((12,), (0, 9, 10, 11, 14, 15)) with (17900, 7) results
2020-09-30 23:04:58,764 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:04:58,766 SpectraRegion INFO: DE result for case ((12,), (0, 9, 10, 11, 14, 15)) with (856, 7) results (filtered)
2020-09-30 23:04:58,854 SpectraRegion INFO: Created matrices with shape (33, 17900) and (3035, 17900) (target, bg)
2020-09-30 23:05:01,063 SpectraRegion INFO: DE result for case ((12,), (0, 9, 10, 11, 14, 15)) with (17900, 7) results
2020-09-30 23:05:01,064 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:05:01,067 SpectraRegion INFO: DE result for case ((12,), (0, 9, 10, 11, 14, 15)) with (855, 7) results (filtered)
2020-09-30 23:05:01,152 SpectraRegion INFO: Created matrices with shape (33, 17900) and (3035, 17900) (target, bg)
2020-09-30 23:05:03,372 SpectraRegion INFO: DE data for case: [11]
2020-09-30 23:05:03,373 SpectraRegion INFO: DE data for control: [0, 15, 12, 14, 10, 9]
2020-09-30 23:05:03,374 SpectraRegion INFO: Running [11] against [0, 15, 12, 14, 10, 9]
2020-09-30 23:05:03,374 SpectraRegion INFO: DE result key: ((11,), (0, 9, 10, 12, 14, 15))
2020-09-30 23:05:08,311 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 3068)
2020-09-30 23:05:08,315 SpectraRegion INFO: DE Sample DataFrame ready. Shape (3068, 3)
2020-09-30 23:05:08,319 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 23:05:09,696 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((11,), (0, 9, 10, 12, 14, 15))
2020-09-30 23:05:09,697 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 23:05:21,420 SpectraRegion INFO: DE-test (rank) finished. Results available: ((11,), (0, 9, 10, 12, 14, 15))
2020-09-30 23:05:21,424 SpectraRegion INFO: DE result for case ((11,), (0, 9, 10, 12, 14, 15)) with (17900, 7) results
2020-09-30 23:05:21,425 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:05:21,429 SpectraRegion INFO: DE result for case ((11,), (0, 9, 10, 12, 14, 15)) with (2583, 7) results (filtered)
2020-09-30 23:05:21,507 SpectraRegion INFO: Created matrices with shape (24, 17900) and (3044, 17900) (target, bg)
2020-09-30 23:05:30,515 SpectraRegion INFO: DE result for case ((11,), (0, 9, 10, 12, 14, 15)) with (17900, 7) results
2020-09-30 23:05:30,516 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:05:30,520 SpectraRegion INFO: DE result for case ((11,), (0, 9, 10, 12, 14, 15)) with (2542, 7) results (filtered)
2020-09-30 23:05:30,598 SpectraRegion INFO: Created matrices with shape (24, 17900) and (3044, 17900) (target, bg)
2020-09-30 23:05:39,484 SpectraRegion INFO: DE data for case: [14]
2020-09-30 23:05:39,484 SpectraRegion INFO: DE data for control: [0, 15, 12, 11, 10, 9]
2020-09-30 23:05:39,485 SpectraRegion INFO: Running [14] against [0, 15, 12, 11, 10, 9]
2020-09-30 23:05:39,485 SpectraRegion INFO: DE result key: ((14,), (0, 9, 10, 11, 12, 15))
2020-09-30 23:05:44,160 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 3068)
2020-09-30 23:05:44,164 SpectraRegion INFO: DE Sample DataFrame ready. Shape (3068, 3)
2020-09-30 23:05:44,170 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 23:05:45,547 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((14,), (0, 9, 10, 11, 12, 15))
2020-09-30 23:05:45,548 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 23:05:57,475 SpectraRegion INFO: DE-test (rank) finished. Results available: ((14,), (0, 9, 10, 11, 12, 15))
2020-09-30 23:05:57,479 SpectraRegion INFO: DE result for case ((14,), (0, 9, 10, 11, 12, 15)) with (17900, 7) results
2020-09-30 23:05:57,480 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:05:57,483 SpectraRegion INFO: DE result for case ((14,), (0, 9, 10, 11, 12, 15)) with (2209, 7) results (filtered)
2020-09-30 23:05:57,560 SpectraRegion INFO: Created matrices with shape (28, 17900) and (3040, 17900) (target, bg)
2020-09-30 23:06:03,976 SpectraRegion INFO: DE result for case ((14,), (0, 9, 10, 11, 12, 15)) with (17900, 7) results
2020-09-30 23:06:03,977 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:06:03,981 SpectraRegion INFO: DE result for case ((14,), (0, 9, 10, 11, 12, 15)) with (2219, 7) results (filtered)
2020-09-30 23:06:04,074 SpectraRegion INFO: Created matrices with shape (28, 17900) and (3040, 17900) (target, bg)
2020-09-30 23:06:10,288 SpectraRegion INFO: DE data for case: [10]
2020-09-30 23:06:10,289 SpectraRegion INFO: DE data for control: [0, 15, 12, 11, 14, 9]
2020-09-30 23:06:10,289 SpectraRegion INFO: Running [10] against [0, 15, 12, 11, 14, 9]
2020-09-30 23:06:10,290 SpectraRegion INFO: DE result key: ((10,), (0, 9, 11, 12, 14, 15))
2020-09-30 23:06:15,066 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 3068)
2020-09-30 23:06:15,070 SpectraRegion INFO: DE Sample DataFrame ready. Shape (3068, 3)
2020-09-30 23:06:15,075 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 23:06:16,456 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((10,), (0, 9, 11, 12, 14, 15))
2020-09-30 23:06:16,457 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 23:06:28,151 SpectraRegion INFO: DE-test (rank) finished. Results available: ((10,), (0, 9, 11, 12, 14, 15))
2020-09-30 23:06:28,155 SpectraRegion INFO: DE result for case ((10,), (0, 9, 11, 12, 14, 15)) with (17900, 7) results
2020-09-30 23:06:28,155 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:06:28,159 SpectraRegion INFO: DE result for case ((10,), (0, 9, 11, 12, 14, 15)) with (1799, 7) results (filtered)
2020-09-30 23:06:28,236 SpectraRegion INFO: Created matrices with shape (36, 17900) and (3032, 17900) (target, bg)
2020-09-30 23:06:32,655 SpectraRegion INFO: DE result for case ((10,), (0, 9, 11, 12, 14, 15)) with (17900, 7) results
2020-09-30 23:06:32,655 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:06:32,659 SpectraRegion INFO: DE result for case ((10,), (0, 9, 11, 12, 14, 15)) with (1807, 7) results (filtered)
2020-09-30 23:06:32,735 SpectraRegion INFO: Created matrices with shape (36, 17900) and (3032, 17900) (target, bg)
2020-09-30 23:06:37,083 SpectraRegion INFO: DE data for case: [9]
2020-09-30 23:06:37,084 SpectraRegion INFO: DE data for control: [0, 15, 12, 11, 14, 10]
2020-09-30 23:06:37,085 SpectraRegion INFO: Running [9] against [0, 15, 12, 11, 14, 10]
2020-09-30 23:06:37,086 SpectraRegion INFO: DE result key: ((9,), (0, 10, 11, 12, 14, 15))
2020-09-30 23:06:41,784 SpectraRegion INFO: DE DataFrame ready. Shape (17900, 3068)
2020-09-30 23:06:41,789 SpectraRegion INFO: DE Sample DataFrame ready. Shape (3068, 3)
2020-09-30 23:06:41,793 SpectraRegion INFO: Performing DE-test: ttest
2020-09-30 23:06:43,204 SpectraRegion INFO: DE-test (ttest) finished. Results available: ((9,), (0, 10, 11, 12, 14, 15))
2020-09-30 23:06:43,205 SpectraRegion INFO: Performing DE-test: rank
2020-09-30 23:06:54,998 SpectraRegion INFO: DE-test (rank) finished. Results available: ((9,), (0, 10, 11, 12, 14, 15))
2020-09-30 23:06:55,002 SpectraRegion INFO: DE result for case ((9,), (0, 10, 11, 12, 14, 15)) with (17900, 7) results
2020-09-30 23:06:55,003 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:06:55,007 SpectraRegion INFO: DE result for case ((9,), (0, 10, 11, 12, 14, 15)) with (1932, 7) results (filtered)
2020-09-30 23:06:55,087 SpectraRegion INFO: Created matrices with shape (34, 17900) and (3034, 17900) (target, bg)
2020-09-30 23:07:00,317 SpectraRegion INFO: DE result for case ((9,), (0, 10, 11, 12, 14, 15)) with (17900, 7) results
2020-09-30 23:07:00,318 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:07:00,321 SpectraRegion INFO: DE result for case ((9,), (0, 10, 11, 12, 14, 15)) with (1937, 7) results (filtered)
2020-09-30 23:07:00,405 SpectraRegion INFO: Created matrices with shape (34, 17900) and (3034, 17900) (target, bg)
#loggers = [logging.getLogger(name) for name in logging.root.manager.loggerDict]
#for logger in loggers:
# logger.setLevel(logging.INFO)
markerGenes0 = slided0_mgenes["ttest"]
markerGenes0.to_csv("deresults/marker_genes_region_0.tsv", sep="\t", index=False)
markerGenes1 = slided1_mgenes["ttest"]
markerGenes1.to_csv("deresults/marker_genes_region_1.tsv", sep="\t", index=False)
markerGenes4 = slided4_mgenes["ttest"]
markerGenes4.to_csv("deresults/marker_genes_region_4.tsv", sep="\t", index=False)
markerGenes5 = slided5_mgenes["ttest"]
markerGenes5.to_csv("deresults/marker_genes_region_5.tsv", sep="\t", index=False)
! python3 analyseMarkers.py --organs "Connective tissue" "Vasculature" "Heart" "Skeletal Muscle" "Smooth muscle" "Immune system" "Blood" "Epithelium" --pvaladj qvalue --markers deresults/marker_genes_region_0.tsv --aorta3d --output deresults/marker_genes_region_0.pred.tsv
! python3 analyseMarkers.py --organs "Connective tissue" "Vasculature" "Heart" "Skeletal Muscle" "Smooth muscle" "Immune system" "Blood" "Epithelium" --pvaladj qvalue --markers deresults/marker_genes_region_1.tsv --aorta3d --output deresults/marker_genes_region_1.pred.tsv
! python3 analyseMarkers.py --organs "Connective tissue" "Vasculature" "Heart" "Skeletal Muscle" "Smooth muscle" "Immune system" "Blood" "Epithelium" --pvaladj qvalue --markers deresults/marker_genes_region_4.tsv --aorta3d --output deresults/marker_genes_region_4.pred.tsv
! python3 analyseMarkers.py --organs "Connective tissue" "Vasculature" "Heart" "Skeletal Muscle" "Smooth muscle" "Immune system" "Blood" "Epithelium" --pvaladj qvalue --markers deresults/marker_genes_region_5.tsv --aorta3d --output deresults/marker_genes_region_5.pred.tsv
Setting number of predictions to 1
Taking value gene from gene
Taking value cluster from clusterID
Taking value logfc from avg_logFC
Taking value pvaladj from qvalue
Taking value expr-mean from mean
Taking value expressing-cell-count from num
Taking value cluster-cell-count from anum
Got 9 clusters.
Starting analysis
Loaded Databases
known genes 38
known (celltype, organ) 50
Setting number of predictions to 1
Taking value gene from gene
Taking value cluster from clusterID
Taking value logfc from avg_logFC
Taking value pvaladj from qvalue
Taking value expr-mean from mean
Taking value expressing-cell-count from num
Taking value cluster-cell-count from anum
Got 7 clusters.
Starting analysis
Loaded Databases
known genes 26
known (celltype, organ) 41
Setting number of predictions to 1
Taking value gene from gene
Taking value cluster from clusterID
Taking value logfc from avg_logFC
Taking value pvaladj from qvalue
Taking value expr-mean from mean
Taking value expressing-cell-count from num
Taking value cluster-cell-count from anum
Got 9 clusters.
Starting analysis
Loaded Databases
known genes 33
known (celltype, organ) 47
Setting number of predictions to 1
Taking value gene from gene
Taking value cluster from clusterID
Taking value logfc from avg_logFC
Taking value pvaladj from qvalue
Taking value expr-mean from mean
Taking value expressing-cell-count from num
Taking value cluster-cell-count from anum
Got 6 clusters.
Starting analysis
Loaded Databases
known genes 48
known (celltype, organ) 54
slided_0.to_aorta3d("./aorta3d/slided/", "slided", 0, protWeights=pw, ctpred="deresults/marker_genes_region_0.pred.tsv")
slided_1.to_aorta3d("./aorta3d/slided/", "slided", 1, protWeights=pw, ctpred="deresults/marker_genes_region_1.pred.tsv")
slided_4.to_aorta3d("./aorta3d/slided/", "slided", 4, protWeights=pw, ctpred="deresults/marker_genes_region_4.pred.tsv")
slided_5.to_aorta3d("./aorta3d/slided/", "slided", 5, protWeights=pw, ctpred="deresults/marker_genes_region_5.pred.tsv")
2020-09-30 23:37:25,306 SpectraRegion INFO: Cell-type assigned: 1 -> Monocytes;Immune system
2020-09-30 23:37:25,307 SpectraRegion INFO: Cell-type assigned: 2 -> Megakaryocytes;Immune system
2020-09-30 23:37:25,308 SpectraRegion INFO: Cell-type assigned: 4 -> Mast cells;Immune system
2020-09-30 23:37:25,308 SpectraRegion INFO: Cell-type assigned: 5 -> Monocytes;Immune system
2020-09-30 23:37:25,309 SpectraRegion INFO: Cell-type assigned: 6 -> Monocytes;Immune system
2020-09-30 23:37:25,309 SpectraRegion INFO: Cell-type assigned: 7 -> Mast cells;Immune system
2020-09-30 23:37:25,309 SpectraRegion INFO: Cell-type assigned: 8 -> Mast cells;Immune system
2020-09-30 23:37:25,310 SpectraRegion INFO: Cell-type assigned: 9 -> Smooth muscle cells;Smooth muscle
2020-09-30 23:37:25,319 SpectraRegion INFO: DE data for case: [0]
2020-09-30 23:37:25,320 SpectraRegion INFO: DE data for control: [5, 7, 9, 3, 8, 2, 4, 6, 1]
2020-09-30 23:37:25,320 SpectraRegion INFO: Running [0] against [5, 7, 9, 3, 8, 2, 4, 6, 1]
2020-09-30 23:37:25,321 SpectraRegion INFO: DE result key: ((0,), (1, 2, 3, 4, 5, 6, 7, 8, 9))
2020-09-30 23:37:25,321 SpectraRegion INFO: DE result key already exists
2020-09-30 23:37:25,324 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 23:37:25,324 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:37:25,327 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 6, 7, 8, 9)) with (1307, 7) results (filtered)
2020-09-30 23:37:25,411 SpectraRegion INFO: Created matrices with shape (1930, 17900) and (725, 17900) (target, bg)
2020-09-30 23:37:29,070 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 23:37:29,071 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:37:29,074 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 6, 7, 8, 9)) with (1307, 7) results (filtered)
2020-09-30 23:37:29,131 SpectraRegion INFO: Created matrices with shape (1930, 17900) and (725, 17900) (target, bg)
2020-09-30 23:37:32,660 SpectraRegion INFO: DE data for case: [5]
2020-09-30 23:37:32,661 SpectraRegion INFO: DE data for control: [0, 7, 9, 3, 8, 2, 4, 6, 1]
2020-09-30 23:37:32,661 SpectraRegion INFO: Running [5] against [0, 7, 9, 3, 8, 2, 4, 6, 1]
2020-09-30 23:37:32,662 SpectraRegion INFO: DE result key: ((5,), (0, 1, 2, 3, 4, 6, 7, 8, 9))
2020-09-30 23:37:32,662 SpectraRegion INFO: DE result key already exists
2020-09-30 23:37:32,664 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 23:37:32,665 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:37:32,667 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 6, 7, 8, 9)) with (450, 7) results (filtered)
2020-09-30 23:37:32,725 SpectraRegion INFO: Created matrices with shape (100, 17900) and (2555, 17900) (target, bg)
2020-09-30 23:37:34,183 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 23:37:34,184 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:37:34,187 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 6, 7, 8, 9)) with (450, 7) results (filtered)
2020-09-30 23:37:34,243 SpectraRegion INFO: Created matrices with shape (100, 17900) and (2555, 17900) (target, bg)
2020-09-30 23:37:35,675 SpectraRegion INFO: DE data for case: [7]
2020-09-30 23:37:35,676 SpectraRegion INFO: DE data for control: [0, 5, 9, 3, 8, 2, 4, 6, 1]
2020-09-30 23:37:35,676 SpectraRegion INFO: Running [7] against [0, 5, 9, 3, 8, 2, 4, 6, 1]
2020-09-30 23:37:35,676 SpectraRegion INFO: DE result key: ((7,), (0, 1, 2, 3, 4, 5, 6, 8, 9))
2020-09-30 23:37:35,677 SpectraRegion INFO: DE result key already exists
2020-09-30 23:37:35,679 SpectraRegion INFO: DE result for case ((7,), (0, 1, 2, 3, 4, 5, 6, 8, 9)) with (17900, 7) results
2020-09-30 23:37:35,679 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:37:35,682 SpectraRegion INFO: DE result for case ((7,), (0, 1, 2, 3, 4, 5, 6, 8, 9)) with (680, 7) results (filtered)
2020-09-30 23:37:35,741 SpectraRegion INFO: Created matrices with shape (161, 17900) and (2494, 17900) (target, bg)
2020-09-30 23:37:37,578 SpectraRegion INFO: DE result for case ((7,), (0, 1, 2, 3, 4, 5, 6, 8, 9)) with (17900, 7) results
2020-09-30 23:37:37,579 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:37:37,581 SpectraRegion INFO: DE result for case ((7,), (0, 1, 2, 3, 4, 5, 6, 8, 9)) with (680, 7) results (filtered)
2020-09-30 23:37:37,639 SpectraRegion INFO: Created matrices with shape (161, 17900) and (2494, 17900) (target, bg)
2020-09-30 23:37:39,489 SpectraRegion INFO: DE data for case: [9]
2020-09-30 23:37:39,490 SpectraRegion INFO: DE data for control: [0, 5, 7, 3, 8, 2, 4, 6, 1]
2020-09-30 23:37:39,490 SpectraRegion INFO: Running [9] against [0, 5, 7, 3, 8, 2, 4, 6, 1]
2020-09-30 23:37:39,491 SpectraRegion INFO: DE result key: ((9,), (0, 1, 2, 3, 4, 5, 6, 7, 8))
2020-09-30 23:37:39,491 SpectraRegion INFO: DE result key already exists
2020-09-30 23:37:39,493 SpectraRegion INFO: DE result for case ((9,), (0, 1, 2, 3, 4, 5, 6, 7, 8)) with (17900, 7) results
2020-09-30 23:37:39,493 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:37:39,496 SpectraRegion INFO: DE result for case ((9,), (0, 1, 2, 3, 4, 5, 6, 7, 8)) with (88, 7) results (filtered)
2020-09-30 23:37:39,553 SpectraRegion INFO: Created matrices with shape (89, 17900) and (2566, 17900) (target, bg)
2020-09-30 23:37:39,635 SpectraRegion INFO: DE result for case ((9,), (0, 1, 2, 3, 4, 5, 6, 7, 8)) with (17900, 7) results
2020-09-30 23:37:39,635 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:37:39,638 SpectraRegion INFO: DE result for case ((9,), (0, 1, 2, 3, 4, 5, 6, 7, 8)) with (65, 7) results (filtered)
2020-09-30 23:37:39,694 SpectraRegion INFO: Created matrices with shape (89, 17900) and (2566, 17900) (target, bg)
2020-09-30 23:37:39,752 SpectraRegion INFO: DE data for case: [3]
2020-09-30 23:37:39,753 SpectraRegion INFO: DE data for control: [0, 5, 7, 9, 8, 2, 4, 6, 1]
2020-09-30 23:37:39,754 SpectraRegion INFO: Running [3] against [0, 5, 7, 9, 8, 2, 4, 6, 1]
2020-09-30 23:37:39,754 SpectraRegion INFO: DE result key: ((3,), (0, 1, 2, 4, 5, 6, 7, 8, 9))
2020-09-30 23:37:39,754 SpectraRegion INFO: DE result key already exists
2020-09-30 23:37:39,757 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 23:37:39,757 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:37:39,760 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 6, 7, 8, 9)) with (1263, 7) results (filtered)
2020-09-30 23:37:39,816 SpectraRegion INFO: Created matrices with shape (51, 17900) and (2604, 17900) (target, bg)
2020-09-30 23:37:43,327 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 23:37:43,328 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:37:43,331 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 6, 7, 8, 9)) with (1261, 7) results (filtered)
2020-09-30 23:37:43,387 SpectraRegion INFO: Created matrices with shape (51, 17900) and (2604, 17900) (target, bg)
2020-09-30 23:37:46,934 SpectraRegion INFO: DE data for case: [8]
2020-09-30 23:37:46,935 SpectraRegion INFO: DE data for control: [0, 5, 7, 9, 3, 2, 4, 6, 1]
2020-09-30 23:37:46,935 SpectraRegion INFO: Running [8] against [0, 5, 7, 9, 3, 2, 4, 6, 1]
2020-09-30 23:37:46,936 SpectraRegion INFO: DE result key: ((8,), (0, 1, 2, 3, 4, 5, 6, 7, 9))
2020-09-30 23:37:46,937 SpectraRegion INFO: DE result key already exists
2020-09-30 23:37:46,938 SpectraRegion INFO: DE result for case ((8,), (0, 1, 2, 3, 4, 5, 6, 7, 9)) with (17900, 7) results
2020-09-30 23:37:46,939 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:37:46,941 SpectraRegion INFO: DE result for case ((8,), (0, 1, 2, 3, 4, 5, 6, 7, 9)) with (253, 7) results (filtered)
2020-09-30 23:37:47,002 SpectraRegion INFO: Created matrices with shape (55, 17900) and (2600, 17900) (target, bg)
2020-09-30 23:37:47,468 SpectraRegion INFO: DE result for case ((8,), (0, 1, 2, 3, 4, 5, 6, 7, 9)) with (17900, 7) results
2020-09-30 23:37:47,469 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:37:47,471 SpectraRegion INFO: DE result for case ((8,), (0, 1, 2, 3, 4, 5, 6, 7, 9)) with (250, 7) results (filtered)
2020-09-30 23:37:47,533 SpectraRegion INFO: Created matrices with shape (55, 17900) and (2600, 17900) (target, bg)
2020-09-30 23:37:47,974 SpectraRegion INFO: DE data for case: [2]
2020-09-30 23:37:47,975 SpectraRegion INFO: DE data for control: [0, 5, 7, 9, 3, 8, 4, 6, 1]
2020-09-30 23:37:47,975 SpectraRegion INFO: Running [2] against [0, 5, 7, 9, 3, 8, 4, 6, 1]
2020-09-30 23:37:47,976 SpectraRegion INFO: DE result key: ((2,), (0, 1, 3, 4, 5, 6, 7, 8, 9))
2020-09-30 23:37:47,976 SpectraRegion INFO: DE result key already exists
2020-09-30 23:37:47,978 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 23:37:47,979 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:37:47,982 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 6, 7, 8, 9)) with (1796, 7) results (filtered)
2020-09-30 23:37:48,044 SpectraRegion INFO: Created matrices with shape (150, 17900) and (2505, 17900) (target, bg)
2020-09-30 23:37:53,614 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 23:37:53,614 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:37:53,617 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 6, 7, 8, 9)) with (1796, 7) results (filtered)
2020-09-30 23:37:53,674 SpectraRegion INFO: Created matrices with shape (150, 17900) and (2505, 17900) (target, bg)
2020-09-30 23:37:59,200 SpectraRegion INFO: DE data for case: [4]
2020-09-30 23:37:59,201 SpectraRegion INFO: DE data for control: [0, 5, 7, 9, 3, 8, 2, 6, 1]
2020-09-30 23:37:59,201 SpectraRegion INFO: Running [4] against [0, 5, 7, 9, 3, 8, 2, 6, 1]
2020-09-30 23:37:59,202 SpectraRegion INFO: DE result key: ((4,), (0, 1, 2, 3, 5, 6, 7, 8, 9))
2020-09-30 23:37:59,202 SpectraRegion INFO: DE result key already exists
2020-09-30 23:37:59,204 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 23:37:59,205 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:37:59,207 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 6, 7, 8, 9)) with (1742, 7) results (filtered)
2020-09-30 23:37:59,264 SpectraRegion INFO: Created matrices with shape (70, 17900) and (2585, 17900) (target, bg)
2020-09-30 23:38:03,136 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 23:38:03,137 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:38:03,140 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 6, 7, 8, 9)) with (1742, 7) results (filtered)
2020-09-30 23:38:03,197 SpectraRegion INFO: Created matrices with shape (70, 17900) and (2585, 17900) (target, bg)
2020-09-30 23:38:07,049 SpectraRegion INFO: DE data for case: [6]
2020-09-30 23:38:07,050 SpectraRegion INFO: DE data for control: [0, 5, 7, 9, 3, 8, 2, 4, 1]
2020-09-30 23:38:07,050 SpectraRegion INFO: Running [6] against [0, 5, 7, 9, 3, 8, 2, 4, 1]
2020-09-30 23:38:07,051 SpectraRegion INFO: DE result key: ((6,), (0, 1, 2, 3, 4, 5, 7, 8, 9))
2020-09-30 23:38:07,051 SpectraRegion INFO: DE result key already exists
2020-09-30 23:38:07,053 SpectraRegion INFO: DE result for case ((6,), (0, 1, 2, 3, 4, 5, 7, 8, 9)) with (17900, 7) results
2020-09-30 23:38:07,054 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:38:07,056 SpectraRegion INFO: DE result for case ((6,), (0, 1, 2, 3, 4, 5, 7, 8, 9)) with (2053, 7) results (filtered)
2020-09-30 23:38:07,114 SpectraRegion INFO: Created matrices with shape (27, 17900) and (2628, 17900) (target, bg)
2020-09-30 23:38:12,974 SpectraRegion INFO: DE result for case ((6,), (0, 1, 2, 3, 4, 5, 7, 8, 9)) with (17900, 7) results
2020-09-30 23:38:12,974 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:38:12,977 SpectraRegion INFO: DE result for case ((6,), (0, 1, 2, 3, 4, 5, 7, 8, 9)) with (2058, 7) results (filtered)
2020-09-30 23:38:13,033 SpectraRegion INFO: Created matrices with shape (27, 17900) and (2628, 17900) (target, bg)
2020-09-30 23:38:18,905 SpectraRegion INFO: DE data for case: [1]
2020-09-30 23:38:18,906 SpectraRegion INFO: DE data for control: [0, 5, 7, 9, 3, 8, 2, 4, 6]
2020-09-30 23:38:18,907 SpectraRegion INFO: Running [1] against [0, 5, 7, 9, 3, 8, 2, 4, 6]
2020-09-30 23:38:18,908 SpectraRegion INFO: DE result key: ((1,), (0, 2, 3, 4, 5, 6, 7, 8, 9))
2020-09-30 23:38:18,908 SpectraRegion INFO: DE result key already exists
2020-09-30 23:38:18,910 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 23:38:18,910 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:38:18,913 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 6, 7, 8, 9)) with (3036, 7) results (filtered)
2020-09-30 23:38:18,970 SpectraRegion INFO: Created matrices with shape (22, 17900) and (2633, 17900) (target, bg)
2020-09-30 23:38:28,282 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-09-30 23:38:28,283 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:38:28,285 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 6, 7, 8, 9)) with (3039, 7) results (filtered)
2020-09-30 23:38:28,341 SpectraRegion INFO: Created matrices with shape (22, 17900) and (2633, 17900) (target, bg)
2020-09-30 23:38:37,734 SpectraRegion INFO: No cell type info for cluster: 0
2020-09-30 23:38:37,735 SpectraRegion INFO: No cell type info for cluster: 3
2020-09-30 23:38:37,745 SpectraRegion INFO: Cell-type assigned: 1 -> Megakaryocytes;Immune system
2020-09-30 23:38:37,746 SpectraRegion INFO: Cell-type assigned: 4 -> B cells;Immune system
2020-09-30 23:38:37,747 SpectraRegion INFO: Cell-type assigned: 5 -> Basophils;Immune system
2020-09-30 23:38:37,748 SpectraRegion INFO: Cell-type assigned: 7 -> Adipocytes;Connective tissue
2020-09-30 23:38:37,748 SpectraRegion INFO: Cell-type assigned: 15 -> Cardiomyocytes;Heart
2020-09-30 23:38:37,761 SpectraRegion INFO: DE data for case: [0]
2020-09-30 23:38:37,762 SpectraRegion INFO: DE data for control: [15, 2, 6, 3, 1, 7, 5, 4]
2020-09-30 23:38:37,763 SpectraRegion INFO: Running [0] against [15, 2, 6, 3, 1, 7, 5, 4]
2020-09-30 23:38:37,765 SpectraRegion INFO: DE result key: ((0,), (1, 2, 3, 4, 5, 6, 7, 15))
2020-09-30 23:38:37,766 SpectraRegion INFO: DE result key already exists
2020-09-30 23:38:37,768 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 6, 7, 15)) with (17900, 7) results
2020-09-30 23:38:37,769 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:38:37,774 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 6, 7, 15)) with (1086, 7) results (filtered)
2020-09-30 23:38:37,869 SpectraRegion INFO: Created matrices with shape (1923, 17900) and (485, 17900) (target, bg)
2020-09-30 23:38:40,673 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 6, 7, 15)) with (17900, 7) results
2020-09-30 23:38:40,673 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:38:40,675 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 6, 7, 15)) with (1086, 7) results (filtered)
2020-09-30 23:38:40,728 SpectraRegion INFO: Created matrices with shape (1923, 17900) and (485, 17900) (target, bg)
2020-09-30 23:38:43,559 SpectraRegion INFO: DE data for case: [15]
2020-09-30 23:38:43,560 SpectraRegion INFO: DE data for control: [0, 2, 6, 3, 1, 7, 5, 4]
2020-09-30 23:38:43,560 SpectraRegion INFO: Running [15] against [0, 2, 6, 3, 1, 7, 5, 4]
2020-09-30 23:38:43,560 SpectraRegion INFO: DE result key: ((15,), (0, 1, 2, 3, 4, 5, 6, 7))
2020-09-30 23:38:43,561 SpectraRegion INFO: DE result key already exists
2020-09-30 23:38:43,563 SpectraRegion INFO: DE result for case ((15,), (0, 1, 2, 3, 4, 5, 6, 7)) with (17900, 7) results
2020-09-30 23:38:43,563 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:38:43,566 SpectraRegion INFO: DE result for case ((15,), (0, 1, 2, 3, 4, 5, 6, 7)) with (12, 7) results (filtered)
2020-09-30 23:38:43,619 SpectraRegion INFO: Created matrices with shape (91, 17900) and (2317, 17900) (target, bg)
2020-09-30 23:38:43,637 SpectraRegion INFO: DE result for case ((15,), (0, 1, 2, 3, 4, 5, 6, 7)) with (17900, 7) results
2020-09-30 23:38:43,637 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:38:43,640 SpectraRegion INFO: DE result for case ((15,), (0, 1, 2, 3, 4, 5, 6, 7)) with (12, 7) results (filtered)
2020-09-30 23:38:43,748 SpectraRegion INFO: Created matrices with shape (91, 17900) and (2317, 17900) (target, bg)
2020-09-30 23:38:43,767 SpectraRegion INFO: DE data for case: [2]
2020-09-30 23:38:43,768 SpectraRegion INFO: DE data for control: [0, 15, 6, 3, 1, 7, 5, 4]
2020-09-30 23:38:43,768 SpectraRegion INFO: Running [2] against [0, 15, 6, 3, 1, 7, 5, 4]
2020-09-30 23:38:43,768 SpectraRegion INFO: DE result key: ((2,), (0, 1, 3, 4, 5, 6, 7, 15))
2020-09-30 23:38:43,769 SpectraRegion INFO: DE result key already exists
2020-09-30 23:38:43,771 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 6, 7, 15)) with (17900, 7) results
2020-09-30 23:38:43,771 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:38:43,774 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 6, 7, 15)) with (970, 7) results (filtered)
2020-09-30 23:38:43,829 SpectraRegion INFO: Created matrices with shape (79, 17900) and (2329, 17900) (target, bg)
2020-09-30 23:38:46,598 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 6, 7, 15)) with (17900, 7) results
2020-09-30 23:38:46,598 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:38:46,601 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 6, 7, 15)) with (970, 7) results (filtered)
2020-09-30 23:38:46,655 SpectraRegion INFO: Created matrices with shape (79, 17900) and (2329, 17900) (target, bg)
2020-09-30 23:38:49,444 SpectraRegion INFO: DE data for case: [6]
2020-09-30 23:38:49,445 SpectraRegion INFO: DE data for control: [0, 15, 2, 3, 1, 7, 5, 4]
2020-09-30 23:38:49,446 SpectraRegion INFO: Running [6] against [0, 15, 2, 3, 1, 7, 5, 4]
2020-09-30 23:38:49,446 SpectraRegion INFO: DE result key: ((6,), (0, 1, 2, 3, 4, 5, 7, 15))
2020-09-30 23:38:49,446 SpectraRegion INFO: DE result key already exists
2020-09-30 23:38:49,448 SpectraRegion INFO: DE result for case ((6,), (0, 1, 2, 3, 4, 5, 7, 15)) with (17900, 7) results
2020-09-30 23:38:49,449 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:38:49,451 SpectraRegion INFO: DE result for case ((6,), (0, 1, 2, 3, 4, 5, 7, 15)) with (1032, 7) results (filtered)
2020-09-30 23:38:49,504 SpectraRegion INFO: Created matrices with shape (86, 17900) and (2322, 17900) (target, bg)
2020-09-30 23:38:52,025 SpectraRegion INFO: DE result for case ((6,), (0, 1, 2, 3, 4, 5, 7, 15)) with (17900, 7) results
2020-09-30 23:38:52,026 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:38:52,028 SpectraRegion INFO: DE result for case ((6,), (0, 1, 2, 3, 4, 5, 7, 15)) with (1032, 7) results (filtered)
2020-09-30 23:38:52,082 SpectraRegion INFO: Created matrices with shape (86, 17900) and (2322, 17900) (target, bg)
2020-09-30 23:38:54,614 SpectraRegion INFO: DE data for case: [3]
2020-09-30 23:38:54,614 SpectraRegion INFO: DE data for control: [0, 15, 2, 6, 1, 7, 5, 4]
2020-09-30 23:38:54,615 SpectraRegion INFO: Running [3] against [0, 15, 2, 6, 1, 7, 5, 4]
2020-09-30 23:38:54,615 SpectraRegion INFO: DE result key: ((3,), (0, 1, 2, 4, 5, 6, 7, 15))
2020-09-30 23:38:54,616 SpectraRegion INFO: DE result key already exists
2020-09-30 23:38:54,616 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 6, 7, 15)) with (17900, 7) results
2020-09-30 23:38:54,617 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:38:54,620 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 6, 7, 15)) with (977, 7) results (filtered)
2020-09-30 23:38:54,675 SpectraRegion INFO: Created matrices with shape (99, 17900) and (2309, 17900) (target, bg)
2020-09-30 23:38:57,443 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 6, 7, 15)) with (17900, 7) results
2020-09-30 23:38:57,444 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:38:57,447 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 6, 7, 15)) with (977, 7) results (filtered)
2020-09-30 23:38:57,500 SpectraRegion INFO: Created matrices with shape (99, 17900) and (2309, 17900) (target, bg)
2020-09-30 23:39:00,256 SpectraRegion INFO: DE data for case: [1]
2020-09-30 23:39:00,257 SpectraRegion INFO: DE data for control: [0, 15, 2, 6, 3, 7, 5, 4]
2020-09-30 23:39:00,257 SpectraRegion INFO: Running [1] against [0, 15, 2, 6, 3, 7, 5, 4]
2020-09-30 23:39:00,257 SpectraRegion INFO: DE result key: ((1,), (0, 2, 3, 4, 5, 6, 7, 15))
2020-09-30 23:39:00,258 SpectraRegion INFO: DE result key already exists
2020-09-30 23:39:00,260 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 6, 7, 15)) with (17900, 7) results
2020-09-30 23:39:00,261 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:39:00,263 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 6, 7, 15)) with (1944, 7) results (filtered)
2020-09-30 23:39:00,321 SpectraRegion INFO: Created matrices with shape (61, 17900) and (2347, 17900) (target, bg)
2020-09-30 23:39:06,161 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 6, 7, 15)) with (17900, 7) results
2020-09-30 23:39:06,162 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:39:06,165 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 6, 7, 15)) with (1944, 7) results (filtered)
2020-09-30 23:39:06,217 SpectraRegion INFO: Created matrices with shape (61, 17900) and (2347, 17900) (target, bg)
2020-09-30 23:39:12,057 SpectraRegion INFO: DE data for case: [7]
2020-09-30 23:39:12,058 SpectraRegion INFO: DE data for control: [0, 15, 2, 6, 3, 1, 5, 4]
2020-09-30 23:39:12,059 SpectraRegion INFO: Running [7] against [0, 15, 2, 6, 3, 1, 5, 4]
2020-09-30 23:39:12,059 SpectraRegion INFO: DE result key: ((7,), (0, 1, 2, 3, 4, 5, 6, 15))
2020-09-30 23:39:12,059 SpectraRegion INFO: DE result key already exists
2020-09-30 23:39:12,061 SpectraRegion INFO: DE result for case ((7,), (0, 1, 2, 3, 4, 5, 6, 15)) with (17900, 7) results
2020-09-30 23:39:12,061 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:39:12,064 SpectraRegion INFO: DE result for case ((7,), (0, 1, 2, 3, 4, 5, 6, 15)) with (1882, 7) results (filtered)
2020-09-30 23:39:12,116 SpectraRegion INFO: Created matrices with shape (39, 17900) and (2369, 17900) (target, bg)
2020-09-30 23:39:16,707 SpectraRegion INFO: DE result for case ((7,), (0, 1, 2, 3, 4, 5, 6, 15)) with (17900, 7) results
2020-09-30 23:39:16,708 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:39:16,710 SpectraRegion INFO: DE result for case ((7,), (0, 1, 2, 3, 4, 5, 6, 15)) with (1888, 7) results (filtered)
2020-09-30 23:39:16,762 SpectraRegion INFO: Created matrices with shape (39, 17900) and (2369, 17900) (target, bg)
2020-09-30 23:39:21,385 SpectraRegion INFO: DE data for case: [5]
2020-09-30 23:39:21,386 SpectraRegion INFO: DE data for control: [0, 15, 2, 6, 3, 1, 7, 4]
2020-09-30 23:39:21,387 SpectraRegion INFO: Running [5] against [0, 15, 2, 6, 3, 1, 7, 4]
2020-09-30 23:39:21,388 SpectraRegion INFO: DE result key: ((5,), (0, 1, 2, 3, 4, 6, 7, 15))
2020-09-30 23:39:21,389 SpectraRegion INFO: DE result key already exists
2020-09-30 23:39:21,390 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 6, 7, 15)) with (17900, 7) results
2020-09-30 23:39:21,391 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:39:21,393 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 6, 7, 15)) with (1840, 7) results (filtered)
2020-09-30 23:39:21,447 SpectraRegion INFO: Created matrices with shape (19, 17900) and (2389, 17900) (target, bg)
2020-09-30 23:39:25,444 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 6, 7, 15)) with (17900, 7) results
2020-09-30 23:39:25,444 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:39:25,447 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 6, 7, 15)) with (1874, 7) results (filtered)
2020-09-30 23:39:25,500 SpectraRegion INFO: Created matrices with shape (19, 17900) and (2389, 17900) (target, bg)
2020-09-30 23:39:29,147 SpectraRegion INFO: DE data for case: [4]
2020-09-30 23:39:29,148 SpectraRegion INFO: DE data for control: [0, 15, 2, 6, 3, 1, 7, 5]
2020-09-30 23:39:29,148 SpectraRegion INFO: Running [4] against [0, 15, 2, 6, 3, 1, 7, 5]
2020-09-30 23:39:29,149 SpectraRegion INFO: DE result key: ((4,), (0, 1, 2, 3, 5, 6, 7, 15))
2020-09-30 23:39:29,149 SpectraRegion INFO: DE result key already exists
2020-09-30 23:39:29,151 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 6, 7, 15)) with (17900, 7) results
2020-09-30 23:39:29,151 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:39:29,154 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 6, 7, 15)) with (946, 7) results (filtered)
2020-09-30 23:39:29,207 SpectraRegion INFO: Created matrices with shape (11, 17900) and (2397, 17900) (target, bg)
2020-09-30 23:39:31,549 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 6, 7, 15)) with (17900, 7) results
2020-09-30 23:39:31,550 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:39:31,552 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 6, 7, 15)) with (1065, 7) results (filtered)
2020-09-30 23:39:31,605 SpectraRegion INFO: Created matrices with shape (11, 17900) and (2397, 17900) (target, bg)
2020-09-30 23:39:34,059 SpectraRegion INFO: No cell type info for cluster: 0
2020-09-30 23:39:34,059 SpectraRegion INFO: No cell type info for cluster: 2
2020-09-30 23:39:34,060 SpectraRegion INFO: No cell type info for cluster: 6
2020-09-30 23:39:34,060 SpectraRegion INFO: No cell type info for cluster: 3
2020-09-30 23:39:34,119 SpectraRegion INFO: Cell-type assigned: 1 -> Cardiomyocytes;Heart
2020-09-30 23:39:34,120 SpectraRegion INFO: Cell-type assigned: 2 -> Adipocytes;Connective tissue
2020-09-30 23:39:34,121 SpectraRegion INFO: Cell-type assigned: 4 -> Platelets;Blood
2020-09-30 23:39:34,121 SpectraRegion INFO: Cell-type assigned: 5 -> Monocytes;Immune system
2020-09-30 23:39:34,121 SpectraRegion INFO: Cell-type assigned: 10 -> Basophils;Immune system
2020-09-30 23:39:34,122 SpectraRegion INFO: Cell-type assigned: 11 -> NK cells;Immune system
2020-09-30 23:39:34,122 SpectraRegion INFO: Cell-type assigned: 12 -> Cardiomyocytes;Heart
2020-09-30 23:39:34,123 SpectraRegion INFO: Cell-type assigned: 13 -> Cardiomyocytes;Heart
2020-09-30 23:39:34,133 SpectraRegion INFO: DE data for case: [0]
2020-09-30 23:39:34,134 SpectraRegion INFO: DE data for control: [13, 3, 10, 12, 2, 11, 1, 5, 4]
2020-09-30 23:39:34,135 SpectraRegion INFO: Running [0] against [13, 3, 10, 12, 2, 11, 1, 5, 4]
2020-09-30 23:39:34,135 SpectraRegion INFO: DE result key: ((0,), (1, 2, 3, 4, 5, 10, 11, 12, 13))
2020-09-30 23:39:34,136 SpectraRegion INFO: DE result key already exists
2020-09-30 23:39:34,137 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 23:39:34,138 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:39:34,140 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 10, 11, 12, 13)) with (1491, 7) results (filtered)
2020-09-30 23:39:34,244 SpectraRegion INFO: Created matrices with shape (2218, 17900) and (526, 17900) (target, bg)
2020-09-30 23:39:38,498 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 23:39:38,498 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:39:38,501 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 10, 11, 12, 13)) with (1491, 7) results (filtered)
2020-09-30 23:39:38,569 SpectraRegion INFO: Created matrices with shape (2218, 17900) and (526, 17900) (target, bg)
2020-09-30 23:39:42,710 SpectraRegion INFO: DE data for case: [13]
2020-09-30 23:39:42,711 SpectraRegion INFO: DE data for control: [0, 3, 10, 12, 2, 11, 1, 5, 4]
2020-09-30 23:39:42,712 SpectraRegion INFO: Running [13] against [0, 3, 10, 12, 2, 11, 1, 5, 4]
2020-09-30 23:39:42,712 SpectraRegion INFO: DE result key: ((13,), (0, 1, 2, 3, 4, 5, 10, 11, 12))
2020-09-30 23:39:42,713 SpectraRegion INFO: DE result key already exists
2020-09-30 23:39:42,714 SpectraRegion INFO: DE result for case ((13,), (0, 1, 2, 3, 4, 5, 10, 11, 12)) with (17900, 7) results
2020-09-30 23:39:42,715 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:39:42,717 SpectraRegion INFO: DE result for case ((13,), (0, 1, 2, 3, 4, 5, 10, 11, 12)) with (89, 7) results (filtered)
2020-09-30 23:39:42,779 SpectraRegion INFO: Created matrices with shape (101, 17900) and (2643, 17900) (target, bg)
2020-09-30 23:39:42,987 SpectraRegion INFO: DE result for case ((13,), (0, 1, 2, 3, 4, 5, 10, 11, 12)) with (17900, 7) results
2020-09-30 23:39:42,988 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:39:42,990 SpectraRegion INFO: DE result for case ((13,), (0, 1, 2, 3, 4, 5, 10, 11, 12)) with (89, 7) results (filtered)
2020-09-30 23:39:43,052 SpectraRegion INFO: Created matrices with shape (101, 17900) and (2643, 17900) (target, bg)
2020-09-30 23:39:43,256 SpectraRegion INFO: DE data for case: [3]
2020-09-30 23:39:43,257 SpectraRegion INFO: DE data for control: [0, 13, 10, 12, 2, 11, 1, 5, 4]
2020-09-30 23:39:43,257 SpectraRegion INFO: Running [3] against [0, 13, 10, 12, 2, 11, 1, 5, 4]
2020-09-30 23:39:43,258 SpectraRegion INFO: DE result key: ((3,), (0, 1, 2, 4, 5, 10, 11, 12, 13))
2020-09-30 23:39:43,258 SpectraRegion INFO: DE result key already exists
2020-09-30 23:39:43,260 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 23:39:43,261 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:39:43,263 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 10, 11, 12, 13)) with (1138, 7) results (filtered)
2020-09-30 23:39:43,324 SpectraRegion INFO: Created matrices with shape (102, 17900) and (2642, 17900) (target, bg)
2020-09-30 23:39:46,500 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 23:39:46,500 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:39:46,503 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 10, 11, 12, 13)) with (1138, 7) results (filtered)
2020-09-30 23:39:46,564 SpectraRegion INFO: Created matrices with shape (102, 17900) and (2642, 17900) (target, bg)
2020-09-30 23:39:49,593 SpectraRegion INFO: DE data for case: [10]
2020-09-30 23:39:49,594 SpectraRegion INFO: DE data for control: [0, 13, 3, 12, 2, 11, 1, 5, 4]
2020-09-30 23:39:49,594 SpectraRegion INFO: Running [10] against [0, 13, 3, 12, 2, 11, 1, 5, 4]
2020-09-30 23:39:49,595 SpectraRegion INFO: DE result key: ((10,), (0, 1, 2, 3, 4, 5, 11, 12, 13))
2020-09-30 23:39:49,595 SpectraRegion INFO: DE result key already exists
2020-09-30 23:39:49,597 SpectraRegion INFO: DE result for case ((10,), (0, 1, 2, 3, 4, 5, 11, 12, 13)) with (17900, 7) results
2020-09-30 23:39:49,598 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:39:49,600 SpectraRegion INFO: DE result for case ((10,), (0, 1, 2, 3, 4, 5, 11, 12, 13)) with (868, 7) results (filtered)
2020-09-30 23:39:49,661 SpectraRegion INFO: Created matrices with shape (49, 17900) and (2695, 17900) (target, bg)
2020-09-30 23:39:51,745 SpectraRegion INFO: DE result for case ((10,), (0, 1, 2, 3, 4, 5, 11, 12, 13)) with (17900, 7) results
2020-09-30 23:39:51,746 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:39:51,748 SpectraRegion INFO: DE result for case ((10,), (0, 1, 2, 3, 4, 5, 11, 12, 13)) with (868, 7) results (filtered)
2020-09-30 23:39:51,810 SpectraRegion INFO: Created matrices with shape (49, 17900) and (2695, 17900) (target, bg)
2020-09-30 23:39:53,900 SpectraRegion INFO: DE data for case: [12]
2020-09-30 23:39:53,900 SpectraRegion INFO: DE data for control: [0, 13, 3, 10, 2, 11, 1, 5, 4]
2020-09-30 23:39:53,901 SpectraRegion INFO: Running [12] against [0, 13, 3, 10, 2, 11, 1, 5, 4]
2020-09-30 23:39:53,901 SpectraRegion INFO: DE result key: ((12,), (0, 1, 2, 3, 4, 5, 10, 11, 13))
2020-09-30 23:39:53,902 SpectraRegion INFO: DE result key already exists
2020-09-30 23:39:53,904 SpectraRegion INFO: DE result for case ((12,), (0, 1, 2, 3, 4, 5, 10, 11, 13)) with (17900, 7) results
2020-09-30 23:39:53,905 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:39:53,908 SpectraRegion INFO: DE result for case ((12,), (0, 1, 2, 3, 4, 5, 10, 11, 13)) with (1575, 7) results (filtered)
2020-09-30 23:39:53,969 SpectraRegion INFO: Created matrices with shape (61, 17900) and (2683, 17900) (target, bg)
2020-09-30 23:39:57,577 SpectraRegion INFO: DE result for case ((12,), (0, 1, 2, 3, 4, 5, 10, 11, 13)) with (17900, 7) results
2020-09-30 23:39:57,578 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:39:57,580 SpectraRegion INFO: DE result for case ((12,), (0, 1, 2, 3, 4, 5, 10, 11, 13)) with (1574, 7) results (filtered)
2020-09-30 23:39:57,639 SpectraRegion INFO: Created matrices with shape (61, 17900) and (2683, 17900) (target, bg)
2020-09-30 23:40:01,187 SpectraRegion INFO: DE data for case: [2]
2020-09-30 23:40:01,187 SpectraRegion INFO: DE data for control: [0, 13, 3, 10, 12, 11, 1, 5, 4]
2020-09-30 23:40:01,188 SpectraRegion INFO: Running [2] against [0, 13, 3, 10, 12, 11, 1, 5, 4]
2020-09-30 23:40:01,189 SpectraRegion INFO: DE result key: ((2,), (0, 1, 3, 4, 5, 10, 11, 12, 13))
2020-09-30 23:40:01,189 SpectraRegion INFO: DE result key already exists
2020-09-30 23:40:01,191 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 23:40:01,192 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:40:01,195 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 10, 11, 12, 13)) with (2184, 7) results (filtered)
2020-09-30 23:40:01,254 SpectraRegion INFO: Created matrices with shape (45, 17900) and (2699, 17900) (target, bg)
2020-09-30 23:40:08,343 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 23:40:08,344 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:40:08,346 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 10, 11, 12, 13)) with (2184, 7) results (filtered)
2020-09-30 23:40:08,407 SpectraRegion INFO: Created matrices with shape (45, 17900) and (2699, 17900) (target, bg)
2020-09-30 23:40:15,385 SpectraRegion INFO: DE data for case: [11]
2020-09-30 23:40:15,385 SpectraRegion INFO: DE data for control: [0, 13, 3, 10, 12, 2, 1, 5, 4]
2020-09-30 23:40:15,386 SpectraRegion INFO: Running [11] against [0, 13, 3, 10, 12, 2, 1, 5, 4]
2020-09-30 23:40:15,386 SpectraRegion INFO: DE result key: ((11,), (0, 1, 2, 3, 4, 5, 10, 12, 13))
2020-09-30 23:40:15,387 SpectraRegion INFO: DE result key already exists
2020-09-30 23:40:15,389 SpectraRegion INFO: DE result for case ((11,), (0, 1, 2, 3, 4, 5, 10, 12, 13)) with (17900, 7) results
2020-09-30 23:40:15,390 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:40:15,392 SpectraRegion INFO: DE result for case ((11,), (0, 1, 2, 3, 4, 5, 10, 12, 13)) with (1552, 7) results (filtered)
2020-09-30 23:40:15,452 SpectraRegion INFO: Created matrices with shape (45, 17900) and (2699, 17900) (target, bg)
2020-09-30 23:40:19,566 SpectraRegion INFO: DE result for case ((11,), (0, 1, 2, 3, 4, 5, 10, 12, 13)) with (17900, 7) results
2020-09-30 23:40:19,566 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:40:19,569 SpectraRegion INFO: DE result for case ((11,), (0, 1, 2, 3, 4, 5, 10, 12, 13)) with (1554, 7) results (filtered)
2020-09-30 23:40:19,628 SpectraRegion INFO: Created matrices with shape (45, 17900) and (2699, 17900) (target, bg)
2020-09-30 23:40:23,792 SpectraRegion INFO: DE data for case: [1]
2020-09-30 23:40:23,793 SpectraRegion INFO: DE data for control: [0, 13, 3, 10, 12, 2, 11, 5, 4]
2020-09-30 23:40:23,793 SpectraRegion INFO: Running [1] against [0, 13, 3, 10, 12, 2, 11, 5, 4]
2020-09-30 23:40:23,794 SpectraRegion INFO: DE result key: ((1,), (0, 2, 3, 4, 5, 10, 11, 12, 13))
2020-09-30 23:40:23,794 SpectraRegion INFO: DE result key already exists
2020-09-30 23:40:23,795 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 23:40:23,796 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:40:23,800 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 10, 11, 12, 13)) with (2040, 7) results (filtered)
2020-09-30 23:40:23,860 SpectraRegion INFO: Created matrices with shape (57, 17900) and (2687, 17900) (target, bg)
2020-09-30 23:40:30,028 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 23:40:30,029 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:40:30,032 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 10, 11, 12, 13)) with (2038, 7) results (filtered)
2020-09-30 23:40:30,105 SpectraRegion INFO: Created matrices with shape (57, 17900) and (2687, 17900) (target, bg)
2020-09-30 23:40:36,316 SpectraRegion INFO: DE data for case: [5]
2020-09-30 23:40:36,317 SpectraRegion INFO: DE data for control: [0, 13, 3, 10, 12, 2, 11, 1, 4]
2020-09-30 23:40:36,317 SpectraRegion INFO: Running [5] against [0, 13, 3, 10, 12, 2, 11, 1, 4]
2020-09-30 23:40:36,318 SpectraRegion INFO: DE result key: ((5,), (0, 1, 2, 3, 4, 10, 11, 12, 13))
2020-09-30 23:40:36,318 SpectraRegion INFO: DE result key already exists
2020-09-30 23:40:36,319 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 23:40:36,320 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:40:36,324 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 10, 11, 12, 13)) with (1661, 7) results (filtered)
2020-09-30 23:40:36,393 SpectraRegion INFO: Created matrices with shape (48, 17900) and (2696, 17900) (target, bg)
2020-09-30 23:40:40,526 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 23:40:40,527 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:40:40,530 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 10, 11, 12, 13)) with (1659, 7) results (filtered)
2020-09-30 23:40:40,589 SpectraRegion INFO: Created matrices with shape (48, 17900) and (2696, 17900) (target, bg)
2020-09-30 23:40:44,728 SpectraRegion INFO: DE data for case: [4]
2020-09-30 23:40:44,729 SpectraRegion INFO: DE data for control: [0, 13, 3, 10, 12, 2, 11, 1, 5]
2020-09-30 23:40:44,729 SpectraRegion INFO: Running [4] against [0, 13, 3, 10, 12, 2, 11, 1, 5]
2020-09-30 23:40:44,730 SpectraRegion INFO: DE result key: ((4,), (0, 1, 2, 3, 5, 10, 11, 12, 13))
2020-09-30 23:40:44,730 SpectraRegion INFO: DE result key already exists
2020-09-30 23:40:44,733 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 23:40:44,733 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:40:44,736 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 10, 11, 12, 13)) with (2953, 7) results (filtered)
2020-09-30 23:40:44,795 SpectraRegion INFO: Created matrices with shape (18, 17900) and (2726, 17900) (target, bg)
2020-09-30 23:40:53,414 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-09-30 23:40:53,415 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:40:53,417 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 10, 11, 12, 13)) with (2920, 7) results (filtered)
2020-09-30 23:40:53,477 SpectraRegion INFO: Created matrices with shape (18, 17900) and (2726, 17900) (target, bg)
2020-09-30 23:41:02,695 SpectraRegion INFO: No cell type info for cluster: 0
2020-09-30 23:41:02,696 SpectraRegion INFO: No cell type info for cluster: 3
2020-09-30 23:41:02,705 SpectraRegion INFO: Cell-type assigned: 9 -> Endothelial cells;Vasculature
2020-09-30 23:41:02,706 SpectraRegion INFO: Cell-type assigned: 10 -> Gamma delta T cells;Immune system
2020-09-30 23:41:02,707 SpectraRegion INFO: Cell-type assigned: 11 -> Platelets;Blood
2020-09-30 23:41:02,707 SpectraRegion INFO: Cell-type assigned: 14 -> Adipocytes;Connective tissue
2020-09-30 23:41:02,708 SpectraRegion INFO: Cell-type assigned: 15 -> Cardiomyocytes;Heart
2020-09-30 23:41:02,720 SpectraRegion INFO: DE data for case: [0]
2020-09-30 23:41:02,720 SpectraRegion INFO: DE data for control: [15, 12, 11, 14, 10, 9]
2020-09-30 23:41:02,721 SpectraRegion INFO: Running [0] against [15, 12, 11, 14, 10, 9]
2020-09-30 23:41:02,721 SpectraRegion INFO: DE result key: ((0,), (9, 10, 11, 12, 14, 15))
2020-09-30 23:41:02,722 SpectraRegion INFO: DE result key already exists
2020-09-30 23:41:02,723 SpectraRegion INFO: DE result for case ((0,), (9, 10, 11, 12, 14, 15)) with (17900, 7) results
2020-09-30 23:41:02,723 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:41:02,726 SpectraRegion INFO: DE result for case ((0,), (9, 10, 11, 12, 14, 15)) with (1125, 7) results (filtered)
2020-09-30 23:41:02,838 SpectraRegion INFO: Created matrices with shape (2815, 17900) and (253, 17900) (target, bg)
2020-09-30 23:41:05,162 SpectraRegion INFO: DE result for case ((0,), (9, 10, 11, 12, 14, 15)) with (17900, 7) results
2020-09-30 23:41:05,163 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:41:05,165 SpectraRegion INFO: DE result for case ((0,), (9, 10, 11, 12, 14, 15)) with (1125, 7) results (filtered)
2020-09-30 23:41:05,234 SpectraRegion INFO: Created matrices with shape (2815, 17900) and (253, 17900) (target, bg)
2020-09-30 23:41:07,563 SpectraRegion INFO: DE data for case: [15]
2020-09-30 23:41:07,564 SpectraRegion INFO: DE data for control: [0, 12, 11, 14, 10, 9]
2020-09-30 23:41:07,564 SpectraRegion INFO: Running [15] against [0, 12, 11, 14, 10, 9]
2020-09-30 23:41:07,565 SpectraRegion INFO: DE result key: ((15,), (0, 9, 10, 11, 12, 14))
2020-09-30 23:41:07,565 SpectraRegion INFO: DE result key already exists
2020-09-30 23:41:07,567 SpectraRegion INFO: DE result for case ((15,), (0, 9, 10, 11, 12, 14)) with (17900, 7) results
2020-09-30 23:41:07,568 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:41:07,570 SpectraRegion INFO: DE result for case ((15,), (0, 9, 10, 11, 12, 14)) with (863, 7) results (filtered)
2020-09-30 23:41:07,639 SpectraRegion INFO: Created matrices with shape (98, 17900) and (2970, 17900) (target, bg)
2020-09-30 23:41:09,509 SpectraRegion INFO: DE result for case ((15,), (0, 9, 10, 11, 12, 14)) with (17900, 7) results
2020-09-30 23:41:09,509 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:41:09,512 SpectraRegion INFO: DE result for case ((15,), (0, 9, 10, 11, 12, 14)) with (863, 7) results (filtered)
2020-09-30 23:41:09,580 SpectraRegion INFO: Created matrices with shape (98, 17900) and (2970, 17900) (target, bg)
2020-09-30 23:41:11,447 SpectraRegion INFO: DE data for case: [12]
2020-09-30 23:41:11,448 SpectraRegion INFO: DE data for control: [0, 15, 11, 14, 10, 9]
2020-09-30 23:41:11,448 SpectraRegion INFO: Running [12] against [0, 15, 11, 14, 10, 9]
2020-09-30 23:41:11,449 SpectraRegion INFO: DE result key: ((12,), (0, 9, 10, 11, 14, 15))
2020-09-30 23:41:11,449 SpectraRegion INFO: DE result key already exists
2020-09-30 23:41:11,451 SpectraRegion INFO: DE result for case ((12,), (0, 9, 10, 11, 14, 15)) with (17900, 7) results
2020-09-30 23:41:11,452 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:41:11,455 SpectraRegion INFO: DE result for case ((12,), (0, 9, 10, 11, 14, 15)) with (856, 7) results (filtered)
2020-09-30 23:41:11,522 SpectraRegion INFO: Created matrices with shape (33, 17900) and (3035, 17900) (target, bg)
2020-09-30 23:41:13,438 SpectraRegion INFO: DE result for case ((12,), (0, 9, 10, 11, 14, 15)) with (17900, 7) results
2020-09-30 23:41:13,438 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:41:13,441 SpectraRegion INFO: DE result for case ((12,), (0, 9, 10, 11, 14, 15)) with (855, 7) results (filtered)
2020-09-30 23:41:13,506 SpectraRegion INFO: Created matrices with shape (33, 17900) and (3035, 17900) (target, bg)
2020-09-30 23:41:15,383 SpectraRegion INFO: DE data for case: [11]
2020-09-30 23:41:15,384 SpectraRegion INFO: DE data for control: [0, 15, 12, 14, 10, 9]
2020-09-30 23:41:15,384 SpectraRegion INFO: Running [11] against [0, 15, 12, 14, 10, 9]
2020-09-30 23:41:15,384 SpectraRegion INFO: DE result key: ((11,), (0, 9, 10, 12, 14, 15))
2020-09-30 23:41:15,385 SpectraRegion INFO: DE result key already exists
2020-09-30 23:41:15,388 SpectraRegion INFO: DE result for case ((11,), (0, 9, 10, 12, 14, 15)) with (17900, 7) results
2020-09-30 23:41:15,388 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:41:15,391 SpectraRegion INFO: DE result for case ((11,), (0, 9, 10, 12, 14, 15)) with (2583, 7) results (filtered)
2020-09-30 23:41:15,457 SpectraRegion INFO: Created matrices with shape (24, 17900) and (3044, 17900) (target, bg)
2020-09-30 23:41:23,438 SpectraRegion INFO: DE result for case ((11,), (0, 9, 10, 12, 14, 15)) with (17900, 7) results
2020-09-30 23:41:23,439 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:41:23,441 SpectraRegion INFO: DE result for case ((11,), (0, 9, 10, 12, 14, 15)) with (2542, 7) results (filtered)
2020-09-30 23:41:23,508 SpectraRegion INFO: Created matrices with shape (24, 17900) and (3044, 17900) (target, bg)
2020-09-30 23:41:31,414 SpectraRegion INFO: DE data for case: [14]
2020-09-30 23:41:31,415 SpectraRegion INFO: DE data for control: [0, 15, 12, 11, 10, 9]
2020-09-30 23:41:31,415 SpectraRegion INFO: Running [14] against [0, 15, 12, 11, 10, 9]
2020-09-30 23:41:31,416 SpectraRegion INFO: DE result key: ((14,), (0, 9, 10, 11, 12, 15))
2020-09-30 23:41:31,416 SpectraRegion INFO: DE result key already exists
2020-09-30 23:41:31,418 SpectraRegion INFO: DE result for case ((14,), (0, 9, 10, 11, 12, 15)) with (17900, 7) results
2020-09-30 23:41:31,419 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:41:31,421 SpectraRegion INFO: DE result for case ((14,), (0, 9, 10, 11, 12, 15)) with (2209, 7) results (filtered)
2020-09-30 23:41:31,488 SpectraRegion INFO: Created matrices with shape (28, 17900) and (3040, 17900) (target, bg)
2020-09-30 23:41:37,033 SpectraRegion INFO: DE result for case ((14,), (0, 9, 10, 11, 12, 15)) with (17900, 7) results
2020-09-30 23:41:37,034 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:41:37,036 SpectraRegion INFO: DE result for case ((14,), (0, 9, 10, 11, 12, 15)) with (2219, 7) results (filtered)
2020-09-30 23:41:37,103 SpectraRegion INFO: Created matrices with shape (28, 17900) and (3040, 17900) (target, bg)
2020-09-30 23:41:42,665 SpectraRegion INFO: DE data for case: [10]
2020-09-30 23:41:42,666 SpectraRegion INFO: DE data for control: [0, 15, 12, 11, 14, 9]
2020-09-30 23:41:42,667 SpectraRegion INFO: Running [10] against [0, 15, 12, 11, 14, 9]
2020-09-30 23:41:42,667 SpectraRegion INFO: DE result key: ((10,), (0, 9, 11, 12, 14, 15))
2020-09-30 23:41:42,668 SpectraRegion INFO: DE result key already exists
2020-09-30 23:41:42,669 SpectraRegion INFO: DE result for case ((10,), (0, 9, 11, 12, 14, 15)) with (17900, 7) results
2020-09-30 23:41:42,669 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:41:42,672 SpectraRegion INFO: DE result for case ((10,), (0, 9, 11, 12, 14, 15)) with (1799, 7) results (filtered)
2020-09-30 23:41:42,742 SpectraRegion INFO: Created matrices with shape (36, 17900) and (3032, 17900) (target, bg)
2020-09-30 23:41:46,596 SpectraRegion INFO: DE result for case ((10,), (0, 9, 11, 12, 14, 15)) with (17900, 7) results
2020-09-30 23:41:46,596 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:41:46,599 SpectraRegion INFO: DE result for case ((10,), (0, 9, 11, 12, 14, 15)) with (1807, 7) results (filtered)
2020-09-30 23:41:46,665 SpectraRegion INFO: Created matrices with shape (36, 17900) and (3032, 17900) (target, bg)
2020-09-30 23:41:50,500 SpectraRegion INFO: DE data for case: [9]
2020-09-30 23:41:50,500 SpectraRegion INFO: DE data for control: [0, 15, 12, 11, 14, 10]
2020-09-30 23:41:50,501 SpectraRegion INFO: Running [9] against [0, 15, 12, 11, 14, 10]
2020-09-30 23:41:50,501 SpectraRegion INFO: DE result key: ((9,), (0, 10, 11, 12, 14, 15))
2020-09-30 23:41:50,502 SpectraRegion INFO: DE result key already exists
2020-09-30 23:41:50,504 SpectraRegion INFO: DE result for case ((9,), (0, 10, 11, 12, 14, 15)) with (17900, 7) results
2020-09-30 23:41:50,505 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:41:50,508 SpectraRegion INFO: DE result for case ((9,), (0, 10, 11, 12, 14, 15)) with (1932, 7) results (filtered)
2020-09-30 23:41:50,574 SpectraRegion INFO: Created matrices with shape (34, 17900) and (3034, 17900) (target, bg)
2020-09-30 23:41:55,153 SpectraRegion INFO: DE result for case ((9,), (0, 10, 11, 12, 14, 15)) with (17900, 7) results
2020-09-30 23:41:55,154 SpectraRegion INFO: DE result logFC inversed
2020-09-30 23:41:55,156 SpectraRegion INFO: DE result for case ((9,), (0, 10, 11, 12, 14, 15)) with (1937, 7) results (filtered)
2020-09-30 23:41:55,222 SpectraRegion INFO: Created matrices with shape (34, 17900) and (3034, 17900) (target, bg)
2020-09-30 23:41:59,909 SpectraRegion INFO: No cell type info for cluster: 0
2020-09-30 23:41:59,909 SpectraRegion INFO: No cell type info for cluster: 12
! python3 analyseMarkers.py --organs "Connective tissue" "Vasculature" "Heart" "Skeletal Muscle" "Smooth muscle" "Immune system" "Blood" "Epithelium" --pvaladj qvalue --markers deresults/marker_genes_region_0.tsv -n 2
! python3 analyseMarkers.py --organs "Connective tissue" "Vasculature" "Heart" "Skeletal Muscle" "Smooth muscle" "Immune system" "Blood" "Epithelium" --pvaladj qvalue --markers deresults/marker_genes_region_1.tsv -n 2
! python3 analyseMarkers.py --organs "Connective tissue" "Vasculature" "Heart" "Skeletal Muscle" "Smooth muscle" "Immune system" "Blood" "Epithelium" --pvaladj qvalue --markers deresults/marker_genes_region_4.tsv -n 2
! python3 analyseMarkers.py --organs "Connective tissue" "Vasculature" "Heart" "Skeletal Muscle" "Smooth muscle" "Immune system" "Blood" "Epithelium" --pvaladj qvalue --markers deresults/marker_genes_region_5.tsv -n 2
From these cell type predictions it can be seen that mostly atherosclerosis relates cell types are showing up.
Creating a CombinedSpectra¶
slided_0.name = "slided_0"
slided_1.name = "slided_1"
slided_4.name = "slided_4"
slided_5.name = "slided_5"
#slided_0 = SpectraRegion.from_pickle("slideD_region_0.pickle")
#slided_1 = SpectraRegion.from_pickle("slideD_region_1.pickle")
#slided_4 = SpectraRegion.from_pickle("slideD_region_4.pickle")
#slided_5 = SpectraRegion.from_pickle("slideD_region_5pickle")
Of course a SpectraRegion can also be re-processed to yield a more realistic clustering.
slided_1.segment(method="WPGMA", number_of_regions=15)
slided_1.plot_segments()
slided_1.filter_clusters(method='remove_singleton')
slided_1.filter_clusters(method='merge_background')
slided_1.filter_clusters(method='remove_islands')
slided_1.filter_clusters(method='remove_islands', minIslandSize=15)
slided_1.plot_segments()
mask = slided_1.segmented == 8
mask[0:22,: ] = False
mask[:,0:22 ] = False
slided_1.segmented[mask] = 15
slided_1.plot_segments()
slided_1.plot_segments(highlight=[15])
slided_5.segment(method="WPGMA", number_of_regions=15)
slided_5.plot_segments()
slided_5.filter_clusters(method='remove_singleton')
slided_5.filter_clusters(method='merge_background')
slided_5.filter_clusters(method='remove_islands')
slided_5.filter_clusters(method='remove_islands', minIslandSize=15)
slided_5.plot_segments()
slided_0.to_pickle("deresults/slideD_region_0.pickle")
slided_1.to_pickle("deresults/slideD_region_1.pickle")
slided_4.to_pickle("deresults/slideD_region_4.pickle")
slided_5.to_pickle("deresults/slideD_region_5.pickle")
slided_0.to_aorta3d("./aorta3d/slided/", "slided", 0, protWeights=pw, ctpred="deresults/marker_genes_region_0.pred.tsv")
slided_1.to_aorta3d("./aorta3d/slided/", "slided", 1, protWeights=pw, ctpred="deresults/marker_genes_region_1.pred.tsv")
slided_4.to_aorta3d("./aorta3d/slided/", "slided", 4, protWeights=pw, ctpred="deresults/marker_genes_region_4.pred.tsv")
slided_5.to_aorta3d("./aorta3d/slided/", "slided", 5, protWeights=pw, ctpred="deresults/marker_genes_region_5.pred.tsv")
2020-10-01 18:53:10,097 SpectraRegion INFO: Cell-type assigned: 1 -> Monocytes;Immune system
INFO:SpectraRegion:Cell-type assigned: 1 -> Monocytes;Immune system
2020-10-01 18:53:10,098 SpectraRegion INFO: Cell-type assigned: 2 -> Megakaryocytes;Immune system
INFO:SpectraRegion:Cell-type assigned: 2 -> Megakaryocytes;Immune system
2020-10-01 18:53:10,099 SpectraRegion INFO: Cell-type assigned: 4 -> Mast cells;Immune system
INFO:SpectraRegion:Cell-type assigned: 4 -> Mast cells;Immune system
2020-10-01 18:53:10,100 SpectraRegion INFO: Cell-type assigned: 5 -> Monocytes;Immune system
INFO:SpectraRegion:Cell-type assigned: 5 -> Monocytes;Immune system
2020-10-01 18:53:10,101 SpectraRegion INFO: Cell-type assigned: 6 -> Monocytes;Immune system
INFO:SpectraRegion:Cell-type assigned: 6 -> Monocytes;Immune system
2020-10-01 18:53:10,102 SpectraRegion INFO: Cell-type assigned: 7 -> Mast cells;Immune system
INFO:SpectraRegion:Cell-type assigned: 7 -> Mast cells;Immune system
2020-10-01 18:53:10,103 SpectraRegion INFO: Cell-type assigned: 8 -> Mast cells;Immune system
INFO:SpectraRegion:Cell-type assigned: 8 -> Mast cells;Immune system
2020-10-01 18:53:10,104 SpectraRegion INFO: Cell-type assigned: 9 -> Smooth muscle cells;Smooth muscle
INFO:SpectraRegion:Cell-type assigned: 9 -> Smooth muscle cells;Smooth muscle
2020-10-01 18:53:10,109 SpectraRegion INFO: Segment Image: ./aorta3d/slided/slided.0.clustering.png
INFO:SpectraRegion:Segment Image: ./aorta3d/slided/slided.0.clustering.png
2020-10-01 18:53:10,114 SpectraRegion INFO: Segment Matrix: /mnt/f/dev/git/pyIMS/examples/aorta3d/slided/slided.0.matrix.npy
INFO:SpectraRegion:Segment Matrix: /mnt/f/dev/git/pyIMS/examples/aorta3d/slided/slided.0.matrix.npy
2020-10-01 18:53:10,119 SpectraRegion INFO: Starting Marker Proteins Analysis
INFO:SpectraRegion:Starting Marker Proteins Analysis
2020-10-01 18:53:10,122 SpectraRegion INFO: DE data for case: [0]
INFO:SpectraRegion:DE data for case: [0]
2020-10-01 18:53:10,123 SpectraRegion INFO: DE data for control: [5, 7, 9, 3, 8, 2, 4, 6, 1]
INFO:SpectraRegion:DE data for control: [5, 7, 9, 3, 8, 2, 4, 6, 1]
2020-10-01 18:53:10,124 SpectraRegion INFO: Running [0] against [5, 7, 9, 3, 8, 2, 4, 6, 1]
INFO:SpectraRegion:Running [0] against [5, 7, 9, 3, 8, 2, 4, 6, 1]
2020-10-01 18:53:10,125 SpectraRegion INFO: DE result key: ((0,), (1, 2, 3, 4, 5, 6, 7, 8, 9))
INFO:SpectraRegion:DE result key: ((0,), (1, 2, 3, 4, 5, 6, 7, 8, 9))
2020-10-01 18:53:10,126 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:53:10,130 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((0,), (1, 2, 3, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-10-01 18:53:10,131 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:53:10,132 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:53:10,136 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 6, 7, 8, 9)) with (1307, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((0,), (1, 2, 3, 4, 5, 6, 7, 8, 9)) with (1307, 7) results (filtered)
2020-10-01 18:53:10,275 SpectraRegion INFO: Created matrices with shape (1930, 17900) and (725, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (1930, 17900) and (725, 17900) (target, bg)
2020-10-01 18:53:14,664 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((0,), (1, 2, 3, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-10-01 18:53:14,665 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:53:14,666 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:53:14,670 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 6, 7, 8, 9)) with (1307, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((0,), (1, 2, 3, 4, 5, 6, 7, 8, 9)) with (1307, 7) results (filtered)
2020-10-01 18:53:14,738 SpectraRegion INFO: Created matrices with shape (1930, 17900) and (725, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (1930, 17900) and (725, 17900) (target, bg)
2020-10-01 18:53:19,139 SpectraRegion INFO: DE data for case: [5]
INFO:SpectraRegion:DE data for case: [5]
2020-10-01 18:53:19,141 SpectraRegion INFO: DE data for control: [0, 7, 9, 3, 8, 2, 4, 6, 1]
INFO:SpectraRegion:DE data for control: [0, 7, 9, 3, 8, 2, 4, 6, 1]
2020-10-01 18:53:19,141 SpectraRegion INFO: Running [5] against [0, 7, 9, 3, 8, 2, 4, 6, 1]
INFO:SpectraRegion:Running [5] against [0, 7, 9, 3, 8, 2, 4, 6, 1]
2020-10-01 18:53:19,143 SpectraRegion INFO: DE result key: ((5,), (0, 1, 2, 3, 4, 6, 7, 8, 9))
INFO:SpectraRegion:DE result key: ((5,), (0, 1, 2, 3, 4, 6, 7, 8, 9))
2020-10-01 18:53:19,144 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:53:19,147 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 6, 7, 8, 9)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((5,), (0, 1, 2, 3, 4, 6, 7, 8, 9)) with (17900, 7) results
2020-10-01 18:53:19,148 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:53:19,149 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:53:19,153 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 6, 7, 8, 9)) with (450, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((5,), (0, 1, 2, 3, 4, 6, 7, 8, 9)) with (450, 7) results (filtered)
2020-10-01 18:53:19,218 SpectraRegion INFO: Created matrices with shape (100, 17900) and (2555, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (100, 17900) and (2555, 17900) (target, bg)
2020-10-01 18:53:21,028 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 6, 7, 8, 9)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((5,), (0, 1, 2, 3, 4, 6, 7, 8, 9)) with (17900, 7) results
2020-10-01 18:53:21,029 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:53:21,030 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:53:21,035 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 6, 7, 8, 9)) with (450, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((5,), (0, 1, 2, 3, 4, 6, 7, 8, 9)) with (450, 7) results (filtered)
2020-10-01 18:53:21,096 SpectraRegion INFO: Created matrices with shape (100, 17900) and (2555, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (100, 17900) and (2555, 17900) (target, bg)
2020-10-01 18:53:22,886 SpectraRegion INFO: DE data for case: [7]
INFO:SpectraRegion:DE data for case: [7]
2020-10-01 18:53:22,887 SpectraRegion INFO: DE data for control: [0, 5, 9, 3, 8, 2, 4, 6, 1]
INFO:SpectraRegion:DE data for control: [0, 5, 9, 3, 8, 2, 4, 6, 1]
2020-10-01 18:53:22,888 SpectraRegion INFO: Running [7] against [0, 5, 9, 3, 8, 2, 4, 6, 1]
INFO:SpectraRegion:Running [7] against [0, 5, 9, 3, 8, 2, 4, 6, 1]
2020-10-01 18:53:22,888 SpectraRegion INFO: DE result key: ((7,), (0, 1, 2, 3, 4, 5, 6, 8, 9))
INFO:SpectraRegion:DE result key: ((7,), (0, 1, 2, 3, 4, 5, 6, 8, 9))
2020-10-01 18:53:22,889 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:53:22,892 SpectraRegion INFO: DE result for case ((7,), (0, 1, 2, 3, 4, 5, 6, 8, 9)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((7,), (0, 1, 2, 3, 4, 5, 6, 8, 9)) with (17900, 7) results
2020-10-01 18:53:22,894 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:53:22,895 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:53:22,900 SpectraRegion INFO: DE result for case ((7,), (0, 1, 2, 3, 4, 5, 6, 8, 9)) with (680, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((7,), (0, 1, 2, 3, 4, 5, 6, 8, 9)) with (680, 7) results (filtered)
2020-10-01 18:53:22,966 SpectraRegion INFO: Created matrices with shape (161, 17900) and (2494, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (161, 17900) and (2494, 17900) (target, bg)
2020-10-01 18:53:25,372 SpectraRegion INFO: DE result for case ((7,), (0, 1, 2, 3, 4, 5, 6, 8, 9)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((7,), (0, 1, 2, 3, 4, 5, 6, 8, 9)) with (17900, 7) results
2020-10-01 18:53:25,373 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:53:25,374 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:53:25,377 SpectraRegion INFO: DE result for case ((7,), (0, 1, 2, 3, 4, 5, 6, 8, 9)) with (680, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((7,), (0, 1, 2, 3, 4, 5, 6, 8, 9)) with (680, 7) results (filtered)
2020-10-01 18:53:25,438 SpectraRegion INFO: Created matrices with shape (161, 17900) and (2494, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (161, 17900) and (2494, 17900) (target, bg)
2020-10-01 18:53:27,830 SpectraRegion INFO: DE data for case: [9]
INFO:SpectraRegion:DE data for case: [9]
2020-10-01 18:53:27,831 SpectraRegion INFO: DE data for control: [0, 5, 7, 3, 8, 2, 4, 6, 1]
INFO:SpectraRegion:DE data for control: [0, 5, 7, 3, 8, 2, 4, 6, 1]
2020-10-01 18:53:27,832 SpectraRegion INFO: Running [9] against [0, 5, 7, 3, 8, 2, 4, 6, 1]
INFO:SpectraRegion:Running [9] against [0, 5, 7, 3, 8, 2, 4, 6, 1]
2020-10-01 18:53:27,833 SpectraRegion INFO: DE result key: ((9,), (0, 1, 2, 3, 4, 5, 6, 7, 8))
INFO:SpectraRegion:DE result key: ((9,), (0, 1, 2, 3, 4, 5, 6, 7, 8))
2020-10-01 18:53:27,834 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:53:27,837 SpectraRegion INFO: DE result for case ((9,), (0, 1, 2, 3, 4, 5, 6, 7, 8)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((9,), (0, 1, 2, 3, 4, 5, 6, 7, 8)) with (17900, 7) results
2020-10-01 18:53:27,838 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:53:27,838 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:53:27,842 SpectraRegion INFO: DE result for case ((9,), (0, 1, 2, 3, 4, 5, 6, 7, 8)) with (88, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((9,), (0, 1, 2, 3, 4, 5, 6, 7, 8)) with (88, 7) results (filtered)
2020-10-01 18:53:27,909 SpectraRegion INFO: Created matrices with shape (89, 17900) and (2566, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (89, 17900) and (2566, 17900) (target, bg)
2020-10-01 18:53:28,025 SpectraRegion INFO: DE result for case ((9,), (0, 1, 2, 3, 4, 5, 6, 7, 8)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((9,), (0, 1, 2, 3, 4, 5, 6, 7, 8)) with (17900, 7) results
2020-10-01 18:53:28,027 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:53:28,028 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:53:28,032 SpectraRegion INFO: DE result for case ((9,), (0, 1, 2, 3, 4, 5, 6, 7, 8)) with (65, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((9,), (0, 1, 2, 3, 4, 5, 6, 7, 8)) with (65, 7) results (filtered)
2020-10-01 18:53:28,098 SpectraRegion INFO: Created matrices with shape (89, 17900) and (2566, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (89, 17900) and (2566, 17900) (target, bg)
2020-10-01 18:53:28,180 SpectraRegion INFO: DE data for case: [3]
INFO:SpectraRegion:DE data for case: [3]
2020-10-01 18:53:28,181 SpectraRegion INFO: DE data for control: [0, 5, 7, 9, 8, 2, 4, 6, 1]
INFO:SpectraRegion:DE data for control: [0, 5, 7, 9, 8, 2, 4, 6, 1]
2020-10-01 18:53:28,182 SpectraRegion INFO: Running [3] against [0, 5, 7, 9, 8, 2, 4, 6, 1]
INFO:SpectraRegion:Running [3] against [0, 5, 7, 9, 8, 2, 4, 6, 1]
2020-10-01 18:53:28,183 SpectraRegion INFO: DE result key: ((3,), (0, 1, 2, 4, 5, 6, 7, 8, 9))
INFO:SpectraRegion:DE result key: ((3,), (0, 1, 2, 4, 5, 6, 7, 8, 9))
2020-10-01 18:53:28,184 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:53:28,188 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((3,), (0, 1, 2, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-10-01 18:53:28,189 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:53:28,190 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:53:28,195 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 6, 7, 8, 9)) with (1263, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((3,), (0, 1, 2, 4, 5, 6, 7, 8, 9)) with (1263, 7) results (filtered)
2020-10-01 18:53:28,261 SpectraRegion INFO: Created matrices with shape (51, 17900) and (2604, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (51, 17900) and (2604, 17900) (target, bg)
2020-10-01 18:53:32,627 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((3,), (0, 1, 2, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-10-01 18:53:32,628 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:53:32,630 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:53:32,633 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 6, 7, 8, 9)) with (1261, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((3,), (0, 1, 2, 4, 5, 6, 7, 8, 9)) with (1261, 7) results (filtered)
2020-10-01 18:53:32,695 SpectraRegion INFO: Created matrices with shape (51, 17900) and (2604, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (51, 17900) and (2604, 17900) (target, bg)
2020-10-01 18:53:37,065 SpectraRegion INFO: DE data for case: [8]
INFO:SpectraRegion:DE data for case: [8]
2020-10-01 18:53:37,066 SpectraRegion INFO: DE data for control: [0, 5, 7, 9, 3, 2, 4, 6, 1]
INFO:SpectraRegion:DE data for control: [0, 5, 7, 9, 3, 2, 4, 6, 1]
2020-10-01 18:53:37,067 SpectraRegion INFO: Running [8] against [0, 5, 7, 9, 3, 2, 4, 6, 1]
INFO:SpectraRegion:Running [8] against [0, 5, 7, 9, 3, 2, 4, 6, 1]
2020-10-01 18:53:37,068 SpectraRegion INFO: DE result key: ((8,), (0, 1, 2, 3, 4, 5, 6, 7, 9))
INFO:SpectraRegion:DE result key: ((8,), (0, 1, 2, 3, 4, 5, 6, 7, 9))
2020-10-01 18:53:37,069 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:53:37,072 SpectraRegion INFO: DE result for case ((8,), (0, 1, 2, 3, 4, 5, 6, 7, 9)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((8,), (0, 1, 2, 3, 4, 5, 6, 7, 9)) with (17900, 7) results
2020-10-01 18:53:37,073 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:53:37,074 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:53:37,078 SpectraRegion INFO: DE result for case ((8,), (0, 1, 2, 3, 4, 5, 6, 7, 9)) with (253, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((8,), (0, 1, 2, 3, 4, 5, 6, 7, 9)) with (253, 7) results (filtered)
2020-10-01 18:53:37,141 SpectraRegion INFO: Created matrices with shape (55, 17900) and (2600, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (55, 17900) and (2600, 17900) (target, bg)
2020-10-01 18:53:37,717 SpectraRegion INFO: DE result for case ((8,), (0, 1, 2, 3, 4, 5, 6, 7, 9)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((8,), (0, 1, 2, 3, 4, 5, 6, 7, 9)) with (17900, 7) results
2020-10-01 18:53:37,718 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:53:37,719 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:53:37,723 SpectraRegion INFO: DE result for case ((8,), (0, 1, 2, 3, 4, 5, 6, 7, 9)) with (250, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((8,), (0, 1, 2, 3, 4, 5, 6, 7, 9)) with (250, 7) results (filtered)
2020-10-01 18:53:37,785 SpectraRegion INFO: Created matrices with shape (55, 17900) and (2600, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (55, 17900) and (2600, 17900) (target, bg)
2020-10-01 18:53:38,368 SpectraRegion INFO: DE data for case: [2]
INFO:SpectraRegion:DE data for case: [2]
2020-10-01 18:53:38,370 SpectraRegion INFO: DE data for control: [0, 5, 7, 9, 3, 8, 4, 6, 1]
INFO:SpectraRegion:DE data for control: [0, 5, 7, 9, 3, 8, 4, 6, 1]
2020-10-01 18:53:38,370 SpectraRegion INFO: Running [2] against [0, 5, 7, 9, 3, 8, 4, 6, 1]
INFO:SpectraRegion:Running [2] against [0, 5, 7, 9, 3, 8, 4, 6, 1]
2020-10-01 18:53:38,371 SpectraRegion INFO: DE result key: ((2,), (0, 1, 3, 4, 5, 6, 7, 8, 9))
INFO:SpectraRegion:DE result key: ((2,), (0, 1, 3, 4, 5, 6, 7, 8, 9))
2020-10-01 18:53:38,372 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:53:38,375 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((2,), (0, 1, 3, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-10-01 18:53:38,376 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:53:38,377 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:53:38,382 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 6, 7, 8, 9)) with (1796, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((2,), (0, 1, 3, 4, 5, 6, 7, 8, 9)) with (1796, 7) results (filtered)
2020-10-01 18:53:38,445 SpectraRegion INFO: Created matrices with shape (150, 17900) and (2505, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (150, 17900) and (2505, 17900) (target, bg)
2020-10-01 18:53:45,157 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((2,), (0, 1, 3, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-10-01 18:53:45,158 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:53:45,159 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:53:45,163 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 6, 7, 8, 9)) with (1796, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((2,), (0, 1, 3, 4, 5, 6, 7, 8, 9)) with (1796, 7) results (filtered)
2020-10-01 18:53:45,229 SpectraRegion INFO: Created matrices with shape (150, 17900) and (2505, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (150, 17900) and (2505, 17900) (target, bg)
2020-10-01 18:53:51,985 SpectraRegion INFO: DE data for case: [4]
INFO:SpectraRegion:DE data for case: [4]
2020-10-01 18:53:51,986 SpectraRegion INFO: DE data for control: [0, 5, 7, 9, 3, 8, 2, 6, 1]
INFO:SpectraRegion:DE data for control: [0, 5, 7, 9, 3, 8, 2, 6, 1]
2020-10-01 18:53:51,987 SpectraRegion INFO: Running [4] against [0, 5, 7, 9, 3, 8, 2, 6, 1]
INFO:SpectraRegion:Running [4] against [0, 5, 7, 9, 3, 8, 2, 6, 1]
2020-10-01 18:53:51,988 SpectraRegion INFO: DE result key: ((4,), (0, 1, 2, 3, 5, 6, 7, 8, 9))
INFO:SpectraRegion:DE result key: ((4,), (0, 1, 2, 3, 5, 6, 7, 8, 9))
2020-10-01 18:53:51,989 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:53:51,992 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 6, 7, 8, 9)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((4,), (0, 1, 2, 3, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-10-01 18:53:51,993 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:53:51,996 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:53:52,000 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 6, 7, 8, 9)) with (1742, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((4,), (0, 1, 2, 3, 5, 6, 7, 8, 9)) with (1742, 7) results (filtered)
2020-10-01 18:53:52,066 SpectraRegion INFO: Created matrices with shape (70, 17900) and (2585, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (70, 17900) and (2585, 17900) (target, bg)
2020-10-01 18:53:56,828 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 6, 7, 8, 9)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((4,), (0, 1, 2, 3, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-10-01 18:53:56,829 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:53:56,830 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:53:56,835 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 6, 7, 8, 9)) with (1742, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((4,), (0, 1, 2, 3, 5, 6, 7, 8, 9)) with (1742, 7) results (filtered)
2020-10-01 18:53:56,896 SpectraRegion INFO: Created matrices with shape (70, 17900) and (2585, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (70, 17900) and (2585, 17900) (target, bg)
2020-10-01 18:54:01,662 SpectraRegion INFO: DE data for case: [6]
INFO:SpectraRegion:DE data for case: [6]
2020-10-01 18:54:01,664 SpectraRegion INFO: DE data for control: [0, 5, 7, 9, 3, 8, 2, 4, 1]
INFO:SpectraRegion:DE data for control: [0, 5, 7, 9, 3, 8, 2, 4, 1]
2020-10-01 18:54:01,665 SpectraRegion INFO: Running [6] against [0, 5, 7, 9, 3, 8, 2, 4, 1]
INFO:SpectraRegion:Running [6] against [0, 5, 7, 9, 3, 8, 2, 4, 1]
2020-10-01 18:54:01,666 SpectraRegion INFO: DE result key: ((6,), (0, 1, 2, 3, 4, 5, 7, 8, 9))
INFO:SpectraRegion:DE result key: ((6,), (0, 1, 2, 3, 4, 5, 7, 8, 9))
2020-10-01 18:54:01,666 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:54:01,669 SpectraRegion INFO: DE result for case ((6,), (0, 1, 2, 3, 4, 5, 7, 8, 9)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((6,), (0, 1, 2, 3, 4, 5, 7, 8, 9)) with (17900, 7) results
2020-10-01 18:54:01,670 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:54:01,671 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:54:01,676 SpectraRegion INFO: DE result for case ((6,), (0, 1, 2, 3, 4, 5, 7, 8, 9)) with (2053, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((6,), (0, 1, 2, 3, 4, 5, 7, 8, 9)) with (2053, 7) results (filtered)
2020-10-01 18:54:01,741 SpectraRegion INFO: Created matrices with shape (27, 17900) and (2628, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (27, 17900) and (2628, 17900) (target, bg)
2020-10-01 18:54:08,808 SpectraRegion INFO: DE result for case ((6,), (0, 1, 2, 3, 4, 5, 7, 8, 9)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((6,), (0, 1, 2, 3, 4, 5, 7, 8, 9)) with (17900, 7) results
2020-10-01 18:54:08,809 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:54:08,810 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:54:08,815 SpectraRegion INFO: DE result for case ((6,), (0, 1, 2, 3, 4, 5, 7, 8, 9)) with (2058, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((6,), (0, 1, 2, 3, 4, 5, 7, 8, 9)) with (2058, 7) results (filtered)
2020-10-01 18:54:08,878 SpectraRegion INFO: Created matrices with shape (27, 17900) and (2628, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (27, 17900) and (2628, 17900) (target, bg)
2020-10-01 18:54:15,921 SpectraRegion INFO: DE data for case: [1]
INFO:SpectraRegion:DE data for case: [1]
2020-10-01 18:54:15,922 SpectraRegion INFO: DE data for control: [0, 5, 7, 9, 3, 8, 2, 4, 6]
INFO:SpectraRegion:DE data for control: [0, 5, 7, 9, 3, 8, 2, 4, 6]
2020-10-01 18:54:15,923 SpectraRegion INFO: Running [1] against [0, 5, 7, 9, 3, 8, 2, 4, 6]
INFO:SpectraRegion:Running [1] against [0, 5, 7, 9, 3, 8, 2, 4, 6]
2020-10-01 18:54:15,924 SpectraRegion INFO: DE result key: ((1,), (0, 2, 3, 4, 5, 6, 7, 8, 9))
INFO:SpectraRegion:DE result key: ((1,), (0, 2, 3, 4, 5, 6, 7, 8, 9))
2020-10-01 18:54:15,924 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:54:15,927 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((1,), (0, 2, 3, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-10-01 18:54:15,929 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:54:15,930 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:54:15,934 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 6, 7, 8, 9)) with (3036, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((1,), (0, 2, 3, 4, 5, 6, 7, 8, 9)) with (3036, 7) results (filtered)
2020-10-01 18:54:15,996 SpectraRegion INFO: Created matrices with shape (22, 17900) and (2633, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (22, 17900) and (2633, 17900) (target, bg)
2020-10-01 18:54:27,189 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((1,), (0, 2, 3, 4, 5, 6, 7, 8, 9)) with (17900, 7) results
2020-10-01 18:54:27,191 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:54:27,192 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:54:27,196 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 6, 7, 8, 9)) with (3039, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((1,), (0, 2, 3, 4, 5, 6, 7, 8, 9)) with (3039, 7) results (filtered)
2020-10-01 18:54:27,258 SpectraRegion INFO: Created matrices with shape (22, 17900) and (2633, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (22, 17900) and (2633, 17900) (target, bg)
2020-10-01 18:54:38,558 SpectraRegion INFO: No cell type info for cluster: '0'
INFO:SpectraRegion:No cell type info for cluster: '0'
2020-10-01 18:54:38,560 SpectraRegion INFO: No cell type info for cluster: '3'
INFO:SpectraRegion:No cell type info for cluster: '3'
2020-10-01 18:54:38,573 SpectraRegion INFO: Cell-type assigned: 1 -> Megakaryocytes;Immune system
INFO:SpectraRegion:Cell-type assigned: 1 -> Megakaryocytes;Immune system
2020-10-01 18:54:38,575 SpectraRegion INFO: Cell-type assigned: 4 -> B cells;Immune system
INFO:SpectraRegion:Cell-type assigned: 4 -> B cells;Immune system
2020-10-01 18:54:38,577 SpectraRegion INFO: Cell-type assigned: 5 -> Basophils;Immune system
INFO:SpectraRegion:Cell-type assigned: 5 -> Basophils;Immune system
2020-10-01 18:54:38,578 SpectraRegion INFO: Cell-type assigned: 7 -> Adipocytes;Connective tissue
INFO:SpectraRegion:Cell-type assigned: 7 -> Adipocytes;Connective tissue
2020-10-01 18:54:38,580 SpectraRegion INFO: Cell-type assigned: 15 -> Cardiomyocytes;Heart
INFO:SpectraRegion:Cell-type assigned: 15 -> Cardiomyocytes;Heart
2020-10-01 18:54:38,588 SpectraRegion INFO: Segment Image: ./aorta3d/slided/slided.1.clustering.png
INFO:SpectraRegion:Segment Image: ./aorta3d/slided/slided.1.clustering.png
2020-10-01 18:54:38,596 SpectraRegion INFO: Segment Matrix: /mnt/f/dev/git/pyIMS/examples/aorta3d/slided/slided.1.matrix.npy
INFO:SpectraRegion:Segment Matrix: /mnt/f/dev/git/pyIMS/examples/aorta3d/slided/slided.1.matrix.npy
2020-10-01 18:54:38,602 SpectraRegion INFO: Starting Marker Proteins Analysis
INFO:SpectraRegion:Starting Marker Proteins Analysis
2020-10-01 18:54:38,606 SpectraRegion INFO: DE data for case: [0]
INFO:SpectraRegion:DE data for case: [0]
2020-10-01 18:54:38,608 SpectraRegion INFO: DE data for control: [15, 2, 6, 3, 1, 7, 5, 4]
INFO:SpectraRegion:DE data for control: [15, 2, 6, 3, 1, 7, 5, 4]
2020-10-01 18:54:38,610 SpectraRegion INFO: Running [0] against [15, 2, 6, 3, 1, 7, 5, 4]
INFO:SpectraRegion:Running [0] against [15, 2, 6, 3, 1, 7, 5, 4]
2020-10-01 18:54:38,611 SpectraRegion INFO: DE result key: ((0,), (1, 2, 3, 4, 5, 6, 7, 15))
INFO:SpectraRegion:DE result key: ((0,), (1, 2, 3, 4, 5, 6, 7, 15))
2020-10-01 18:54:38,612 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:54:38,616 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 6, 7, 15)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((0,), (1, 2, 3, 4, 5, 6, 7, 15)) with (17900, 7) results
2020-10-01 18:54:38,617 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:54:38,618 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:54:38,622 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 6, 7, 15)) with (1086, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((0,), (1, 2, 3, 4, 5, 6, 7, 15)) with (1086, 7) results (filtered)
2020-10-01 18:54:38,733 SpectraRegion INFO: Created matrices with shape (1923, 17900) and (485, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (1923, 17900) and (485, 17900) (target, bg)
2020-10-01 18:54:42,214 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 6, 7, 15)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((0,), (1, 2, 3, 4, 5, 6, 7, 15)) with (17900, 7) results
2020-10-01 18:54:42,215 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:54:42,217 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:54:42,221 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 6, 7, 15)) with (1086, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((0,), (1, 2, 3, 4, 5, 6, 7, 15)) with (1086, 7) results (filtered)
2020-10-01 18:54:42,280 SpectraRegion INFO: Created matrices with shape (1923, 17900) and (485, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (1923, 17900) and (485, 17900) (target, bg)
2020-10-01 18:54:45,762 SpectraRegion INFO: DE data for case: [15]
INFO:SpectraRegion:DE data for case: [15]
2020-10-01 18:54:45,764 SpectraRegion INFO: DE data for control: [0, 2, 6, 3, 1, 7, 5, 4]
INFO:SpectraRegion:DE data for control: [0, 2, 6, 3, 1, 7, 5, 4]
2020-10-01 18:54:45,764 SpectraRegion INFO: Running [15] against [0, 2, 6, 3, 1, 7, 5, 4]
INFO:SpectraRegion:Running [15] against [0, 2, 6, 3, 1, 7, 5, 4]
2020-10-01 18:54:45,765 SpectraRegion INFO: DE result key: ((15,), (0, 1, 2, 3, 4, 5, 6, 7))
INFO:SpectraRegion:DE result key: ((15,), (0, 1, 2, 3, 4, 5, 6, 7))
2020-10-01 18:54:45,766 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:54:45,770 SpectraRegion INFO: DE result for case ((15,), (0, 1, 2, 3, 4, 5, 6, 7)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((15,), (0, 1, 2, 3, 4, 5, 6, 7)) with (17900, 7) results
2020-10-01 18:54:45,771 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:54:45,772 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:54:45,776 SpectraRegion INFO: DE result for case ((15,), (0, 1, 2, 3, 4, 5, 6, 7)) with (12, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((15,), (0, 1, 2, 3, 4, 5, 6, 7)) with (12, 7) results (filtered)
2020-10-01 18:54:45,836 SpectraRegion INFO: Created matrices with shape (91, 17900) and (2317, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (91, 17900) and (2317, 17900) (target, bg)
2020-10-01 18:54:45,860 SpectraRegion INFO: DE result for case ((15,), (0, 1, 2, 3, 4, 5, 6, 7)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((15,), (0, 1, 2, 3, 4, 5, 6, 7)) with (17900, 7) results
2020-10-01 18:54:45,861 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:54:45,863 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:54:45,870 SpectraRegion INFO: DE result for case ((15,), (0, 1, 2, 3, 4, 5, 6, 7)) with (12, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((15,), (0, 1, 2, 3, 4, 5, 6, 7)) with (12, 7) results (filtered)
2020-10-01 18:54:46,024 SpectraRegion INFO: Created matrices with shape (91, 17900) and (2317, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (91, 17900) and (2317, 17900) (target, bg)
2020-10-01 18:54:46,049 SpectraRegion INFO: DE data for case: [2]
INFO:SpectraRegion:DE data for case: [2]
2020-10-01 18:54:46,051 SpectraRegion INFO: DE data for control: [0, 15, 6, 3, 1, 7, 5, 4]
INFO:SpectraRegion:DE data for control: [0, 15, 6, 3, 1, 7, 5, 4]
2020-10-01 18:54:46,052 SpectraRegion INFO: Running [2] against [0, 15, 6, 3, 1, 7, 5, 4]
INFO:SpectraRegion:Running [2] against [0, 15, 6, 3, 1, 7, 5, 4]
2020-10-01 18:54:46,053 SpectraRegion INFO: DE result key: ((2,), (0, 1, 3, 4, 5, 6, 7, 15))
INFO:SpectraRegion:DE result key: ((2,), (0, 1, 3, 4, 5, 6, 7, 15))
2020-10-01 18:54:46,054 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:54:46,057 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 6, 7, 15)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((2,), (0, 1, 3, 4, 5, 6, 7, 15)) with (17900, 7) results
2020-10-01 18:54:46,058 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:54:46,059 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:54:46,064 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 6, 7, 15)) with (970, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((2,), (0, 1, 3, 4, 5, 6, 7, 15)) with (970, 7) results (filtered)
2020-10-01 18:54:46,126 SpectraRegion INFO: Created matrices with shape (79, 17900) and (2329, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (79, 17900) and (2329, 17900) (target, bg)
2020-10-01 18:54:49,555 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 6, 7, 15)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((2,), (0, 1, 3, 4, 5, 6, 7, 15)) with (17900, 7) results
2020-10-01 18:54:49,556 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:54:49,557 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:54:49,561 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 6, 7, 15)) with (970, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((2,), (0, 1, 3, 4, 5, 6, 7, 15)) with (970, 7) results (filtered)
2020-10-01 18:54:49,619 SpectraRegion INFO: Created matrices with shape (79, 17900) and (2329, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (79, 17900) and (2329, 17900) (target, bg)
2020-10-01 18:54:52,974 SpectraRegion INFO: DE data for case: [6]
INFO:SpectraRegion:DE data for case: [6]
2020-10-01 18:54:52,975 SpectraRegion INFO: DE data for control: [0, 15, 2, 3, 1, 7, 5, 4]
INFO:SpectraRegion:DE data for control: [0, 15, 2, 3, 1, 7, 5, 4]
2020-10-01 18:54:52,976 SpectraRegion INFO: Running [6] against [0, 15, 2, 3, 1, 7, 5, 4]
INFO:SpectraRegion:Running [6] against [0, 15, 2, 3, 1, 7, 5, 4]
2020-10-01 18:54:52,977 SpectraRegion INFO: DE result key: ((6,), (0, 1, 2, 3, 4, 5, 7, 15))
INFO:SpectraRegion:DE result key: ((6,), (0, 1, 2, 3, 4, 5, 7, 15))
2020-10-01 18:54:52,978 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:54:52,982 SpectraRegion INFO: DE result for case ((6,), (0, 1, 2, 3, 4, 5, 7, 15)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((6,), (0, 1, 2, 3, 4, 5, 7, 15)) with (17900, 7) results
2020-10-01 18:54:52,983 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:54:52,984 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:54:52,987 SpectraRegion INFO: DE result for case ((6,), (0, 1, 2, 3, 4, 5, 7, 15)) with (1032, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((6,), (0, 1, 2, 3, 4, 5, 7, 15)) with (1032, 7) results (filtered)
2020-10-01 18:54:53,047 SpectraRegion INFO: Created matrices with shape (86, 17900) and (2322, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (86, 17900) and (2322, 17900) (target, bg)
2020-10-01 18:54:56,182 SpectraRegion INFO: DE result for case ((6,), (0, 1, 2, 3, 4, 5, 7, 15)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((6,), (0, 1, 2, 3, 4, 5, 7, 15)) with (17900, 7) results
2020-10-01 18:54:56,183 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:54:56,184 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:54:56,188 SpectraRegion INFO: DE result for case ((6,), (0, 1, 2, 3, 4, 5, 7, 15)) with (1032, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((6,), (0, 1, 2, 3, 4, 5, 7, 15)) with (1032, 7) results (filtered)
2020-10-01 18:54:56,247 SpectraRegion INFO: Created matrices with shape (86, 17900) and (2322, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (86, 17900) and (2322, 17900) (target, bg)
2020-10-01 18:54:59,341 SpectraRegion INFO: DE data for case: [3]
INFO:SpectraRegion:DE data for case: [3]
2020-10-01 18:54:59,342 SpectraRegion INFO: DE data for control: [0, 15, 2, 6, 1, 7, 5, 4]
INFO:SpectraRegion:DE data for control: [0, 15, 2, 6, 1, 7, 5, 4]
2020-10-01 18:54:59,343 SpectraRegion INFO: Running [3] against [0, 15, 2, 6, 1, 7, 5, 4]
INFO:SpectraRegion:Running [3] against [0, 15, 2, 6, 1, 7, 5, 4]
2020-10-01 18:54:59,344 SpectraRegion INFO: DE result key: ((3,), (0, 1, 2, 4, 5, 6, 7, 15))
INFO:SpectraRegion:DE result key: ((3,), (0, 1, 2, 4, 5, 6, 7, 15))
2020-10-01 18:54:59,345 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:54:59,348 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 6, 7, 15)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((3,), (0, 1, 2, 4, 5, 6, 7, 15)) with (17900, 7) results
2020-10-01 18:54:59,349 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:54:59,350 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:54:59,354 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 6, 7, 15)) with (977, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((3,), (0, 1, 2, 4, 5, 6, 7, 15)) with (977, 7) results (filtered)
2020-10-01 18:54:59,410 SpectraRegion INFO: Created matrices with shape (99, 17900) and (2309, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (99, 17900) and (2309, 17900) (target, bg)
2020-10-01 18:55:02,789 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 6, 7, 15)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((3,), (0, 1, 2, 4, 5, 6, 7, 15)) with (17900, 7) results
2020-10-01 18:55:02,791 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:55:02,791 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:55:02,795 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 6, 7, 15)) with (977, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((3,), (0, 1, 2, 4, 5, 6, 7, 15)) with (977, 7) results (filtered)
2020-10-01 18:55:02,853 SpectraRegion INFO: Created matrices with shape (99, 17900) and (2309, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (99, 17900) and (2309, 17900) (target, bg)
2020-10-01 18:55:06,220 SpectraRegion INFO: DE data for case: [1]
INFO:SpectraRegion:DE data for case: [1]
2020-10-01 18:55:06,221 SpectraRegion INFO: DE data for control: [0, 15, 2, 6, 3, 7, 5, 4]
INFO:SpectraRegion:DE data for control: [0, 15, 2, 6, 3, 7, 5, 4]
2020-10-01 18:55:06,222 SpectraRegion INFO: Running [1] against [0, 15, 2, 6, 3, 7, 5, 4]
INFO:SpectraRegion:Running [1] against [0, 15, 2, 6, 3, 7, 5, 4]
2020-10-01 18:55:06,222 SpectraRegion INFO: DE result key: ((1,), (0, 2, 3, 4, 5, 6, 7, 15))
INFO:SpectraRegion:DE result key: ((1,), (0, 2, 3, 4, 5, 6, 7, 15))
2020-10-01 18:55:06,224 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:55:06,227 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 6, 7, 15)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((1,), (0, 2, 3, 4, 5, 6, 7, 15)) with (17900, 7) results
2020-10-01 18:55:06,228 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:55:06,229 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:55:06,233 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 6, 7, 15)) with (1944, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((1,), (0, 2, 3, 4, 5, 6, 7, 15)) with (1944, 7) results (filtered)
2020-10-01 18:55:06,291 SpectraRegion INFO: Created matrices with shape (61, 17900) and (2347, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (61, 17900) and (2347, 17900) (target, bg)
2020-10-01 18:55:13,341 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 6, 7, 15)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((1,), (0, 2, 3, 4, 5, 6, 7, 15)) with (17900, 7) results
2020-10-01 18:55:13,342 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:55:13,343 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:55:13,348 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 6, 7, 15)) with (1944, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((1,), (0, 2, 3, 4, 5, 6, 7, 15)) with (1944, 7) results (filtered)
2020-10-01 18:55:13,405 SpectraRegion INFO: Created matrices with shape (61, 17900) and (2347, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (61, 17900) and (2347, 17900) (target, bg)
2020-10-01 18:55:20,437 SpectraRegion INFO: DE data for case: [7]
INFO:SpectraRegion:DE data for case: [7]
2020-10-01 18:55:20,438 SpectraRegion INFO: DE data for control: [0, 15, 2, 6, 3, 1, 5, 4]
INFO:SpectraRegion:DE data for control: [0, 15, 2, 6, 3, 1, 5, 4]
2020-10-01 18:55:20,439 SpectraRegion INFO: Running [7] against [0, 15, 2, 6, 3, 1, 5, 4]
INFO:SpectraRegion:Running [7] against [0, 15, 2, 6, 3, 1, 5, 4]
2020-10-01 18:55:20,440 SpectraRegion INFO: DE result key: ((7,), (0, 1, 2, 3, 4, 5, 6, 15))
INFO:SpectraRegion:DE result key: ((7,), (0, 1, 2, 3, 4, 5, 6, 15))
2020-10-01 18:55:20,441 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:55:20,444 SpectraRegion INFO: DE result for case ((7,), (0, 1, 2, 3, 4, 5, 6, 15)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((7,), (0, 1, 2, 3, 4, 5, 6, 15)) with (17900, 7) results
2020-10-01 18:55:20,445 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:55:20,446 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:55:20,450 SpectraRegion INFO: DE result for case ((7,), (0, 1, 2, 3, 4, 5, 6, 15)) with (1882, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((7,), (0, 1, 2, 3, 4, 5, 6, 15)) with (1882, 7) results (filtered)
2020-10-01 18:55:20,507 SpectraRegion INFO: Created matrices with shape (39, 17900) and (2369, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (39, 17900) and (2369, 17900) (target, bg)
2020-10-01 18:55:26,145 SpectraRegion INFO: DE result for case ((7,), (0, 1, 2, 3, 4, 5, 6, 15)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((7,), (0, 1, 2, 3, 4, 5, 6, 15)) with (17900, 7) results
2020-10-01 18:55:26,146 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:55:26,147 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:55:26,150 SpectraRegion INFO: DE result for case ((7,), (0, 1, 2, 3, 4, 5, 6, 15)) with (1888, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((7,), (0, 1, 2, 3, 4, 5, 6, 15)) with (1888, 7) results (filtered)
2020-10-01 18:55:26,207 SpectraRegion INFO: Created matrices with shape (39, 17900) and (2369, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (39, 17900) and (2369, 17900) (target, bg)
2020-10-01 18:55:31,880 SpectraRegion INFO: DE data for case: [5]
INFO:SpectraRegion:DE data for case: [5]
2020-10-01 18:55:31,881 SpectraRegion INFO: DE data for control: [0, 15, 2, 6, 3, 1, 7, 4]
INFO:SpectraRegion:DE data for control: [0, 15, 2, 6, 3, 1, 7, 4]
2020-10-01 18:55:31,882 SpectraRegion INFO: Running [5] against [0, 15, 2, 6, 3, 1, 7, 4]
INFO:SpectraRegion:Running [5] against [0, 15, 2, 6, 3, 1, 7, 4]
2020-10-01 18:55:31,883 SpectraRegion INFO: DE result key: ((5,), (0, 1, 2, 3, 4, 6, 7, 15))
INFO:SpectraRegion:DE result key: ((5,), (0, 1, 2, 3, 4, 6, 7, 15))
2020-10-01 18:55:31,884 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:55:31,887 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 6, 7, 15)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((5,), (0, 1, 2, 3, 4, 6, 7, 15)) with (17900, 7) results
2020-10-01 18:55:31,888 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:55:31,889 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:55:31,892 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 6, 7, 15)) with (1840, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((5,), (0, 1, 2, 3, 4, 6, 7, 15)) with (1840, 7) results (filtered)
2020-10-01 18:55:31,949 SpectraRegion INFO: Created matrices with shape (19, 17900) and (2389, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (19, 17900) and (2389, 17900) (target, bg)
2020-10-01 18:55:36,968 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 6, 7, 15)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((5,), (0, 1, 2, 3, 4, 6, 7, 15)) with (17900, 7) results
2020-10-01 18:55:36,969 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:55:36,970 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:55:36,973 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 6, 7, 15)) with (1874, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((5,), (0, 1, 2, 3, 4, 6, 7, 15)) with (1874, 7) results (filtered)
2020-10-01 18:55:37,031 SpectraRegion INFO: Created matrices with shape (19, 17900) and (2389, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (19, 17900) and (2389, 17900) (target, bg)
2020-10-01 18:55:41,699 SpectraRegion INFO: DE data for case: [4]
INFO:SpectraRegion:DE data for case: [4]
2020-10-01 18:55:41,700 SpectraRegion INFO: DE data for control: [0, 15, 2, 6, 3, 1, 7, 5]
INFO:SpectraRegion:DE data for control: [0, 15, 2, 6, 3, 1, 7, 5]
2020-10-01 18:55:41,702 SpectraRegion INFO: Running [4] against [0, 15, 2, 6, 3, 1, 7, 5]
INFO:SpectraRegion:Running [4] against [0, 15, 2, 6, 3, 1, 7, 5]
2020-10-01 18:55:41,703 SpectraRegion INFO: DE result key: ((4,), (0, 1, 2, 3, 5, 6, 7, 15))
INFO:SpectraRegion:DE result key: ((4,), (0, 1, 2, 3, 5, 6, 7, 15))
2020-10-01 18:55:41,704 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:55:41,707 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 6, 7, 15)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((4,), (0, 1, 2, 3, 5, 6, 7, 15)) with (17900, 7) results
2020-10-01 18:55:41,708 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:55:41,709 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:55:41,713 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 6, 7, 15)) with (946, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((4,), (0, 1, 2, 3, 5, 6, 7, 15)) with (946, 7) results (filtered)
2020-10-01 18:55:41,770 SpectraRegion INFO: Created matrices with shape (11, 17900) and (2397, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (11, 17900) and (2397, 17900) (target, bg)
2020-10-01 18:55:44,710 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 6, 7, 15)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((4,), (0, 1, 2, 3, 5, 6, 7, 15)) with (17900, 7) results
2020-10-01 18:55:44,711 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:55:44,712 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:55:44,716 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 6, 7, 15)) with (1065, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((4,), (0, 1, 2, 3, 5, 6, 7, 15)) with (1065, 7) results (filtered)
2020-10-01 18:55:44,774 SpectraRegion INFO: Created matrices with shape (11, 17900) and (2397, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (11, 17900) and (2397, 17900) (target, bg)
2020-10-01 18:55:47,823 SpectraRegion INFO: No cell type info for cluster: '0'
INFO:SpectraRegion:No cell type info for cluster: '0'
2020-10-01 18:55:47,824 SpectraRegion INFO: No cell type info for cluster: '2'
INFO:SpectraRegion:No cell type info for cluster: '2'
2020-10-01 18:55:47,825 SpectraRegion INFO: No cell type info for cluster: '6'
INFO:SpectraRegion:No cell type info for cluster: '6'
2020-10-01 18:55:47,826 SpectraRegion INFO: No cell type info for cluster: '3'
INFO:SpectraRegion:No cell type info for cluster: '3'
2020-10-01 18:55:47,857 SpectraRegion INFO: Cell-type assigned: 1 -> Cardiomyocytes;Heart
INFO:SpectraRegion:Cell-type assigned: 1 -> Cardiomyocytes;Heart
2020-10-01 18:55:47,858 SpectraRegion INFO: Cell-type assigned: 2 -> Adipocytes;Connective tissue
INFO:SpectraRegion:Cell-type assigned: 2 -> Adipocytes;Connective tissue
2020-10-01 18:55:47,862 SpectraRegion INFO: Cell-type assigned: 4 -> Platelets;Blood
INFO:SpectraRegion:Cell-type assigned: 4 -> Platelets;Blood
2020-10-01 18:55:47,864 SpectraRegion INFO: Cell-type assigned: 5 -> Monocytes;Immune system
INFO:SpectraRegion:Cell-type assigned: 5 -> Monocytes;Immune system
2020-10-01 18:55:47,865 SpectraRegion INFO: Cell-type assigned: 10 -> Basophils;Immune system
INFO:SpectraRegion:Cell-type assigned: 10 -> Basophils;Immune system
2020-10-01 18:55:47,867 SpectraRegion INFO: Cell-type assigned: 11 -> NK cells;Immune system
INFO:SpectraRegion:Cell-type assigned: 11 -> NK cells;Immune system
2020-10-01 18:55:47,868 SpectraRegion INFO: Cell-type assigned: 12 -> Cardiomyocytes;Heart
INFO:SpectraRegion:Cell-type assigned: 12 -> Cardiomyocytes;Heart
2020-10-01 18:55:47,870 SpectraRegion INFO: Cell-type assigned: 13 -> Cardiomyocytes;Heart
INFO:SpectraRegion:Cell-type assigned: 13 -> Cardiomyocytes;Heart
2020-10-01 18:55:47,878 SpectraRegion INFO: Segment Image: ./aorta3d/slided/slided.4.clustering.png
INFO:SpectraRegion:Segment Image: ./aorta3d/slided/slided.4.clustering.png
2020-10-01 18:55:47,884 SpectraRegion INFO: Segment Matrix: /mnt/f/dev/git/pyIMS/examples/aorta3d/slided/slided.4.matrix.npy
INFO:SpectraRegion:Segment Matrix: /mnt/f/dev/git/pyIMS/examples/aorta3d/slided/slided.4.matrix.npy
2020-10-01 18:55:47,890 SpectraRegion INFO: Starting Marker Proteins Analysis
INFO:SpectraRegion:Starting Marker Proteins Analysis
2020-10-01 18:55:47,894 SpectraRegion INFO: DE data for case: [0]
INFO:SpectraRegion:DE data for case: [0]
2020-10-01 18:55:47,895 SpectraRegion INFO: DE data for control: [13, 3, 10, 12, 2, 11, 1, 5, 4]
INFO:SpectraRegion:DE data for control: [13, 3, 10, 12, 2, 11, 1, 5, 4]
2020-10-01 18:55:47,896 SpectraRegion INFO: Running [0] against [13, 3, 10, 12, 2, 11, 1, 5, 4]
INFO:SpectraRegion:Running [0] against [13, 3, 10, 12, 2, 11, 1, 5, 4]
2020-10-01 18:55:47,897 SpectraRegion INFO: DE result key: ((0,), (1, 2, 3, 4, 5, 10, 11, 12, 13))
INFO:SpectraRegion:DE result key: ((0,), (1, 2, 3, 4, 5, 10, 11, 12, 13))
2020-10-01 18:55:47,900 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:55:47,902 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((0,), (1, 2, 3, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-10-01 18:55:47,903 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:55:47,904 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:55:47,908 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 10, 11, 12, 13)) with (1491, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((0,), (1, 2, 3, 4, 5, 10, 11, 12, 13)) with (1491, 7) results (filtered)
2020-10-01 18:55:48,033 SpectraRegion INFO: Created matrices with shape (2218, 17900) and (526, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (2218, 17900) and (526, 17900) (target, bg)
2020-10-01 18:55:53,007 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((0,), (1, 2, 3, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-10-01 18:55:53,008 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:55:53,009 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:55:53,013 SpectraRegion INFO: DE result for case ((0,), (1, 2, 3, 4, 5, 10, 11, 12, 13)) with (1491, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((0,), (1, 2, 3, 4, 5, 10, 11, 12, 13)) with (1491, 7) results (filtered)
2020-10-01 18:55:53,079 SpectraRegion INFO: Created matrices with shape (2218, 17900) and (526, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (2218, 17900) and (526, 17900) (target, bg)
2020-10-01 18:55:58,096 SpectraRegion INFO: DE data for case: [13]
INFO:SpectraRegion:DE data for case: [13]
2020-10-01 18:55:58,097 SpectraRegion INFO: DE data for control: [0, 3, 10, 12, 2, 11, 1, 5, 4]
INFO:SpectraRegion:DE data for control: [0, 3, 10, 12, 2, 11, 1, 5, 4]
2020-10-01 18:55:58,098 SpectraRegion INFO: Running [13] against [0, 3, 10, 12, 2, 11, 1, 5, 4]
INFO:SpectraRegion:Running [13] against [0, 3, 10, 12, 2, 11, 1, 5, 4]
2020-10-01 18:55:58,099 SpectraRegion INFO: DE result key: ((13,), (0, 1, 2, 3, 4, 5, 10, 11, 12))
INFO:SpectraRegion:DE result key: ((13,), (0, 1, 2, 3, 4, 5, 10, 11, 12))
2020-10-01 18:55:58,099 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:55:58,102 SpectraRegion INFO: DE result for case ((13,), (0, 1, 2, 3, 4, 5, 10, 11, 12)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((13,), (0, 1, 2, 3, 4, 5, 10, 11, 12)) with (17900, 7) results
2020-10-01 18:55:58,103 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:55:58,105 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:55:58,108 SpectraRegion INFO: DE result for case ((13,), (0, 1, 2, 3, 4, 5, 10, 11, 12)) with (89, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((13,), (0, 1, 2, 3, 4, 5, 10, 11, 12)) with (89, 7) results (filtered)
2020-10-01 18:55:58,173 SpectraRegion INFO: Created matrices with shape (101, 17900) and (2643, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (101, 17900) and (2643, 17900) (target, bg)
2020-10-01 18:55:58,478 SpectraRegion INFO: DE result for case ((13,), (0, 1, 2, 3, 4, 5, 10, 11, 12)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((13,), (0, 1, 2, 3, 4, 5, 10, 11, 12)) with (17900, 7) results
2020-10-01 18:55:58,479 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:55:58,480 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:55:58,484 SpectraRegion INFO: DE result for case ((13,), (0, 1, 2, 3, 4, 5, 10, 11, 12)) with (89, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((13,), (0, 1, 2, 3, 4, 5, 10, 11, 12)) with (89, 7) results (filtered)
2020-10-01 18:55:58,550 SpectraRegion INFO: Created matrices with shape (101, 17900) and (2643, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (101, 17900) and (2643, 17900) (target, bg)
2020-10-01 18:55:58,853 SpectraRegion INFO: DE data for case: [3]
INFO:SpectraRegion:DE data for case: [3]
2020-10-01 18:55:58,854 SpectraRegion INFO: DE data for control: [0, 13, 10, 12, 2, 11, 1, 5, 4]
INFO:SpectraRegion:DE data for control: [0, 13, 10, 12, 2, 11, 1, 5, 4]
2020-10-01 18:55:58,855 SpectraRegion INFO: Running [3] against [0, 13, 10, 12, 2, 11, 1, 5, 4]
INFO:SpectraRegion:Running [3] against [0, 13, 10, 12, 2, 11, 1, 5, 4]
2020-10-01 18:55:58,856 SpectraRegion INFO: DE result key: ((3,), (0, 1, 2, 4, 5, 10, 11, 12, 13))
INFO:SpectraRegion:DE result key: ((3,), (0, 1, 2, 4, 5, 10, 11, 12, 13))
2020-10-01 18:55:58,857 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:55:58,860 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((3,), (0, 1, 2, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-10-01 18:55:58,861 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:55:58,863 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:55:58,866 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 10, 11, 12, 13)) with (1138, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((3,), (0, 1, 2, 4, 5, 10, 11, 12, 13)) with (1138, 7) results (filtered)
2020-10-01 18:55:58,932 SpectraRegion INFO: Created matrices with shape (102, 17900) and (2642, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (102, 17900) and (2642, 17900) (target, bg)
2020-10-01 18:56:02,728 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((3,), (0, 1, 2, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-10-01 18:56:02,729 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:56:02,730 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:56:02,734 SpectraRegion INFO: DE result for case ((3,), (0, 1, 2, 4, 5, 10, 11, 12, 13)) with (1138, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((3,), (0, 1, 2, 4, 5, 10, 11, 12, 13)) with (1138, 7) results (filtered)
2020-10-01 18:56:02,798 SpectraRegion INFO: Created matrices with shape (102, 17900) and (2642, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (102, 17900) and (2642, 17900) (target, bg)
2020-10-01 18:56:06,585 SpectraRegion INFO: DE data for case: [10]
INFO:SpectraRegion:DE data for case: [10]
2020-10-01 18:56:06,586 SpectraRegion INFO: DE data for control: [0, 13, 3, 12, 2, 11, 1, 5, 4]
INFO:SpectraRegion:DE data for control: [0, 13, 3, 12, 2, 11, 1, 5, 4]
2020-10-01 18:56:06,587 SpectraRegion INFO: Running [10] against [0, 13, 3, 12, 2, 11, 1, 5, 4]
INFO:SpectraRegion:Running [10] against [0, 13, 3, 12, 2, 11, 1, 5, 4]
2020-10-01 18:56:06,588 SpectraRegion INFO: DE result key: ((10,), (0, 1, 2, 3, 4, 5, 11, 12, 13))
INFO:SpectraRegion:DE result key: ((10,), (0, 1, 2, 3, 4, 5, 11, 12, 13))
2020-10-01 18:56:06,589 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:56:06,593 SpectraRegion INFO: DE result for case ((10,), (0, 1, 2, 3, 4, 5, 11, 12, 13)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((10,), (0, 1, 2, 3, 4, 5, 11, 12, 13)) with (17900, 7) results
2020-10-01 18:56:06,594 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:56:06,595 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:56:06,599 SpectraRegion INFO: DE result for case ((10,), (0, 1, 2, 3, 4, 5, 11, 12, 13)) with (868, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((10,), (0, 1, 2, 3, 4, 5, 11, 12, 13)) with (868, 7) results (filtered)
2020-10-01 18:56:06,665 SpectraRegion INFO: Created matrices with shape (49, 17900) and (2695, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (49, 17900) and (2695, 17900) (target, bg)
2020-10-01 18:56:09,314 SpectraRegion INFO: DE result for case ((10,), (0, 1, 2, 3, 4, 5, 11, 12, 13)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((10,), (0, 1, 2, 3, 4, 5, 11, 12, 13)) with (17900, 7) results
2020-10-01 18:56:09,315 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:56:09,316 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:56:09,320 SpectraRegion INFO: DE result for case ((10,), (0, 1, 2, 3, 4, 5, 11, 12, 13)) with (868, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((10,), (0, 1, 2, 3, 4, 5, 11, 12, 13)) with (868, 7) results (filtered)
2020-10-01 18:56:09,384 SpectraRegion INFO: Created matrices with shape (49, 17900) and (2695, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (49, 17900) and (2695, 17900) (target, bg)
2020-10-01 18:56:12,069 SpectraRegion INFO: DE data for case: [12]
INFO:SpectraRegion:DE data for case: [12]
2020-10-01 18:56:12,070 SpectraRegion INFO: DE data for control: [0, 13, 3, 10, 2, 11, 1, 5, 4]
INFO:SpectraRegion:DE data for control: [0, 13, 3, 10, 2, 11, 1, 5, 4]
2020-10-01 18:56:12,071 SpectraRegion INFO: Running [12] against [0, 13, 3, 10, 2, 11, 1, 5, 4]
INFO:SpectraRegion:Running [12] against [0, 13, 3, 10, 2, 11, 1, 5, 4]
2020-10-01 18:56:12,072 SpectraRegion INFO: DE result key: ((12,), (0, 1, 2, 3, 4, 5, 10, 11, 13))
INFO:SpectraRegion:DE result key: ((12,), (0, 1, 2, 3, 4, 5, 10, 11, 13))
2020-10-01 18:56:12,073 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:56:12,076 SpectraRegion INFO: DE result for case ((12,), (0, 1, 2, 3, 4, 5, 10, 11, 13)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((12,), (0, 1, 2, 3, 4, 5, 10, 11, 13)) with (17900, 7) results
2020-10-01 18:56:12,077 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:56:12,078 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:56:12,083 SpectraRegion INFO: DE result for case ((12,), (0, 1, 2, 3, 4, 5, 10, 11, 13)) with (1575, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((12,), (0, 1, 2, 3, 4, 5, 10, 11, 13)) with (1575, 7) results (filtered)
2020-10-01 18:56:12,149 SpectraRegion INFO: Created matrices with shape (61, 17900) and (2683, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (61, 17900) and (2683, 17900) (target, bg)
2020-10-01 18:56:16,509 SpectraRegion INFO: DE result for case ((12,), (0, 1, 2, 3, 4, 5, 10, 11, 13)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((12,), (0, 1, 2, 3, 4, 5, 10, 11, 13)) with (17900, 7) results
2020-10-01 18:56:16,510 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:56:16,511 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:56:16,515 SpectraRegion INFO: DE result for case ((12,), (0, 1, 2, 3, 4, 5, 10, 11, 13)) with (1574, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((12,), (0, 1, 2, 3, 4, 5, 10, 11, 13)) with (1574, 7) results (filtered)
2020-10-01 18:56:16,581 SpectraRegion INFO: Created matrices with shape (61, 17900) and (2683, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (61, 17900) and (2683, 17900) (target, bg)
2020-10-01 18:56:20,951 SpectraRegion INFO: DE data for case: [2]
INFO:SpectraRegion:DE data for case: [2]
2020-10-01 18:56:20,952 SpectraRegion INFO: DE data for control: [0, 13, 3, 10, 12, 11, 1, 5, 4]
INFO:SpectraRegion:DE data for control: [0, 13, 3, 10, 12, 11, 1, 5, 4]
2020-10-01 18:56:20,953 SpectraRegion INFO: Running [2] against [0, 13, 3, 10, 12, 11, 1, 5, 4]
INFO:SpectraRegion:Running [2] against [0, 13, 3, 10, 12, 11, 1, 5, 4]
2020-10-01 18:56:20,954 SpectraRegion INFO: DE result key: ((2,), (0, 1, 3, 4, 5, 10, 11, 12, 13))
INFO:SpectraRegion:DE result key: ((2,), (0, 1, 3, 4, 5, 10, 11, 12, 13))
2020-10-01 18:56:20,955 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:56:20,958 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((2,), (0, 1, 3, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-10-01 18:56:20,959 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:56:20,961 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:56:20,964 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 10, 11, 12, 13)) with (2184, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((2,), (0, 1, 3, 4, 5, 10, 11, 12, 13)) with (2184, 7) results (filtered)
2020-10-01 18:56:21,029 SpectraRegion INFO: Created matrices with shape (45, 17900) and (2699, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (45, 17900) and (2699, 17900) (target, bg)
2020-10-01 18:56:29,447 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((2,), (0, 1, 3, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-10-01 18:56:29,448 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:56:29,449 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:56:29,453 SpectraRegion INFO: DE result for case ((2,), (0, 1, 3, 4, 5, 10, 11, 12, 13)) with (2184, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((2,), (0, 1, 3, 4, 5, 10, 11, 12, 13)) with (2184, 7) results (filtered)
2020-10-01 18:56:29,519 SpectraRegion INFO: Created matrices with shape (45, 17900) and (2699, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (45, 17900) and (2699, 17900) (target, bg)
2020-10-01 18:56:37,968 SpectraRegion INFO: DE data for case: [11]
INFO:SpectraRegion:DE data for case: [11]
2020-10-01 18:56:37,969 SpectraRegion INFO: DE data for control: [0, 13, 3, 10, 12, 2, 1, 5, 4]
INFO:SpectraRegion:DE data for control: [0, 13, 3, 10, 12, 2, 1, 5, 4]
2020-10-01 18:56:37,971 SpectraRegion INFO: Running [11] against [0, 13, 3, 10, 12, 2, 1, 5, 4]
INFO:SpectraRegion:Running [11] against [0, 13, 3, 10, 12, 2, 1, 5, 4]
2020-10-01 18:56:37,972 SpectraRegion INFO: DE result key: ((11,), (0, 1, 2, 3, 4, 5, 10, 12, 13))
INFO:SpectraRegion:DE result key: ((11,), (0, 1, 2, 3, 4, 5, 10, 12, 13))
2020-10-01 18:56:37,973 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:56:37,976 SpectraRegion INFO: DE result for case ((11,), (0, 1, 2, 3, 4, 5, 10, 12, 13)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((11,), (0, 1, 2, 3, 4, 5, 10, 12, 13)) with (17900, 7) results
2020-10-01 18:56:37,977 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:56:37,978 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:56:37,983 SpectraRegion INFO: DE result for case ((11,), (0, 1, 2, 3, 4, 5, 10, 12, 13)) with (1552, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((11,), (0, 1, 2, 3, 4, 5, 10, 12, 13)) with (1552, 7) results (filtered)
2020-10-01 18:56:38,050 SpectraRegion INFO: Created matrices with shape (45, 17900) and (2699, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (45, 17900) and (2699, 17900) (target, bg)
2020-10-01 18:56:43,198 SpectraRegion INFO: DE result for case ((11,), (0, 1, 2, 3, 4, 5, 10, 12, 13)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((11,), (0, 1, 2, 3, 4, 5, 10, 12, 13)) with (17900, 7) results
2020-10-01 18:56:43,199 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:56:43,201 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:56:43,205 SpectraRegion INFO: DE result for case ((11,), (0, 1, 2, 3, 4, 5, 10, 12, 13)) with (1554, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((11,), (0, 1, 2, 3, 4, 5, 10, 12, 13)) with (1554, 7) results (filtered)
2020-10-01 18:56:43,270 SpectraRegion INFO: Created matrices with shape (45, 17900) and (2699, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (45, 17900) and (2699, 17900) (target, bg)
2020-10-01 18:56:48,420 SpectraRegion INFO: DE data for case: [1]
INFO:SpectraRegion:DE data for case: [1]
2020-10-01 18:56:48,422 SpectraRegion INFO: DE data for control: [0, 13, 3, 10, 12, 2, 11, 5, 4]
INFO:SpectraRegion:DE data for control: [0, 13, 3, 10, 12, 2, 11, 5, 4]
2020-10-01 18:56:48,423 SpectraRegion INFO: Running [1] against [0, 13, 3, 10, 12, 2, 11, 5, 4]
INFO:SpectraRegion:Running [1] against [0, 13, 3, 10, 12, 2, 11, 5, 4]
2020-10-01 18:56:48,424 SpectraRegion INFO: DE result key: ((1,), (0, 2, 3, 4, 5, 10, 11, 12, 13))
INFO:SpectraRegion:DE result key: ((1,), (0, 2, 3, 4, 5, 10, 11, 12, 13))
2020-10-01 18:56:48,425 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:56:48,428 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((1,), (0, 2, 3, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-10-01 18:56:48,429 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:56:48,429 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:56:48,434 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 10, 11, 12, 13)) with (2040, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((1,), (0, 2, 3, 4, 5, 10, 11, 12, 13)) with (2040, 7) results (filtered)
2020-10-01 18:56:48,500 SpectraRegion INFO: Created matrices with shape (57, 17900) and (2687, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (57, 17900) and (2687, 17900) (target, bg)
2020-10-01 18:56:56,073 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((1,), (0, 2, 3, 4, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-10-01 18:56:56,074 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:56:56,075 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:56:56,079 SpectraRegion INFO: DE result for case ((1,), (0, 2, 3, 4, 5, 10, 11, 12, 13)) with (2038, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((1,), (0, 2, 3, 4, 5, 10, 11, 12, 13)) with (2038, 7) results (filtered)
2020-10-01 18:56:56,153 SpectraRegion INFO: Created matrices with shape (57, 17900) and (2687, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (57, 17900) and (2687, 17900) (target, bg)
2020-10-01 18:57:03,798 SpectraRegion INFO: DE data for case: [5]
INFO:SpectraRegion:DE data for case: [5]
2020-10-01 18:57:03,799 SpectraRegion INFO: DE data for control: [0, 13, 3, 10, 12, 2, 11, 1, 4]
INFO:SpectraRegion:DE data for control: [0, 13, 3, 10, 12, 2, 11, 1, 4]
2020-10-01 18:57:03,800 SpectraRegion INFO: Running [5] against [0, 13, 3, 10, 12, 2, 11, 1, 4]
INFO:SpectraRegion:Running [5] against [0, 13, 3, 10, 12, 2, 11, 1, 4]
2020-10-01 18:57:03,802 SpectraRegion INFO: DE result key: ((5,), (0, 1, 2, 3, 4, 10, 11, 12, 13))
INFO:SpectraRegion:DE result key: ((5,), (0, 1, 2, 3, 4, 10, 11, 12, 13))
2020-10-01 18:57:03,803 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:57:03,805 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 10, 11, 12, 13)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((5,), (0, 1, 2, 3, 4, 10, 11, 12, 13)) with (17900, 7) results
2020-10-01 18:57:03,806 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:57:03,807 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:57:03,811 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 10, 11, 12, 13)) with (1661, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((5,), (0, 1, 2, 3, 4, 10, 11, 12, 13)) with (1661, 7) results (filtered)
2020-10-01 18:57:03,877 SpectraRegion INFO: Created matrices with shape (48, 17900) and (2696, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (48, 17900) and (2696, 17900) (target, bg)
2020-10-01 18:57:09,256 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 10, 11, 12, 13)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((5,), (0, 1, 2, 3, 4, 10, 11, 12, 13)) with (17900, 7) results
2020-10-01 18:57:09,257 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:57:09,258 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:57:09,263 SpectraRegion INFO: DE result for case ((5,), (0, 1, 2, 3, 4, 10, 11, 12, 13)) with (1659, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((5,), (0, 1, 2, 3, 4, 10, 11, 12, 13)) with (1659, 7) results (filtered)
2020-10-01 18:57:09,329 SpectraRegion INFO: Created matrices with shape (48, 17900) and (2696, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (48, 17900) and (2696, 17900) (target, bg)
2020-10-01 18:57:14,534 SpectraRegion INFO: DE data for case: [4]
INFO:SpectraRegion:DE data for case: [4]
2020-10-01 18:57:14,535 SpectraRegion INFO: DE data for control: [0, 13, 3, 10, 12, 2, 11, 1, 5]
INFO:SpectraRegion:DE data for control: [0, 13, 3, 10, 12, 2, 11, 1, 5]
2020-10-01 18:57:14,537 SpectraRegion INFO: Running [4] against [0, 13, 3, 10, 12, 2, 11, 1, 5]
INFO:SpectraRegion:Running [4] against [0, 13, 3, 10, 12, 2, 11, 1, 5]
2020-10-01 18:57:14,538 SpectraRegion INFO: DE result key: ((4,), (0, 1, 2, 3, 5, 10, 11, 12, 13))
INFO:SpectraRegion:DE result key: ((4,), (0, 1, 2, 3, 5, 10, 11, 12, 13))
2020-10-01 18:57:14,539 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:57:14,542 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 10, 11, 12, 13)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((4,), (0, 1, 2, 3, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-10-01 18:57:14,543 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:57:14,544 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:57:14,548 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 10, 11, 12, 13)) with (2953, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((4,), (0, 1, 2, 3, 5, 10, 11, 12, 13)) with (2953, 7) results (filtered)
2020-10-01 18:57:14,611 SpectraRegion INFO: Created matrices with shape (18, 17900) and (2726, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (18, 17900) and (2726, 17900) (target, bg)
2020-10-01 18:57:25,081 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 10, 11, 12, 13)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((4,), (0, 1, 2, 3, 5, 10, 11, 12, 13)) with (17900, 7) results
2020-10-01 18:57:25,082 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:57:25,083 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:57:25,087 SpectraRegion INFO: DE result for case ((4,), (0, 1, 2, 3, 5, 10, 11, 12, 13)) with (2920, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((4,), (0, 1, 2, 3, 5, 10, 11, 12, 13)) with (2920, 7) results (filtered)
2020-10-01 18:57:25,150 SpectraRegion INFO: Created matrices with shape (18, 17900) and (2726, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (18, 17900) and (2726, 17900) (target, bg)
2020-10-01 18:57:36,016 SpectraRegion INFO: No cell type info for cluster: '0'
INFO:SpectraRegion:No cell type info for cluster: '0'
2020-10-01 18:57:36,018 SpectraRegion INFO: No cell type info for cluster: '3'
INFO:SpectraRegion:No cell type info for cluster: '3'
2020-10-01 18:57:36,031 SpectraRegion INFO: Cell-type assigned: 9 -> Endothelial cells;Vasculature
INFO:SpectraRegion:Cell-type assigned: 9 -> Endothelial cells;Vasculature
2020-10-01 18:57:36,033 SpectraRegion INFO: Cell-type assigned: 10 -> Gamma delta T cells;Immune system
INFO:SpectraRegion:Cell-type assigned: 10 -> Gamma delta T cells;Immune system
2020-10-01 18:57:36,034 SpectraRegion INFO: Cell-type assigned: 11 -> Platelets;Blood
INFO:SpectraRegion:Cell-type assigned: 11 -> Platelets;Blood
2020-10-01 18:57:36,036 SpectraRegion INFO: Cell-type assigned: 14 -> Adipocytes;Connective tissue
INFO:SpectraRegion:Cell-type assigned: 14 -> Adipocytes;Connective tissue
2020-10-01 18:57:36,037 SpectraRegion INFO: Cell-type assigned: 15 -> Cardiomyocytes;Heart
INFO:SpectraRegion:Cell-type assigned: 15 -> Cardiomyocytes;Heart
2020-10-01 18:57:36,044 SpectraRegion INFO: Segment Image: ./aorta3d/slided/slided.5.clustering.png
INFO:SpectraRegion:Segment Image: ./aorta3d/slided/slided.5.clustering.png
2020-10-01 18:57:36,051 SpectraRegion INFO: Segment Matrix: /mnt/f/dev/git/pyIMS/examples/aorta3d/slided/slided.5.matrix.npy
INFO:SpectraRegion:Segment Matrix: /mnt/f/dev/git/pyIMS/examples/aorta3d/slided/slided.5.matrix.npy
2020-10-01 18:57:36,056 SpectraRegion INFO: Starting Marker Proteins Analysis
INFO:SpectraRegion:Starting Marker Proteins Analysis
2020-10-01 18:57:36,060 SpectraRegion INFO: DE data for case: [0]
INFO:SpectraRegion:DE data for case: [0]
2020-10-01 18:57:36,061 SpectraRegion INFO: DE data for control: [15, 12, 11, 14, 10, 9]
INFO:SpectraRegion:DE data for control: [15, 12, 11, 14, 10, 9]
2020-10-01 18:57:36,063 SpectraRegion INFO: Running [0] against [15, 12, 11, 14, 10, 9]
INFO:SpectraRegion:Running [0] against [15, 12, 11, 14, 10, 9]
2020-10-01 18:57:36,065 SpectraRegion INFO: DE result key: ((0,), (9, 10, 11, 12, 14, 15))
INFO:SpectraRegion:DE result key: ((0,), (9, 10, 11, 12, 14, 15))
2020-10-01 18:57:36,066 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:57:36,068 SpectraRegion INFO: DE result for case ((0,), (9, 10, 11, 12, 14, 15)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((0,), (9, 10, 11, 12, 14, 15)) with (17900, 7) results
2020-10-01 18:57:36,070 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:57:36,071 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:57:36,075 SpectraRegion INFO: DE result for case ((0,), (9, 10, 11, 12, 14, 15)) with (1125, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((0,), (9, 10, 11, 12, 14, 15)) with (1125, 7) results (filtered)
2020-10-01 18:57:36,211 SpectraRegion INFO: Created matrices with shape (2815, 17900) and (253, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (2815, 17900) and (253, 17900) (target, bg)
2020-10-01 18:57:39,206 SpectraRegion INFO: DE result for case ((0,), (9, 10, 11, 12, 14, 15)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((0,), (9, 10, 11, 12, 14, 15)) with (17900, 7) results
2020-10-01 18:57:39,207 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:57:39,208 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:57:39,212 SpectraRegion INFO: DE result for case ((0,), (9, 10, 11, 12, 14, 15)) with (1125, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((0,), (9, 10, 11, 12, 14, 15)) with (1125, 7) results (filtered)
2020-10-01 18:57:39,283 SpectraRegion INFO: Created matrices with shape (2815, 17900) and (253, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (2815, 17900) and (253, 17900) (target, bg)
2020-10-01 18:57:42,231 SpectraRegion INFO: DE data for case: [15]
INFO:SpectraRegion:DE data for case: [15]
2020-10-01 18:57:42,233 SpectraRegion INFO: DE data for control: [0, 12, 11, 14, 10, 9]
INFO:SpectraRegion:DE data for control: [0, 12, 11, 14, 10, 9]
2020-10-01 18:57:42,234 SpectraRegion INFO: Running [15] against [0, 12, 11, 14, 10, 9]
INFO:SpectraRegion:Running [15] against [0, 12, 11, 14, 10, 9]
2020-10-01 18:57:42,235 SpectraRegion INFO: DE result key: ((15,), (0, 9, 10, 11, 12, 14))
INFO:SpectraRegion:DE result key: ((15,), (0, 9, 10, 11, 12, 14))
2020-10-01 18:57:42,235 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:57:42,238 SpectraRegion INFO: DE result for case ((15,), (0, 9, 10, 11, 12, 14)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((15,), (0, 9, 10, 11, 12, 14)) with (17900, 7) results
2020-10-01 18:57:42,239 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:57:42,240 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:57:42,245 SpectraRegion INFO: DE result for case ((15,), (0, 9, 10, 11, 12, 14)) with (863, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((15,), (0, 9, 10, 11, 12, 14)) with (863, 7) results (filtered)
2020-10-01 18:57:42,315 SpectraRegion INFO: Created matrices with shape (98, 17900) and (2970, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (98, 17900) and (2970, 17900) (target, bg)
2020-10-01 18:57:44,680 SpectraRegion INFO: DE result for case ((15,), (0, 9, 10, 11, 12, 14)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((15,), (0, 9, 10, 11, 12, 14)) with (17900, 7) results
2020-10-01 18:57:44,681 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:57:44,682 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:57:44,686 SpectraRegion INFO: DE result for case ((15,), (0, 9, 10, 11, 12, 14)) with (863, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((15,), (0, 9, 10, 11, 12, 14)) with (863, 7) results (filtered)
2020-10-01 18:57:44,757 SpectraRegion INFO: Created matrices with shape (98, 17900) and (2970, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (98, 17900) and (2970, 17900) (target, bg)
2020-10-01 18:57:47,103 SpectraRegion INFO: DE data for case: [12]
INFO:SpectraRegion:DE data for case: [12]
2020-10-01 18:57:47,104 SpectraRegion INFO: DE data for control: [0, 15, 11, 14, 10, 9]
INFO:SpectraRegion:DE data for control: [0, 15, 11, 14, 10, 9]
2020-10-01 18:57:47,105 SpectraRegion INFO: Running [12] against [0, 15, 11, 14, 10, 9]
INFO:SpectraRegion:Running [12] against [0, 15, 11, 14, 10, 9]
2020-10-01 18:57:47,106 SpectraRegion INFO: DE result key: ((12,), (0, 9, 10, 11, 14, 15))
INFO:SpectraRegion:DE result key: ((12,), (0, 9, 10, 11, 14, 15))
2020-10-01 18:57:47,107 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:57:47,110 SpectraRegion INFO: DE result for case ((12,), (0, 9, 10, 11, 14, 15)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((12,), (0, 9, 10, 11, 14, 15)) with (17900, 7) results
2020-10-01 18:57:47,111 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:57:47,112 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:57:47,116 SpectraRegion INFO: DE result for case ((12,), (0, 9, 10, 11, 14, 15)) with (856, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((12,), (0, 9, 10, 11, 14, 15)) with (856, 7) results (filtered)
2020-10-01 18:57:47,190 SpectraRegion INFO: Created matrices with shape (33, 17900) and (3035, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (33, 17900) and (3035, 17900) (target, bg)
2020-10-01 18:57:49,633 SpectraRegion INFO: DE result for case ((12,), (0, 9, 10, 11, 14, 15)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((12,), (0, 9, 10, 11, 14, 15)) with (17900, 7) results
2020-10-01 18:57:49,634 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:57:49,635 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:57:49,639 SpectraRegion INFO: DE result for case ((12,), (0, 9, 10, 11, 14, 15)) with (855, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((12,), (0, 9, 10, 11, 14, 15)) with (855, 7) results (filtered)
2020-10-01 18:57:49,712 SpectraRegion INFO: Created matrices with shape (33, 17900) and (3035, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (33, 17900) and (3035, 17900) (target, bg)
2020-10-01 18:57:52,110 SpectraRegion INFO: DE data for case: [11]
INFO:SpectraRegion:DE data for case: [11]
2020-10-01 18:57:52,111 SpectraRegion INFO: DE data for control: [0, 15, 12, 14, 10, 9]
INFO:SpectraRegion:DE data for control: [0, 15, 12, 14, 10, 9]
2020-10-01 18:57:52,112 SpectraRegion INFO: Running [11] against [0, 15, 12, 14, 10, 9]
INFO:SpectraRegion:Running [11] against [0, 15, 12, 14, 10, 9]
2020-10-01 18:57:52,113 SpectraRegion INFO: DE result key: ((11,), (0, 9, 10, 12, 14, 15))
INFO:SpectraRegion:DE result key: ((11,), (0, 9, 10, 12, 14, 15))
2020-10-01 18:57:52,114 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:57:52,117 SpectraRegion INFO: DE result for case ((11,), (0, 9, 10, 12, 14, 15)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((11,), (0, 9, 10, 12, 14, 15)) with (17900, 7) results
2020-10-01 18:57:52,118 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:57:52,119 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:57:52,125 SpectraRegion INFO: DE result for case ((11,), (0, 9, 10, 12, 14, 15)) with (2583, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((11,), (0, 9, 10, 12, 14, 15)) with (2583, 7) results (filtered)
2020-10-01 18:57:52,197 SpectraRegion INFO: Created matrices with shape (24, 17900) and (3044, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (24, 17900) and (3044, 17900) (target, bg)
2020-10-01 18:58:01,721 SpectraRegion INFO: DE result for case ((11,), (0, 9, 10, 12, 14, 15)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((11,), (0, 9, 10, 12, 14, 15)) with (17900, 7) results
2020-10-01 18:58:01,722 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:58:01,723 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:58:01,727 SpectraRegion INFO: DE result for case ((11,), (0, 9, 10, 12, 14, 15)) with (2542, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((11,), (0, 9, 10, 12, 14, 15)) with (2542, 7) results (filtered)
2020-10-01 18:58:01,798 SpectraRegion INFO: Created matrices with shape (24, 17900) and (3044, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (24, 17900) and (3044, 17900) (target, bg)
2020-10-01 18:58:11,142 SpectraRegion INFO: DE data for case: [14]
INFO:SpectraRegion:DE data for case: [14]
2020-10-01 18:58:11,144 SpectraRegion INFO: DE data for control: [0, 15, 12, 11, 10, 9]
INFO:SpectraRegion:DE data for control: [0, 15, 12, 11, 10, 9]
2020-10-01 18:58:11,145 SpectraRegion INFO: Running [14] against [0, 15, 12, 11, 10, 9]
INFO:SpectraRegion:Running [14] against [0, 15, 12, 11, 10, 9]
2020-10-01 18:58:11,146 SpectraRegion INFO: DE result key: ((14,), (0, 9, 10, 11, 12, 15))
INFO:SpectraRegion:DE result key: ((14,), (0, 9, 10, 11, 12, 15))
2020-10-01 18:58:11,147 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:58:11,150 SpectraRegion INFO: DE result for case ((14,), (0, 9, 10, 11, 12, 15)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((14,), (0, 9, 10, 11, 12, 15)) with (17900, 7) results
2020-10-01 18:58:11,150 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:58:11,151 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:58:11,155 SpectraRegion INFO: DE result for case ((14,), (0, 9, 10, 11, 12, 15)) with (2209, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((14,), (0, 9, 10, 11, 12, 15)) with (2209, 7) results (filtered)
2020-10-01 18:58:11,226 SpectraRegion INFO: Created matrices with shape (28, 17900) and (3040, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (28, 17900) and (3040, 17900) (target, bg)
2020-10-01 18:58:18,056 SpectraRegion INFO: DE result for case ((14,), (0, 9, 10, 11, 12, 15)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((14,), (0, 9, 10, 11, 12, 15)) with (17900, 7) results
2020-10-01 18:58:18,057 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:58:18,058 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:58:18,062 SpectraRegion INFO: DE result for case ((14,), (0, 9, 10, 11, 12, 15)) with (2219, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((14,), (0, 9, 10, 11, 12, 15)) with (2219, 7) results (filtered)
2020-10-01 18:58:18,132 SpectraRegion INFO: Created matrices with shape (28, 17900) and (3040, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (28, 17900) and (3040, 17900) (target, bg)
2020-10-01 18:58:24,941 SpectraRegion INFO: DE data for case: [10]
INFO:SpectraRegion:DE data for case: [10]
2020-10-01 18:58:24,942 SpectraRegion INFO: DE data for control: [0, 15, 12, 11, 14, 9]
INFO:SpectraRegion:DE data for control: [0, 15, 12, 11, 14, 9]
2020-10-01 18:58:24,943 SpectraRegion INFO: Running [10] against [0, 15, 12, 11, 14, 9]
INFO:SpectraRegion:Running [10] against [0, 15, 12, 11, 14, 9]
2020-10-01 18:58:24,944 SpectraRegion INFO: DE result key: ((10,), (0, 9, 11, 12, 14, 15))
INFO:SpectraRegion:DE result key: ((10,), (0, 9, 11, 12, 14, 15))
2020-10-01 18:58:24,945 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:58:24,948 SpectraRegion INFO: DE result for case ((10,), (0, 9, 11, 12, 14, 15)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((10,), (0, 9, 11, 12, 14, 15)) with (17900, 7) results
2020-10-01 18:58:24,950 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:58:24,951 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:58:24,955 SpectraRegion INFO: DE result for case ((10,), (0, 9, 11, 12, 14, 15)) with (1799, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((10,), (0, 9, 11, 12, 14, 15)) with (1799, 7) results (filtered)
2020-10-01 18:58:25,027 SpectraRegion INFO: Created matrices with shape (36, 17900) and (3032, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (36, 17900) and (3032, 17900) (target, bg)
2020-10-01 18:58:29,770 SpectraRegion INFO: DE result for case ((10,), (0, 9, 11, 12, 14, 15)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((10,), (0, 9, 11, 12, 14, 15)) with (17900, 7) results
2020-10-01 18:58:29,771 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:58:29,772 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:58:29,776 SpectraRegion INFO: DE result for case ((10,), (0, 9, 11, 12, 14, 15)) with (1807, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((10,), (0, 9, 11, 12, 14, 15)) with (1807, 7) results (filtered)
2020-10-01 18:58:29,847 SpectraRegion INFO: Created matrices with shape (36, 17900) and (3032, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (36, 17900) and (3032, 17900) (target, bg)
2020-10-01 18:58:34,589 SpectraRegion INFO: DE data for case: [9]
INFO:SpectraRegion:DE data for case: [9]
2020-10-01 18:58:34,590 SpectraRegion INFO: DE data for control: [0, 15, 12, 11, 14, 10]
INFO:SpectraRegion:DE data for control: [0, 15, 12, 11, 14, 10]
2020-10-01 18:58:34,591 SpectraRegion INFO: Running [9] against [0, 15, 12, 11, 14, 10]
INFO:SpectraRegion:Running [9] against [0, 15, 12, 11, 14, 10]
2020-10-01 18:58:34,592 SpectraRegion INFO: DE result key: ((9,), (0, 10, 11, 12, 14, 15))
INFO:SpectraRegion:DE result key: ((9,), (0, 10, 11, 12, 14, 15))
2020-10-01 18:58:34,593 SpectraRegion INFO: DE result key already exists
INFO:SpectraRegion:DE result key already exists
2020-10-01 18:58:34,596 SpectraRegion INFO: DE result for case ((9,), (0, 10, 11, 12, 14, 15)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((9,), (0, 10, 11, 12, 14, 15)) with (17900, 7) results
2020-10-01 18:58:34,598 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:58:34,599 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:58:34,603 SpectraRegion INFO: DE result for case ((9,), (0, 10, 11, 12, 14, 15)) with (1932, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((9,), (0, 10, 11, 12, 14, 15)) with (1932, 7) results (filtered)
2020-10-01 18:58:34,674 SpectraRegion INFO: Created matrices with shape (34, 17900) and (3034, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (34, 17900) and (3034, 17900) (target, bg)
2020-10-01 18:58:40,396 SpectraRegion INFO: DE result for case ((9,), (0, 10, 11, 12, 14, 15)) with (17900, 7) results
INFO:SpectraRegion:DE result for case ((9,), (0, 10, 11, 12, 14, 15)) with (17900, 7) results
2020-10-01 18:58:40,397 SpectraRegion INFO: DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
INFO:SpectraRegion:DF column names ['gene', 'pval', 'qval', 'log2fc', 'mean', 'zero_mean', 'zero_variance']
2020-10-01 18:58:40,398 SpectraRegion INFO: DE result logFC inversed
INFO:SpectraRegion:DE result logFC inversed
2020-10-01 18:58:40,403 SpectraRegion INFO: DE result for case ((9,), (0, 10, 11, 12, 14, 15)) with (1937, 7) results (filtered)
INFO:SpectraRegion:DE result for case ((9,), (0, 10, 11, 12, 14, 15)) with (1937, 7) results (filtered)
2020-10-01 18:58:40,473 SpectraRegion INFO: Created matrices with shape (34, 17900) and (3034, 17900) (target, bg)
INFO:SpectraRegion:Created matrices with shape (34, 17900) and (3034, 17900) (target, bg)
2020-10-01 18:58:46,290 SpectraRegion INFO: No cell type info for cluster: '0'
INFO:SpectraRegion:No cell type info for cluster: '0'
2020-10-01 18:58:46,292 SpectraRegion INFO: No cell type info for cluster: '12'
INFO:SpectraRegion:No cell type info for cluster: '12'
! python3 /mnt/f/dev/git/Aorta3D/files2model/register/register_pimz.py --id slided \
--files \
/mnt/f/dev/git/pyIMS/examples/aorta3d/slided/slided.1.info \
/mnt/f/dev/git/pyIMS/examples/aorta3d/slided/slided.0.info \
/mnt/f/dev/git/pyIMS/examples/aorta3d/slided/slided.4.info \
/mnt/f/dev/git/pyIMS/examples/aorta3d/slided/slided.5.info \
--output /mnt/f/dev/git/pyIMS/examples/aorta3d/slided/registered/slided_server.conf
2020-10-02 13:02:52.506568: W tensorflow/stream_executor/platform/default/dso_loader.cc:59] Could not load dynamic library 'libcudart.so.10.1'; dlerror: libcudart.so.10.1: cannot open shared object file: No such file or directory
2020-10-02 13:02:52.506609: I tensorflow/stream_executor/cuda/cudart_stub.cc:29] Ignore above cudart dlerror if you do not have a GPU set up on your machine.
<frozen importlib._bootstrap>:219: FutureWarning: Passing (type, 1) or '1type' as a synonym of type is deprecated; in a future version of numpy, it will be understood as (type, (1,)) / '(1,)type'.
/mnt/f/dev/git/pyIMS/examples/aorta3d/slided/slided.1.info
['region', 'path_upgma', 'info', 'segment_file']
/mnt/f/dev/git/pyIMS/examples/aorta3d/slided/slided.0.info
['region', 'path_upgma', 'info', 'segment_file']
/mnt/f/dev/git/pyIMS/examples/aorta3d/slided/slided.4.info
['region', 'path_upgma', 'info', 'segment_file']
/mnt/f/dev/git/pyIMS/examples/aorta3d/slided/slided.5.info
['region', 'path_upgma', 'info', 'segment_file']
Determined most similar image: 3, /mnt/f/dev/git/pyIMS/examples/aorta3d/slided/slided.5.clustering.png
/mnt/f/dev/git/Aorta3D/files2model/register/register_pimz.py:270: FutureWarning: The behavior of rgb2gray will change in scikit-image 0.19. Currently, rgb2gray allows 2D grayscale image to be passed as inputs and leaves them unmodified as outputs. Starting from version 0.19, 2D arrays will be treated as 1D images with 3 channels.
trans = register_pair.start_ransac(img1=rgb2gray(masks[mostSimilar]), img2=rgb2gray(curImgMask), brief=True, common_factor=cf)
(208, 236)
(172, 224)
/mnt/f/dev/git/Aorta3D/files2model/register/register_pair.py:106: FutureWarning: Until version 0.16, threshold_rel was set to 0.1 by default. Starting from version 0.16, the default value is set to None. Until version 0.18, a None value corresponds to a threshold value of 0.1. The default behavior will match skimage.feature.peak_local_max. To avoid this warning, set threshold_rel=0.
keypoints1 = corner_peaks(corner_harris(img1), min_distance=5)
/mnt/f/dev/git/Aorta3D/files2model/register/register_pair.py:107: FutureWarning: Until version 0.16, threshold_rel was set to 0.1 by default. Starting from version 0.16, the default value is set to None. Until version 0.18, a None value corresponds to a threshold value of 0.1. The default behavior will match skimage.feature.peak_local_max. To avoid this warning, set threshold_rel=0.
keypoints2 = corner_peaks(corner_harris(img2), min_distance=5)
/mnt/f/dev/git/pyIMS/examples/aorta3d/slided/slided.1.clustering.png (172, 224) (172, 224) (172, 224) (172, 224)
['/mnt/f/dev/git/pyIMS/examples/aorta3d/slided/slided.1.clustering.png_reg_seg.png']
0 /mnt/f/dev/git/pyIMS/examples/aorta3d/slided/slided.1.clustering.png_reg_seg.png
0 /mnt/f/dev/git/pyIMS/examples/aorta3d/slided/slided.1.clustering.png_reg_seg.png
broke at 0 /mnt/f/dev/git/pyIMS/examples/aorta3d/slided/slided.1.clustering.png_reg_seg.png
/mnt/f/dev/git/Aorta3D/files2model/register/register_pimz.py:82: RuntimeWarning: invalid value encountered in true_divide
V = V / np.max(V)
/mnt/f/dev/git/Aorta3D/files2model/register/register_pimz.py:94: RuntimeWarning: invalid value encountered in greater
Vtaorta[Vtaorta > 10] = 100
(172, 224, 30)
Traceback (most recent call last):
File "/mnt/f/dev/git/Aorta3D/files2model/register/register_pimz.py", line 297, in <module>
createStlModel([outName + '_reg_seg.png'], outName + "_model.stl", None, full=True)
File "/mnt/f/dev/git/Aorta3D/files2model/register/register_pimz.py", line 103, in createStlModel
s_aortaVertices,s_aortaFaces = sn.surface_net(Vtaorta, 75)
File "/mnt/f/dev/git/Aorta3D/files2model/register/stlstuff.py", line 88, in surface_net
vdata[vidx]= data[i,j,k]
ValueError: cannot convert float NaN to integer
Here a CombinedSpectra is creates. This class allows to compare multiple SpectraRegions
combSpec = CombinedSpectra({0: slided_0, 1: slided_1, 4: slided_4, 5: slided_5})
The consensus_smilarity() function calculates the similarity between all the contained spectra’s regions using the average cluster/region spectra.
combSpec.consensus_similarity()
combSpec.consensus_similarity_matrix
Since the inspection of this matrix becomes tidious, particularly if large, it an be plotted :)
combSpec.plot_consensus_similarity()
It can already be seen that some classes are more similar to each other, than others.
Now the single slide/region clusters are reclustered.
combSpec.cluster_concensus_spectra(number_of_clusters=10)
A detailed print out of the newly assigned cluster for each slide, region.
combSpec.region_cluster2cluster
The new assignment can also be plotted:
combSpec.plot_common_segments()
And of course, interpreted.
Slide 1 and Slide 5 appear to be similar, and slide 0 and slide 4.
combSpec.plot_common_segments(highlight=(4))
Similar to a normal SpectraRegion, also specific masses can be plotted. Here we can see that mass 14954 is not present in slides 1 and 5!
_ = combSpec.mass_heatmap(14954)
A first DE Analysis: Tunica Media¶
Slide 0 cluster 2 and slide 4 cluster 1 seem to be very similar. We now want to see whether some differences can be observed.
For this, we suspect that slides 0 and 4 are disease-samples, and slides 1 and 5 are controls.
We now compare the outer regions of both samples:
combSpec.regions["slided_0"].plot_segments(highlight=[8,9])
combSpec.regions["slided_1"].plot_segments(highlight=[12,14])
combSpec.get_internormed_regions()
print(np.median(combSpec.region_array_scaled["slided_1"]), np.median( combSpec.regions["slided_1"].region_array))
combSpec.mass_intensity(14954, regions=["slided_0", "slided_1"], scaled=False)
combSpec.mass_intensity(14954, regions=["slided_0", "slided_1"], scaled=True)
resdf, expr, pdata = combSpec.find_markers("slided_0", [8,9], "slided_1", [12,14], pw, scaled=True)
combSpec.list_de_results()
mydf = resdf["ttest"][('slided_0', (8,9,), 'slided_1', (12,14,))]
In order to plot a volcano plot for the differential masses/proteins, the result DF is subset to only have masses displayed that have a mean intensity of at least 2.
from adjustText import adjust_text
pd.set_option('display.max_rows', None)
mydf
plt.figure(figsize=(16,10))
xydots = [(x,y) for x,y in zip(list(-mydf["avg_logFC"]), list(-np.log10(mydf["qvalue"])))]
dotgene = list(mydf["gene"])
texts = []
seenProts = set()
for i in range(len(xydots)):
x = xydots[i][0]
y = xydots[i][1]
if not dotgene[i] in seenProts and abs(y) >= 10 and abs(x) >= 0.5:
texts.append(plt.text(x * (1 + 0.01), y * (1 + 0.01) , dotgene[i], fontsize=12))
plt.plot(x, y, 'ro')
seenProts.add(dotgene[i])
else:
plt.plot(x, y, 'bo')
adjust_text(texts, force_points=0.2, force_text=0.2, expand_points=(1, 1), expand_text=(1, 1), arrowprops=dict(arrowstyle="-", color='black', lw=0.5))
plt.show()
In this comparison the tunica media of atherosclerotic (slided_0) and control (slided_1) aorta was compared. It must be noted, that the selected regions are considerably thicker in slided_1 compared to slided_0, but it is a known phenomenon that “The media underlying intimal athero- sclerotic plaque is considerably thinner” (Milutinović, A., Šuput, D., & Zorc-Pleskovič, R. (2020). Pathogenesis of atherosclerosis in the tunica intima, media, and adventitia of coronary arteries: An updated review. In Bosnian Journal of Basic Medical Sciences (Vol. 20, Issue 1, pp. 21–30). Association of Basic Medical Sciences of FBIH. https://doi.org/10.17305/bjbms.2019.4320 ).
However, due to the proximity to the background tissue (liver), for each mass it should be checked whether this mass is not also prevalent in the background tissue and therefore is present in the cluster due to diffusion, bad clustering, or other reasons. The mass_heatmap-function give a good and fast overview!
Tmsb4x -¶
First it is very interesting to see a difference in Tmsb4x between the (suspected) control and disease aortas. The diseased-aorta-media has significantly less Tmsb4x (-0.5). This is not surprising, but was already found 5 years ago ( Zaima, N., Sasaki, T., Tanaka, H., Cheng, X. W., Onoue, K., Hayasaka, T., Goto-Inoue, N., Enomoto, H., Unno, N., Kuzuya, M., & Setou, M. (2011). Imaging mass spectrometry-based histopathologic examination of atherosclerotic lesions. Atherosclerosis, 217(2), 427–432. https://doi.org/10.1016/j.atherosclerosis.2011.03.044 ). Indeed, this can be seen as proof-of-concept.
Ptges3 +¶
On the contrary, there are other proteins, which are up-regulated in the atherosclerotic aorta. Among these is Ptges3 (+1.25), which was shown to be involved in the inflammatory response of bovine endometrial epithelial cells. Almughlliq, F. B., Koh, Y. Q., Peiris, H. N., Vaswani, K., Arachchige, B. J., Reed, S., & Mitchell, M. D. (2018). Eicosanoid pathway expression in bovine endometrial epithelial and stromal cells in response to lipopolysaccharide, interleukin 1 beta, and tumor necrosis factor alpha. Reproductive Biology, 18(4), 390–396. https://doi.org/10.1016/j.repbio.2018.10.001
Further proteins of interest include
Chchd4 +¶
Chchd4 was already identified as a biomarker for chronic obstructive pulmonary disease (COPD), a chronic inflammatory disease - in such, maybe? comparable to atherosclerosis. Chchd4 affects the mitochondrial metabolism, being named in the p53-axis controlling tumor proliferation.
Maghsoudloo, M., Azimzadeh Jamalkandi, S., Najafi, A., & Masoudi-Nejad, A. (2020). An efficient hybrid feature selection method to identify potential biomarkers in common chronic lung inflammatory diseases. Genomics, 112(5), 3284–3293. https://doi.org/10.1016/j.ygeno.2020.06.010
Coa6 –¶
The respiratory metabolism appears to play an important role in atherosclerosis. Many regulated proteins fall into this category. A Coa6 deficiency is known to be causing many disease phenotypes, including cardiomyopathy, encephalomyopathy, skeletal muscle myopathy, Leigh syndrome, metabolic acidosis and occasional hepatic failure. In summary: Coa6 deficiency does not contribute to a healthy Aorta.
Ghosh, A., Trivedi, P. P., Timbalia, S. A., Griffin, A. T., Rahn, J. J., Chan, S. S. L., & Gohil, V. M. (2014). Copper supplementation restores cytochrome c oxidase assembly defect in a mitochondrial disease model of COA6 deficiency. Human Molecular Genetics, 23(13), 3596–3606. https://doi.org/10.1093/hmg/ddu069
Cst3 +¶
Serum Cystein3 is associated with subclinical atherosclerosis, which can also be seen in the middle of the IMS aorta. It also does not seem unlikely, that Cst3 is elsewhere upregulated in atherosclerotic aorta.
Chung, Y. K., Lee, Y. J., Kim, K. W., Cho, R. K., Chung, S. M., Moon, J. S., Yoon, J. S., Won, K. C., & Lee, H. W. (2018). Serum cystatin C is associated with subclinical atherosclerosis in patients with type 2 diabetes: A retrospective study. Diabetes and Vascular Disease Research, 15(1), 24–30. https://doi.org/10.1177/1479164117738156
Ndufa11 +, Cox7a2 -¶
This proteins is of interesting, and together with other identified proteins ( like Cox7a2 ), is part of the mitochondria. Atherosclerosis therefore might have an effect on the energy metabolism, similar to an ischemic stroke: James, R., Searcy, J. L., Le Bihan, T., Martin, S. F., Gliddon, C. M., Povey, J., Deighton, R. F., Kerr, L. E., McCulloch, J., & Horsburgh, K. (2012). Proteomic analysis of mitochondria in APOE transgenic mice and in response to an ischemic challenge. Journal of Cerebral Blood Flow and Metabolism, 32(1), 164–176. https://doi.org/10.1038/jcbfm.2011.120
Ndufb6 +¶
Ndufb6 was identified to being critical for the development of PM2.5-induced fibrosis in mouse lungs. Therefore it is likely related to inflammatory processes.
Han, X., Liu, H., Zhang, Z., Yang, W., Wu, C., Liu, X., Zhang, F., Sun, B., Zhao, Y., Jiang, G., Yang, Y. G., & Ding, W. (2020). Epitranscriptomic 5-Methylcytosine Profile in PM2.5-induced Mouse Pulmonary Fibrosis. Genomics, Proteomics and Bioinformatics, 18(1), 41–51. https://doi.org/10.1016/j.gpb.2019.11.005
_ = combSpec.mass_heatmap(pw.protein2mass.get("Tmsb4x"), scaled=True)
combSpec.mass_intensity(pw.protein2mass.get("Tmsb4x"), regions=["slided_0", "slided_1"], scaled=True)
for gene in np.unique(mydf["gene"]):
print(gene)
_ = combSpec.mass_heatmap(pw.protein2mass.get(gene), scaled=True)
combSpec.mass_intensity(pw.protein2mass.get("Ptges3"), regions=["slided_0", "slided_1"], scaled=True)
Another DE Analysis: whole Aorta¶
Having spotted several differences between the Tunica media of atherosclerotic and suspected healthy aorta, a full comparison might also be of interest.
For this, all slided_0 and slided_1 regions are compared - with the exception of the backgrounds.
slided_0_regions = tuple(sorted([x for x in np.unique(slided_0.segmented) if x > 0]))
slided_1_regions = tuple(sorted([x for x in np.unique(slided_1.segmented) if x > 0]))
combSpec.regions["slided_0"].plot_segments(highlight=slided_0_regions)
combSpec.regions["slided_1"].plot_segments(highlight=slided_1_regions)
resdf_all, expr, pdata = combSpec.find_markers("slided_0", slided_0_regions, "slided_1", slided_1_regions, pw, scaled=True)
combSpec.list_de_results()
mydf_all = resdf_all["ttest"][('slided_0', slided_0_regions, 'slided_1', slided_1_regions)]
plt.figure(figsize=(16,10))
xydots = [(x,y) for x,y in zip(list(-mydf_all["avg_logFC"]), list(-np.log10(mydf_all["qvalue"])))]
dotgene = list(mydf_all["gene"])
texts = []
seenProts = set()
for i in range(len(xydots)):
x = xydots[i][0]
y = xydots[i][1]
if not dotgene[i] in seenProts and abs(y) >= 10 and abs(x) >= 0.5:
texts.append(plt.text(x * (1 + 0.01), y * (1 + 0.01) , dotgene[i], fontsize=12))
plt.plot(x, y, 'ro')
seenProts.add(dotgene[i])
else:
plt.plot(x, y, 'bo')
adjust_text(texts, force_points=0.2, force_text=0.2, expand_points=(1, 1), expand_text=(1, 1), arrowprops=dict(arrowstyle="-", color='black', lw=0.5))
plt.show()
The comparison of both aorta results again in several differential proteins.
Again, several proteins involved in the respiratory metabolism are listed, like Ndufc2, Ndufa11.
Of interest here are Ifitm3, Ccdc126 and Ubl5, which are all located in the inner part of the aorta.
Ifitm3¶
Known gene involved in the innate immune system (T cells): Yánez, D. C., Ross, S., & Crompton, T. (2020). The IFITM protein family in adaptive immunity. In Immunology (Vol. 159, Issue 4, pp. 365–372). Blackwell Publishing Ltd. https://doi.org/10.1111/imm.13163
Ifitm3 is involved in the plaque uptake in Alzheimer’s disease: Hur, J. Y., Frost, G. R., Wu, X., Crump, C., Pan, S. J., Wong, E., Barros, M., Li, T., Nie, P., Zhai, Y., Wang, J. C., Tcw, J., Guo, L., McKenzie, A., Ming, C., Zhou, X., Wang, M., Sagi, Y., Renton, A. E., … Li, Y. M. (2020). The innate immunity protein IFITM3 modulates γ-secretase in Alzheimer’s disease. Nature, 1–6. https://doi.org/10.1038/s41586-020-2681-2
Mrps14¶
Mrps14 effects the mitochondrial translation. Hence, again, a disturbation of mitochondrial work can be observed.
Jackson, C. B., Huemer, M., Bolognini, R., Martin, F., Szinnai, G., Donner, B. C., Richter, U., Battersby, B. J., Nuoffer, J. M., Suomalainen, A., & Schaller, A. (2019). A variant in MRPS14 (uS14m) causes perinatal hypertrophic cardiomyopathy with neonatal lactic acidosis, growth retardation, dysmorphic features and neurological involvement. Human Molecular Genetics, 28(4), 639–649. https://doi.org/10.1093/hmg/ddy374
Acot13¶
In chicken it was observed that an Acot13 decreases works as inhibitor during Preadipocytes Differentiation. Hence, an upregulation of Acot13 leads to an increase in preadipocyte differentiation, which also plays a role in plaque formation.
_ = combSpec.mass_heatmap(pw.protein2mass.get("Ifitm3"), scaled=True)
_ = combSpec.mass_heatmap(pw.protein2mass.get("Mrps14"), scaled=True)
_ = combSpec.mass_heatmap(pw.protein2mass.get("Acot13"), scaled=True)
for x in set(mydf_all["gene"]):
print(x)
_ = combSpec.mass_heatmap(pw.protein2mass.get(x), scaled=True)
combSpec.mass_intensity(pw.protein2mass.get("Ccdc126"), regions=["slided_0", "slided_1"], scaled=True)
combSpec.regions["slided_0"].plot_segments(highlight=1)
_ = combSpec.mass_heatmap(pw.protein2mass.get("Ccdc126"), scaled=True)
combSpec.mass_intensity(pw.protein2mass.get("Hsbp1"), regions=["slided_0", "slided_1"], scaled=True)
_ = combSpec.mass_heatmap(pw.protein2mass.get("Hsbp1"), scaled=True)
The core DE¶
slided_0_inner_regions = tuple([1,3,4,6])
slided_1_inner_regions = tuple([2,3,7,8,9])
combSpec.regions["slided_0"].plot_segments(highlight=slided_0_inner_regions)
combSpec.regions["slided_1"].plot_segments(highlight=slided_1_inner_regions)
resdf_inner, expr, pdata = combSpec.find_markers("slided_0", slided_0_inner_regions, "slided_1", slided_1_inner_regions, pw, scaled=True)
mydf_inner = resdf_inner["ttest"][('slided_0', slided_0_inner_regions, 'slided_1', slided_1_inner_regions)]
plt.figure(figsize=(16,10))
xydots = [(x,y) for x,y in zip(list(-mydf_inner["avg_logFC"]), list(-np.log10(mydf_inner["qvalue"])))]
dotgene = list(mydf_inner["gene"])
texts = []
seenProts = set()
for i in range(len(xydots)):
x = xydots[i][0]
y = xydots[i][1]
if not dotgene[i] in seenProts and abs(y) >= 10 and abs(x) >= 0.5:
texts.append(plt.text(x * (1 + 0.01), y * (1 + 0.01) , dotgene[i], fontsize=12))
plt.plot(x, y, 'ro')
seenProts.add(dotgene[i])
else:
plt.plot(x, y, 'bo')
adjust_text(texts, force_points=0.2, force_text=0.2, expand_points=(1, 1), expand_text=(1, 1), arrowprops=dict(arrowstyle="-", color='black', lw=0.5))
plt.show()
Again the same candidates as previously shown can be seen.
Mrps14, Ccdc126, Ndufa11 - which mostly relate to mitochondrial activity.
Timm8b¶
Likewise is Timm8b significantly upregulated.
Timm8b is found to be upregulated in colon mucosa cardinogenesis of diabetes type 2 patients, and is associated with mitochondrial dysfunction. A similar pattern might be observable in atherosclerosis as well.
Del Puerto-Nevado, L., Santiago-Hernandez, A., Solanes-Casado, S., Gonzalez, N., Ricote, M., Corton, M., Prieto, I., Mas, S., Sanz, A. B., Aguilera, O., Gomez-Guerrero, C., Ayuso, C., Ortiz, A., Rojo, F., Egido, J., Garcia-Foncillas, J., Minguez, P., & Alvarez-Llamas, G. (2019). Diabetes-mediated promotion of colon mucosa carcinogenesis is associated with mitochondrial dysfunction. Molecular Oncology, 13(9), 1887–1897. https://doi.org/10.1002/1878-0261.12531
GO analysis for plaque-DE experiment¶
! wget http://ftp.ebi.ac.uk//pub/databases/GO/goa/HUMAN/goa_human.gaf.gz
! wget 'http://purl.obolibrary.org/obo/go/go-basic.obo'
import gzip
import Bio.UniProt.GOA as GOA
from goatools.go_enrichment import GOEnrichmentStudy
from goatools import obo_parser
goa_human = "goa_human.gaf.gz"
# File is a gunzip file, so we need to open it in this way
with gzip.open(goa_human, 'rt') as arab_gaf_fp:
human_funcs = {} # Initialise the dictionary of functions
# Iterate on each function using Bio.UniProt.GOA library.
for entry in GOA.gafiterator(arab_gaf_fp):
uniprot_id = entry.pop('DB_Object_Symbol')
human_funcs[uniprot_id] = entry
go = obo_parser.GODag("go-basic.obo")
for i,x in enumerate(human_funcs):
if x.startswith("CCL"):
print(i,x, human_funcs[x])
break
keyword = 'growth'
growth_dict = {x: human_funcs[x]
for x in human_funcs
if keyword in human_funcs[x]['DB_Object_Name']}
print('UniProt IDs of annotations with "growth" in their name:')
print("Total: {}".format(len(growth_dict)))
pop = [x.upper() for x in pw.protein2mass]
assoc = {}
for x in human_funcs:
if x not in assoc:
assoc[x] = set()
assoc[x].add(str(human_funcs[x]['GO_ID']))
methods = ["bonferroni", "fdr"]
study = list(set([x.upper() for x in mydf["gene"]])) #mydf_all
g = GOEnrichmentStudy(pop, assoc, go,
propagate_counts=True,
alpha=0.05,
methods=['bonferroni', 'fdr_bh'])
g_res = g.run_study(study)
for x in sorted(g_res, key=lambda x: (x.study_count, -x.p_uncorrected), reverse=True):
if x.study_count > 1:
print(x.study_count, x)